How to Print List in Java
-
Print List in Java Using the Enhanced
for
Loop -
Print List in Java Using the
toString()
Method -
Print List in Java Using the
forEach()
Method -
Print List in Java Using the
Iterator
Interface - Conclusion
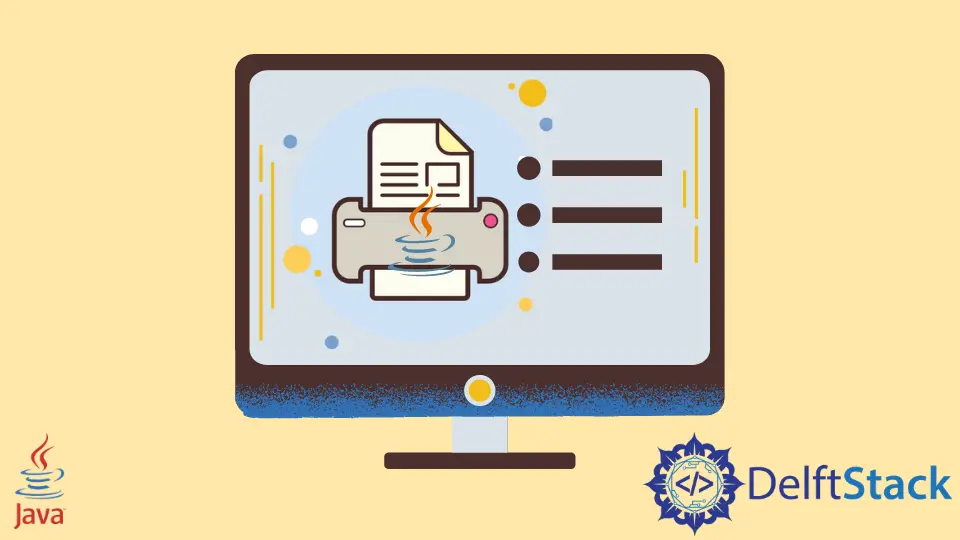
Iterating through a list is a fundamental operation in Java, and various approaches are available to accomplish this task. In this comprehensive guide, we’ll explore different methods to print every single item of a list in Java, discussing the syntax, usage, and suitability of each method.
Print List in Java Using the Enhanced for
Loop
One of the simplest and most readable methods to iterate through list elements is using the enhanced for
loop.
The enhanced for
loop, also known as the for-each
loop, simplifies the process of iterating through collections, including lists. Its syntax is as follows:
for (ElementType element : collection) {
// code to be executed for each element
}
Here, ElementType
is the type of elements in the collection, and collection
is the list or array to be iterated. The loop automatically iterates over each element in the collection, and the specified code block is executed for each iteration.
Let’s consider a scenario where we have a list of strings and want to print each string using the enhanced for
loop.
import java.util.Arrays;
import java.util.List;
public class EnhancedForLoopExample {
public static void main(String[] args) {
List<String> stringList = Arrays.asList("Apple", "Banana", "Orange");
for (String fruit : stringList) {
System.out.println(fruit);
}
}
}
Output:
Apple
Banana
Orange
In this example, we first create a list of strings using Arrays.asList()
. The list, named stringList
, contains three fruit names: Apple
, Banana
, and Orange
.
The enhanced for
loop is then employed to iterate through each element in the stringList
. The loop header for (String fruit : stringList)
declares a variable fruit
of type String
, which takes on the value of each element in the list during each iteration.
Inside the loop, System.out.println(fruit)
prints each fruit to the console. The enhanced for
loop handles the iteration, making the code concise and easy to read.
This method is particularly useful when you only need to traverse the elements of a collection sequentially without requiring the index or manipulating the iteration variable. The enhanced for
loop enhances the readability of the code, reducing the chances of errors and making it more maintainable.
Print List in Java Using the toString()
Method
While the enhanced for
loop provides a clean and concise way to iterate through a list in Java, another approach involves leveraging the toString()
method. This method is particularly useful when the list contains objects with a meaningful toString()
implementation.
The toString()
method is a part of the Object
class in Java. Its general syntax is as follows:
public String toString() {
// code to convert the object to a string representation
}
To use this method for printing elements in a list, the objects in the list should have a customized toString()
method that returns a meaningful string representation.
Consider a scenario where we have a custom class Book
with a proper toString()
method. We’ll create a list of Book
objects and print each object using the toString()
method.
import java.util.ArrayList;
import java.util.List;
class Book {
private String title;
private String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
@Override
public String toString() {
return "Book: " + title + " by " + author;
}
}
public class ToStringMethodExample {
public static void main(String[] args) {
List<Book> bookList = new ArrayList<>();
bookList.add(new Book("The Great Gatsby", "F. Scott Fitzgerald"));
bookList.add(new Book("To Kill a Mockingbird", "Harper Lee"));
bookList.add(new Book("1984", "George Orwell"));
for (Book book : bookList) {
System.out.println(book.toString());
}
}
}
Output:
Book: The Great Gatsby by F. Scott Fitzgerald
Book: To Kill a Mockingbird by Harper Lee
Book: 1984 by George Orwell
In this example, we define a Book
class with a constructor that takes the title and author as parameters. The class also overrides the toString()
method to provide a custom string representation, combining the title and author.
In the main
method, we create a list of Book
objects, and each object is added to the bookList
. The enhanced for
loop is then used to iterate through the list.
For each Book
object, the toString()
method is implicitly called within the System.out.println()
statement. This results in printing a meaningful string representation of each Book
object.
Using the toString()
method in this manner is advantageous when you want a customized and meaningful representation of objects in your list. It enhances code readability and makes the output more informative, especially in scenarios where the default toString()
implementation provided by the Object
class may not be sufficient.
Print List in Java Using the forEach()
Method
In Java, the introduction of functional programming features in Java 8 brought along the versatile forEach()
method, providing an elegant way to iterate through collections like lists. This method simplifies the process of applying an action to each element of the list.
The forEach()
method is part of the Iterable
interface and was introduced in Java 8. Its syntax is as follows:
void forEach(Consumer<? super T> action);
Here, action
is a functional interface representing the operation to be performed on each element. The method applies this operation to each element in the collection.
Let’s explore an example using a list of integers. We’ll use the forEach()
method to print each element in the list.
import java.util.Arrays;
import java.util.List;
public class ForEachMethodExample {
public static void main(String[] args) {
List<Integer> numberList = Arrays.asList(1, 2, 3, 4, 5);
numberList.forEach(item -> System.out.println(item));
}
}
Output:
1
2
3
4
5
In this example, we create a list of integers using Arrays.asList()
, named numberList
. The forEach()
method is then applied to this list, taking a lambda expression as its argument.
The lambda expression (item -> System.out.println(item))
serves as the action to be performed on each element. It specifies that for each item
in the list, the code block inside the lambda (in this case, System.out.println(item)
) will be executed.
The forEach()
method internally handles the iteration through the list, making the code concise and expressive. It encapsulates the iteration logic, enhancing code readability.
The lambda expression brings a functional programming aspect to the loop, making it more flexible for various operations.
Utilizing the forEach()
method is particularly beneficial when the logic to be applied to each element is concise and can be expressed as a lambda expression. It contributes to writing cleaner, more expressive code with less boilerplate.
Print List in Java Using the Iterator
Interface
Java provides the Iterator
interface as a means to traverse collections, offering more control and flexibility over the iteration process. This interface is part of the java.util
package.
Its basic syntax involves creating an iterator object and using it to traverse through the elements of a collection:
Iterator<T> iterator = collection.iterator();
while (iterator.hasNext()) {
T element = iterator.next();
// code to be executed for each element
}
Here, T
is the type of elements in the collection, and collection
is the list or collection to be iterated. The hasNext()
method checks if there are more elements, and next()
retrieves the next element in the iteration.
Let’s consider an example where we have a list of Double
values, and we want to print each value using an Iterator
.
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class IteratorExample {
public static void main(String[] args) {
List<Double> doubleList = new ArrayList<>();
doubleList.add(3.14);
doubleList.add(2.71);
doubleList.add(1.618);
Iterator<Double> iterator = doubleList.iterator();
while (iterator.hasNext()) {
Double value = iterator.next();
System.out.println(value);
}
}
}
Output:
3.14
2.71
1.618
In this example, we create a list of Double
values named doubleList
. We then obtain an iterator for this list using the iterator()
method, creating an Iterator<Double>
object named iterator
.
The while
loop checks if there are more elements in the list using iterator.hasNext()
. If there are, the loop enters, and iterator.next()
is called to retrieve the next element.
This element, in this case, a Double
value, is stored in the variable value
.
The System.out.println(value)
statement then prints each Double
value to the console. The iterator moves to the next element in the list with each iteration.
Using an Iterator
provides fine-grained control over the iteration process, allowing you to perform more complex operations on each element. It is especially useful when you need to manipulate the iterator itself or when dealing with collections that do not support the enhanced for
loop or forEach()
method.
Conclusion
Mastering list iteration in Java involves choosing the right method based on the specific requirements of your code.
Whether it’s the simplicity of the enhanced for
loop, the meaningful representation provided by toString()
, the expressive power of forEach()
, or the fine-grained control offered by Iterator
, Java provides a variety of tools to make list iteration efficient and readable.
Choose the method that best fits your use case and coding style.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn