How to Print a String in Java
-
Using the
print()
Method to Print a String in Java -
Using
Scanner
Input andprintln
Method to Print a String in Java -
Using the
printf()
Method to Print a String in Java
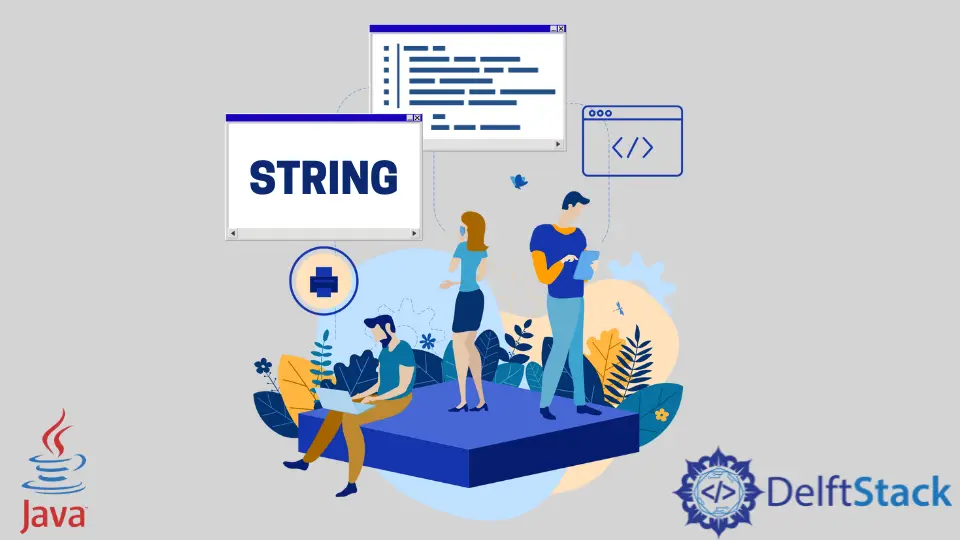
In Java, the string is an object that represents a sequence of characters. Here we will look at various methods to print a string in Java.
Using the print()
Method to Print a String in Java
In the code snippet given below, we have a string-type variable, str
. To print the value of this variable for the user on the console screen, we will use the print()
method.
We pass the text to be printed as a parameter to this method as a String
. The cursor stays at the end of the text at the console, and the next print starts from the same line as we can see in the output.
public class StringPrint {
public static void main(String[] args) {
String str = "This is a string stored in a variable.";
System.out.print(str);
System.out.print("Second print statement.");
}
}
Output:
This is a string stored in a variable.Second print statement.
Using Scanner
Input and println
Method to Print a String in Java
Here, we use the Scanner
class to get input from the user.
We create an object of the Scanner
class, and we ask the user to enter his name using the print()
method. We call the nextLine()
method on the input
object to get the user’s input string.
The user input is stored in a String
type variable. Later, we used the println()
method to print the concatenated string and the +
operator to concatenate two strings.
Then, the print()
and println()
methods are used to print the string inside the quotes. But in the println()
method, the cursor moves to the beginning of the next line.
At last, we close the scanner input using the close()
method.
import java.util.Scanner;
public class StringPrint {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = input.nextLine();
System.out.println("Your name is " + name);
input.close();
}
}
Output:
Enter your name: Joy
Your name is Joy
Using the printf()
Method to Print a String in Java
The printf()
method provides string formatting. We can provide various format specifiers; based on these specifiers, it formats the string and prints it on the console.
Here we have used two format specifiers, %s
and %d
, where %s
is used for string while %d
is used for signed decimal integer. We have also used \n
, which inserts a new line at a specific point in the text.
public class StringPrint {
public static void main(String[] args) {
String str = "The color of this flower is ";
int i = 20;
System.out.printf("Printing my string : %s\n", str);
System.out.printf("Printing int : %d\n", i);
}
}
Output:
Printing my string : The color of this flower is
Printing int : 20
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn