How to Replace a Backslash With a Double Backslash in Java
- Understanding Backslashes in Java
- Method 1: Using String.replace() Method
- Method 2: Using String.replaceAll() Method
- Method 3: Using StringBuilder for Performance
- Conclusion
- FAQ
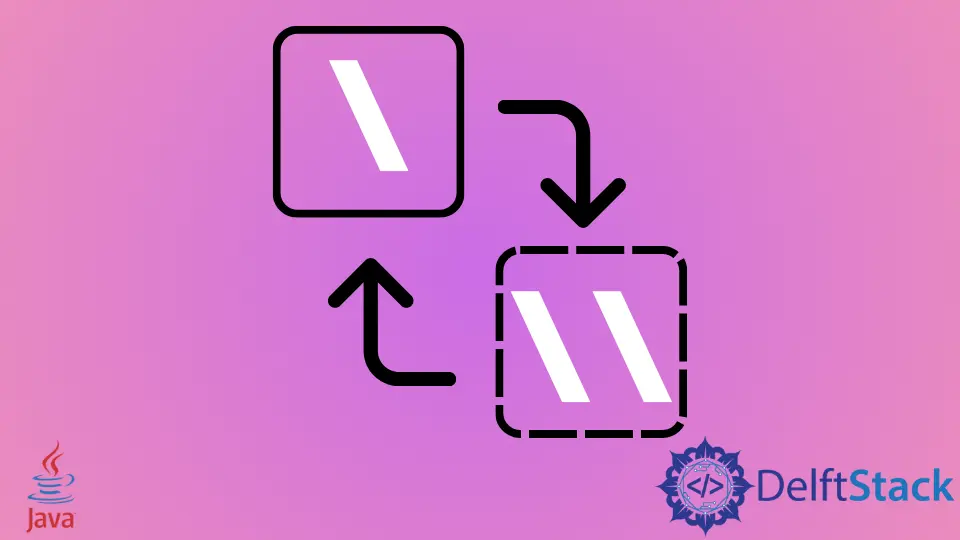
When working with strings in Java, you may occasionally need to manipulate text by replacing characters. One common scenario is replacing a single backslash with a double backslash. This need often arises when dealing with file paths or regular expressions.
In this article, we will explore various methods to achieve this in Java, providing clear examples and explanations. Whether you are a beginner or have some experience in Java programming, you will find this guide helpful and informative. Let’s dive in and learn how to replace a backslash with a double backslash in Java!
Understanding Backslashes in Java
Before we dive into the methods of replacing backslashes, it’s essential to understand why backslashes can be tricky in Java. The backslash (\
) is an escape character in Java, which means it is used to represent special characters. For instance, \n
represents a newline, and \t
represents a tab. Because of this, a single backslash needs to be escaped itself when you want to use it as a literal character. Hence, to represent a single backslash in a string, you actually need to use two backslashes (\\
). This can lead to confusion, especially for those new to Java.
Method 1: Using String.replace() Method
One of the simplest ways to replace a backslash with a double backslash in Java is by using the String.replace()
method. This method takes two parameters: the character or substring you want to replace and the new character or substring you want to use. Here’s how it works:
public class BackslashReplacement {
public static void main(String[] args) {
String originalString = "C:\\Users\\Example";
String modifiedString = originalString.replace("\\", "\\\\");
System.out.println(modifiedString);
}
}
Output:
C:\\Users\\Example
In this code, we start with a string that contains single backslashes. The replace()
method is called on the originalString
, where we specify that we want to replace each single backslash with a double backslash. The result is stored in modifiedString
, which we then print out. This method is straightforward and effective for simple replacements.
Method 2: Using String.replaceAll() Method
Another method to replace a backslash with a double backslash in Java is by using the String.replaceAll()
method. This method is particularly useful when dealing with regular expressions. Here’s how you can implement it:
public class BackslashReplacement {
public static void main(String[] args) {
String originalString = "C:\\Users\\Example";
String modifiedString = originalString.replaceAll("\\\\", "\\\\\\\\");
System.out.println(modifiedString);
}
}
Output:
C:\\Users\\Example
In this example, the replaceAll()
method is used instead of replace()
. The key difference is that replaceAll()
interprets the first argument as a regular expression. Therefore, we need to escape the backslash twice in both the target and replacement strings. The result is the same as before, but this method can be more powerful when working with patterns.
Method 3: Using StringBuilder for Performance
If you are dealing with larger strings or require multiple replacements, using StringBuilder
can be more efficient. This approach allows you to build your string dynamically. Here’s how you can use it:
public class BackslashReplacement {
public static void main(String[] args) {
String originalString = "C:\\Users\\Example";
StringBuilder modifiedString = new StringBuilder();
for (char ch : originalString.toCharArray()) {
if (ch == '\\') {
modifiedString.append("\\\\");
} else {
modifiedString.append(ch);
}
}
System.out.println(modifiedString.toString());
}
}
Output:
C:\\Users\\Example
In this code, we create a StringBuilder
instance to build our new string. We iterate through each character in the originalString
. If we encounter a backslash, we append two backslashes to the StringBuilder
. If the character is anything else, we append it as is. Finally, we convert the StringBuilder
back to a string and print the result. This method is particularly beneficial when you have to handle a large number of replacements, as it minimizes the overhead associated with string concatenation.
Conclusion
Replacing a backslash with a double backslash in Java is a straightforward task, thanks to the flexibility of string manipulation methods available in the language. Whether you choose to use String.replace()
, String.replaceAll()
, or StringBuilder
, each method has its advantages depending on your specific needs. By understanding how to handle backslashes effectively, you can avoid common pitfalls and ensure your Java applications run smoothly. Happy coding!
FAQ
-
What is the purpose of using double backslashes in Java?
Double backslashes are used to represent a single backslash in string literals due to backslash being an escape character. -
Can I use single backslashes in regular expressions in Java?
Yes, but you need to escape them, hence using double backslashes in the regular expression. -
Is there a performance difference between using String.replace() and StringBuilder?
Yes, StringBuilder is generally more efficient for multiple replacements or when working with large strings. -
Can I replace other characters in the same way?
Absolutely! The same methods can be applied to replace any character or substring in Java strings. -
How do I know which method to use for string replacement?
It depends on your specific needs; for simple replacements, usereplace()
, for regex patterns, usereplaceAll()
, and for performance with large strings, useStringBuilder
.