Not Equals in Java
-
The Not Equals Operator:
!=
-
Comparing Objects with
!=
-
Using the
.equals()
Method for Content Comparison - Summary of Not Equals in Java
- Conclusion
- FAQ
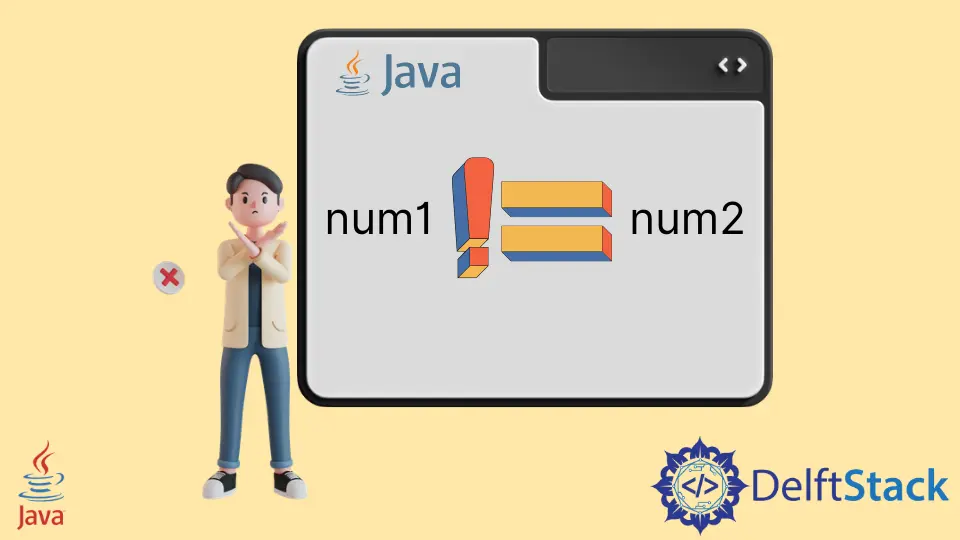
In the world of programming, understanding how to compare values is crucial, and in Java, the concept of “not equals” is fundamental.
This tutorial shines some light on the topic of not equals in Java, exploring how to effectively use the !=
operator, and how it differs from the .equals()
method. Whether you’re a beginner or looking to brush up on your Java skills, this guide will help clarify these concepts and provide practical examples. By the end, you’ll have a solid grasp of how to implement not equals in your Java projects, ensuring your code is both efficient and effective.
The Not Equals Operator: !=
In Java, the !=
operator is used to compare two values to determine if they are not equal. This operator can be applied to both primitive data types and object references. When using !=
, Java checks the values of the variables on either side of the operator. If the values are different, the expression evaluates to true
; otherwise, it evaluates to false
.
Here’s a simple example demonstrating the !=
operator:
public class NotEqualsExample {
public static void main(String[] args) {
int a = 5;
int b = 10;
if (a != b) {
System.out.println("a is not equal to b");
} else {
System.out.println("a is equal to b");
}
}
}
Output:
a is not equal to b
In this example, we declare two integer variables, a
and b
. The if
statement checks if a
is not equal to b
. Since 5 is not equal to 10, the program prints “a is not equal to b”. This simple demonstration highlights how the !=
operator functions for primitive data types.
Comparing Objects with !=
When it comes to object comparison, the !=
operator checks if two references point to different objects in memory. This is crucial in Java, as comparing objects involves more complexity than comparing primitive types. If two object references point to the same object, !=
will return false
, even if the contents of the objects are different.
Consider the following example:
public class ObjectNotEqualsExample {
public static void main(String[] args) {
String str1 = new String("Hello");
String str2 = new String("Hello");
if (str1 != str2) {
System.out.println("str1 and str2 are not the same object");
} else {
System.out.println("str1 and str2 are the same object");
}
}
}
Output:
str1 and str2 are not the same object
In this case, even though str1
and str2
contain the same string value, they are two separate objects in memory. Hence, the !=
operator returns true
, indicating that they are not the same object. This distinction is vital when working with Java objects, as it emphasizes the importance of understanding how references work.
Using the .equals()
Method for Content Comparison
While the !=
operator checks for reference inequality, the .equals()
method is used to compare the content of objects. This method is particularly useful for comparing strings and other complex objects. It’s essential to use .equals()
when you want to determine if two objects are logically equivalent rather than just checking if they are the same instance.
Here’s an example that illustrates this:
public class EqualsMethodExample {
public static void main(String[] args) {
String str1 = new String("Hello");
String str2 = new String("Hello");
if (!str1.equals(str2)) {
System.out.println("str1 and str2 are not equal in content");
} else {
System.out.println("str1 and str2 are equal in content");
}
}
}
Output:
str1 and str2 are equal in content
In this example, we use the .equals()
method to compare the contents of str1
and str2
. Since both strings contain the same text, the output indicates that they are equal in content. This showcases the critical difference between using !=
and .equals()
, emphasizing that !=
checks for reference equality, while .equals()
checks for value equality.
Summary of Not Equals in Java
Understanding the concept of not equals in Java is essential for any programmer. The !=
operator serves as a straightforward way to check if two values or references are not equal. However, when dealing with objects, it’s crucial to remember that !=
checks for reference inequality, while the .equals()
method checks for content equality. This knowledge will help you write more effective and bug-free Java code.
In summary, whether you’re comparing primitive types or objects, knowing when and how to use the !=
operator versus the .equals()
method can significantly impact the functionality of your Java applications.
Conclusion
In conclusion, mastering the not equals concept in Java is a fundamental skill for any developer. The !=
operator and the .equals()
method serve distinct purposes, and understanding their differences is key to writing robust code. By applying these concepts correctly, you can ensure that your Java applications behave as expected, leading to cleaner, more efficient programming. Keep practicing these comparisons, and you’ll become more proficient in Java programming in no time.
FAQ
-
what is the difference between
!=
and.equals()
in Java?
The!=
operator checks if two references point to different objects in memory, while.equals()
checks if the contents of two objects are logically equivalent. -
can
!=
be used with primitive data types?
Yes, the!=
operator can be used with primitive data types to compare their values directly. -
why is it important to use
.equals()
for object comparison?
Using.equals()
is important because it checks for value equality, ensuring that two objects with the same content are recognized as equal. -
what happens if you use
!=
on two strings with the same content?
If you use!=
on two string objects with the same content, it will returntrue
if they are different instances in memory, even if the content is the same. -
how do I compare two objects for equality in Java?
You can compare two objects for equality using the.equals()
method, which checks if the objects are logically equivalent based on their content.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn