How to Return a String in Java
-
Use the
return
Statement to Return a String in Java -
Use
StringBuilder
to Return a String in Java -
Use
String.format()
to Return a String in Java - Conclusion
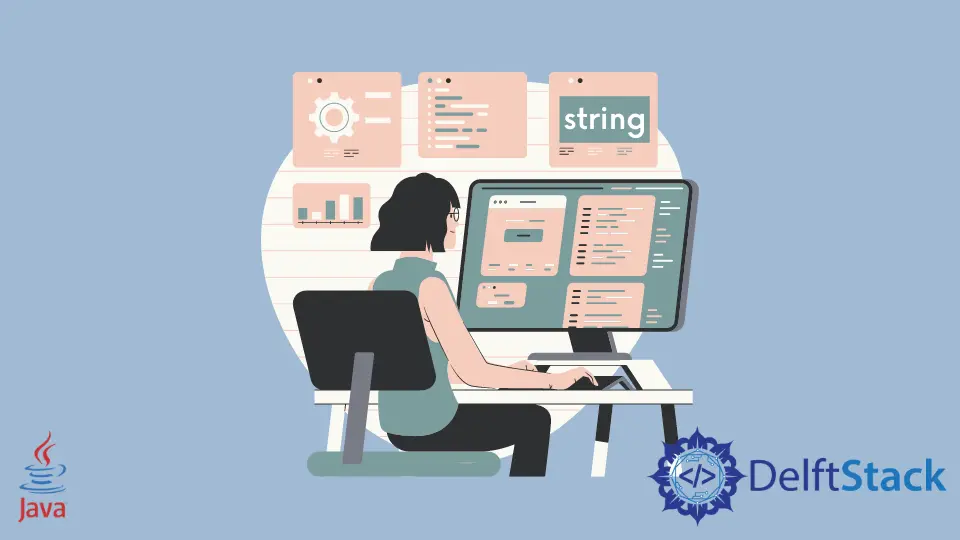
In Java programming, manipulating strings is a fundamental skill that both beginners and seasoned developers must master. One crucial aspect of string manipulation is understanding how to effectively return strings from methods.
Whether you’re just starting your Java journey or looking to refine your skills as a seasoned professional, this article aims to serve as your go-to guide for mastering the art of returning strings in Java. This article delves into various techniques to accomplish this.
Use the return
Statement to Return a String in Java
In Java, the return
statement is the most straightforward approach.
Just like a chef finishing a dish and presenting it to the customer, the return
statement lets a method serve up its output to the rest of the program. It’s the final touch that completes the method’s task, ensuring that the calculated value, piece of information, or result finds its way back to where it’s needed.
In essence, return
marks the endpoint of a method’s journey, delivering what it promises to contribute to the overall functionality of the program. In the context of strings, use return
to return a predefined string or concatenate strings.
Syntax:
return "Hello there!";
To understand this clearly, look at the sample code below:
public class Main {
public static String returnString() {
return "Hello, World!";
}
public static void main(String[] args) {
String result = returnString();
System.out.println(result);
}
}
Output:
Hello, World!
In this Java program, the returnString()
method is designed to fulfill a simple purpose — it returns a string.
Specifically, in this example, the string the method returns is "Hello, World!"
. This method serves as an example of how a function in Java can produce a string output.
The main
method, as the name implies, is the main entry point of the program. Here, a variable named result
is declared to store the value returned by the returnString()
method.
This method call essentially retrieves the string "Hello, World!"
and assigns it to the result
variable.
Finally, the program prints the value stored in the result
variable using System.out.println()
. As a result, when this Java program is executed, the console will display the text "Hello, World!"
.
This code serves as a concise illustration of the foundational concept of returning strings in Java.
Use StringBuilder
to Return a String in Java
In Java, the StringBuilder
class is a powerful tool when it comes to manipulating and constructing strings dynamically. Unlike regular strings that are immutable (unchangeable), StringBuilder
provides a mutable alternative, allowing for efficient modifications and concatenations.
One of the key applications of StringBuilder
is in the construction of strings that are returned from methods. By leveraging the StringBuilder
methods, such as append()
, you can efficiently build the desired string within the method and then convert it to a regular string using toString()
.
This approach ensures flexibility and performance when generating strings in Java.
Syntax:
StringBuilder stringBuilder = new StringBuilder();
The line of code above simply creates a StringBuilder
with the variable name stringBuilder
. For now, it’s like an empty container waiting to hold some text.
We would implement this in the following sample code below.
public class Main {
public static String returnString() {
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("Hello, ");
stringBuilder.append("World!");
return stringBuilder.toString();
}
public static void main(String[] args) {
String result = returnString();
System.out.println(result);
}
}
Output:
Hello, World!
In the provided Java code, the returnString()
method utilizes a StringBuilder
to concatenate two distinct strings. The first string, "Hello, "
, is appended to the StringBuilder
, followed by the second string, "World!"
.
Subsequently, the complete string is obtained by invoking the toString()
method on the StringBuilder
, and this resulting string is then returned by the returnString()
method.
The program’s entry point is the "main"
method, where execution begins. Within this method, the returnString()
method is invoked, and the returned string is stored in a variable named "result"
.
Finally, the content of the "result"
variable is printed to the console using the System.out.println()
statement.
In essence, when the program is executed, it will display the concatenated "Hello, World!"
string as the output. This example illustrates a straightforward yet effective use of StringBuilder for string manipulation in Java, showcasing a common practice employed by developers to efficiently build and return strings.
Use String.format()
to Return a String in Java
In Java, the String.format()
method serves as a versatile tool for creating well-formatted strings. It allows developers to construct strings with dynamic content by replacing placeholders with actual values.
Think of it as a template where you define the structure of your string, leaving blanks to be filled later.
This method is particularly useful when you need precise control over the appearance of your output or when constructing complex strings with variable components. In this section, we’ll explore how String.format()
is employed to return strings in a clean and organized manner.
Syntax:
String.format("Hello, %s", "World!");
In this example, %s
is a placeholder for a string. The String.format()
method replaces %s
with the provided argument "World,"
resulting in the formatted string "Hello, World!"
.
Take a look at the full working code:
public class Main {
public static String returnString() {
return String.format("Hello, %s", "World!");
}
public static void main(String[] args) {
String result = returnString();
System.out.println(result);
}
}
Output:
Hello, World!
In this Java program, the method returnString()
utilizes the String.format()
function to create a dynamic string by incorporating the placeholder %s
. This placeholder is subsequently replaced with the literal string "World!"
during the execution of the program.
Moving on to the main
function, it serves as the entry point of the program. Here, the returnString()
method is invoked, and its result is stored in a variable named result
.
Finally, the System.out.println()
statement is employed to display the content of the result
variable on the console. In essence, when the program is executed, it will output the string "Hello, World!"
.
This concise Java code exemplifies a fundamental principle of string manipulation, demonstrating how to create dynamic strings by incorporating variables within a predefined template. It is a simple yet illustrative example that showcases the use of the String.format()
method to enhance the flexibility and readability of string construction in Java.
Conclusion
Each method demonstrated in this article has its unique advantages. The return
statement is simple and effective for fixed strings, StringBuilder
is powerful for dynamic string manipulation, and String.format()
provides a clean way to format strings.
Depending on your use case, choose the method that best suits your needs. Feel free to experiment with these methods in your own projects to get a hands-on understanding of their applicability and efficiency.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn