The setOnAction Method in JavaFX
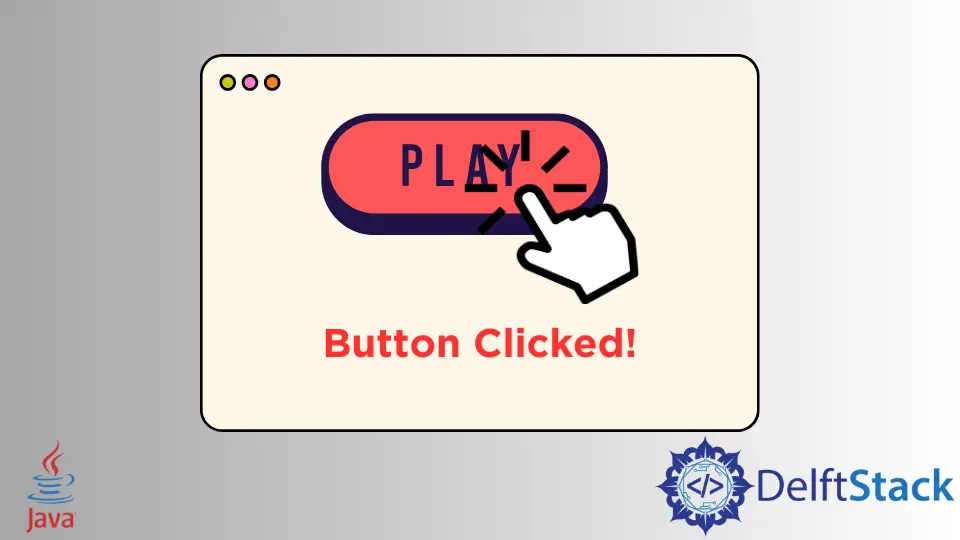
An essential aspect of building interactive user interfaces in JavaFX is implementing actions. An action represents a system process that triggers when a user commands the system to perform a specific task.
Without actions, the user interface remains static and non-responsive. In JavaFX, the setOnAction()
method plays a crucial role in defining these actions.
In this article, we will show how we can create an action for any UI component. Also, we will show an example with an explanation to make this topic easier to understand.
Use the setOnAction()
Method in JavaFX
In JavaFX, the setOnAction()
method is a fundamental tool for adding action functionality to UI components, such as buttons, menus, or other interactive elements that users can interact with. This method allows developers to specify what should happen when a particular event, often a user interaction like a button click, occurs.
The syntax for using setOnAction()
is straightforward:
button.setOnAction(event
-> {
// Action code goes here
});
Here, we set the action for the button using a lambda expression, which defines the behavior that occurs when the button is clicked.
To use the setOnAction()
method, follow these steps:
-
First, create the UI control (e.g., a button) that you want to associate with the action.
Button button = new Button("Click me");
-
Define the action you want to execute when the event is triggered. This is typically done using a lambda expression.
button.setOnAction(event -> { // Define the action to be performed after the button is clicked System.out.println("Button clicked!"); });
-
Use the
setOnAction()
method to associate the defined action with the UI control.button.setOnAction(/* action defined here */);
-
Within the action block, you can include any logic or operations you want to perform when the event is triggered.
To illustrate the use of setOnAction()
further, let’s explore a simple example where we create a UI with a button and a label. When the button is clicked, the label’s text is updated to indicate the button was clicked.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class FXsetAction extends Application {
Button Btn;
Label lbl;
Scene scene;
HBox _hbox;
public void start(Stage PrimaryStage) throws Exception {
PrimaryStage.setTitle("JavaFX setOnAction");
lbl = new Label("Button not clicked");
Btn = new Button("Click");
Btn.setOnAction(value -> { lbl.setText("Button Clicked!!!"); });
_hbox = new HBox(Btn, lbl);
scene = new Scene(_hbox, 400, 300);
PrimaryStage.setScene(scene);
PrimaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
In this example code, we created a simple graphical user interface (GUI). First, the code imports the necessary JavaFX packages for building the interface.
The main class, FXsetAction
, extends Application
as it’s a JavaFX application. It defines class-level variables: a Button
(Btn
), a Label
(lbl
), a Scene
(scene
), and an HBox
layout (_hbox
).
Then, the start
method, an override from Application
, sets the application’s title and creates a label with the default text "Button not clicked"
and a button labeled "Click"
. It sets up an event handler for the button so that when it’s clicked, the label text changes to "Button Clicked!!!"
.
The button and label are placed within an HBox
. Finally, the scene is set with the HBox as its root, the stage is set with the scene, and the application is displayed when executed using the main
method.
Now, when you run the code after a successful compilation, a window will open with the title JavaFX setOnAction
, and after you press a key from your keyboard, you will see an output like the one below.
Output:
Extending Functionality With setOnAction()
Beyond updating a label’s text, the setOnAction()
method can be used to implement a wide range of functionalities, depending on the application’s requirements. Actions can involve anything from updating UI elements to invoking complex operations, fetching data from a server, or transitioning to another scene within the application.
Here, we’ll discuss in more detail the versatility and potential applications of extending functionality with setOnAction()
.
Update UI Elements
One of the most common uses of setOnAction()
is updating UI elements to reflect changes based on user interactions. For instance, clicking a button could change the text of a label, toggle the visibility of a node, or modify the properties of a UI component like color, font, or size.
button.setOnAction(event -> {
label.setText("Button Clicked!");
// Update other UI elements or properties
});
Perform Data Manipulation
Actions triggered by setOnAction()
can involve data manipulation, such as updating a database, saving a file, or processing input data. For example, clicking a Save
button could save the contents of a form to a database.
saveButton.setOnAction(event -> {
// Save data to the database
dataManager.saveFormData(formData);
});
Navigation and Scene Transitions
The setOnAction()
method can also be used to facilitate navigation within an application. This includes transitioning between different scenes, opening new windows, or changing the displayed content based on user actions.
For example, clicking a Next
button could transition to the next screen in a multi-step process.
nextButton.setOnAction(event -> {
// Change to the next scene
stage.setScene(nextScene);
});
Perform Validation
Actions triggered by setOnAction()
can also involve validation of user input. For instance, clicking a Submit
button could trigger validation checks to ensure that the entered data meets certain criteria.
submitButton.setOnAction(event -> {
if (inputIsValid()) {
// Perform action on valid input
} else {
// Display an error message or handle invalid input
}
});
Event Handling and Error Handling
Besides modifying UI elements or data, setOnAction()
can be used to handle events and errors gracefully. For example, clicking a button could trigger actions that handle potential errors or exceptions that may occur during the application’s execution.
actionButton.setOnAction(event -> {
try {
// Perform action that may throw an exception
performPotentiallyRiskyAction();
} catch (Exception e) {
// Handle the exception and display an error message
displayErrorMessage("An error occurred: " + e.getMessage());
}
});
Integration with External Services
Actions triggered by setOnAction()
can involve integration with external services or APIs. For instance, clicking a Send
button could initiate communication with a server to send a message.
sendButton.setOnAction(event -> {
// Send a message via a messaging API
messagingService.sendMessage(message);
});
Conclusion
The setOnAction()
method empowers developers to create interactive, feature-rich applications that cater to diverse user requirements with its ability to execute a wide range of actions. By effectively leveraging this method, developers can enhance the application’s functionality, user experience, and overall usefulness.
Remember, if your IDE doesn’t support the automatic inclusion of libraries and packages, you may need to manually include these necessary libraries and packages before compiling.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn