Multiple Classes in One File in Java
- Introduction to Multiple Classes in One File
- Multiple Classes in One File with Nested Classes
- Multiple Classes in One File with Non-Nested Classes
- Best Practices When Having Multiple Classes in One File
- Conclusion
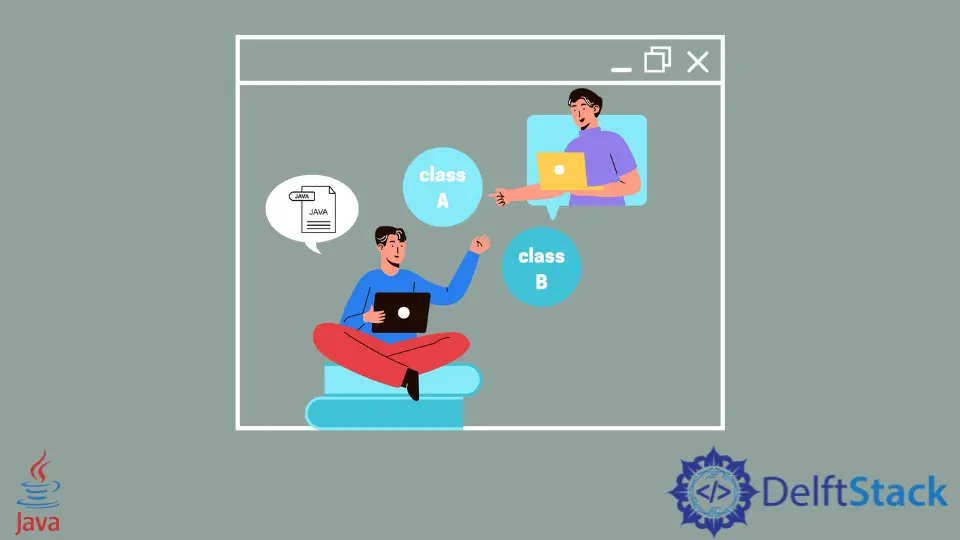
It is possible to have multiple classes in one file in Java, allowing for logical grouping and organization of related functionalities within a single source file.
We’ll explore how to structure and manage multiple classes in a single Java file, considering best practices for clarity and maintainability in your codebase.
Introduction to Multiple Classes in One File
In Java programming, it’s common to organize code into separate classes, with each class typically residing in its own file. However, there are scenarios where it makes sense to have multiple classes within a single Java file.
Using multiple classes in one file in Java is often done for simplicity and when the classes are closely related or serve complementary purposes. It can make the codebase more compact and easier to manage, especially for small utility classes or when certain classes are tightly coupled.
This approach is convenient when the classes are not expected to be reused in other parts of the application, and it helps maintain a focused and cohesive structure within the code. However, it’s crucial to balance simplicity with the principles of code organization and readability.
Multiple Classes in One File with Nested Classes
Nested classes are used for multiple classes in one file because they allow logical grouping of related classes, improving code organization. This method keeps closely associated classes together, enhancing readability and maintainability.
Other techniques, like having separate files for each class, may lead to scattered code and make it harder to comprehend the relationships between classes. Nested classes provide a convenient way to encapsulate functionality within a single file while maintaining a clear and cohesive structure.
Consider a scenario where you’re developing a simple banking application. You might have a main class representing an account and within it, nested classes for various functionalities such as transactions and interest calculations.
Take note that if you define classes within another class, and those classes are not declared as static
, they are considered inner classes. Therefore, inner classes are a subset of nested classes.
While all inner classes are nested classes, not all nested classes are necessarily inner classes. The specific terminology used may vary, but in practice, they often refer to the same concept of defining classes within another class for improved encapsulation and organization.
Let’s explore this with a complete working code example:
public class BankAccount {
// Main class representing a bank account
private String accountNumber;
private double balance;
// Constructor
public BankAccount(String accountNumber, double balance) {
this.accountNumber = accountNumber;
this.balance = balance;
}
// Nested class for transactions
class Transactions {
public void deposit(double amount) {
balance += amount;
System.out.println("Deposited: $" + amount);
}
public void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
System.out.println("Withdrawn: $" + amount);
} else {
System.out.println("Insufficient funds");
}
}
}
// Nested class for interest calculations
class InterestCalculator {
private double interestRate;
// Constructor
public InterestCalculator(double interestRate) {
this.interestRate = interestRate;
}
public void calculateInterest() {
double interest = balance * interestRate / 100;
balance += interest;
System.out.println("Interest Calculated: $" + interest);
}
}
// Main method
public static void main(String[] args) {
// Create a BankAccount object
BankAccount account = new BankAccount("123456", 1000);
// Accessing nested classes
BankAccount.Transactions transactions = account.new Transactions();
BankAccount.InterestCalculator interestCalculator = account.new InterestCalculator(5);
// Performing transactions
transactions.deposit(500);
transactions.withdraw(200);
// Calculating interest
interestCalculator.calculateInterest();
}
}
In this example, we start with a BankAccount
class representing a basic bank account. Inside this class, we have two nested classes: Transactions
and InterestCalculator
.
These nested classes encapsulate transaction-related functionalities and interest calculations, respectively.
In the main
method, we demonstrate how to use nested classes by creating an instance of BankAccount
and accessing its nested classes. We perform transactions such as deposits and withdrawals using the Transactions
class and calculate interest using the InterestCalculator
class.
Output:
The output demonstrates the effective organization of code using nested classes. Each aspect of the bank account functionality is encapsulated within its respective nested class, resulting in a clean and modular code structure.
Utilizing nested classes for multiple classes in one file is a powerful tool for enhancing code organization. It promotes a logical structure, improves readability, and encapsulates related functionality within a single Java file.
As demonstrated in our banking example, this approach proves beneficial in creating well-structured and maintainable code. Consider incorporating nested classes into your Java programming arsenal to streamline your code and make it more intuitive for yourself and your fellow developers.
Multiple Classes in One File with Non-Nested Classes
Non-nested classes are used for multiple classes in one file to maintain a straightforward and modular code structure. This approach allows developers to separate distinct functionalities into independent classes within the same file, promoting clarity and ease of maintenance.
Unlike other methods, such as nested classes or separate files for each class, non-nested classes offer a simple organization while keeping related functionalities neatly encapsulated. This method is beneficial for scenarios where a file contains multiple standalone classes with specific roles.
Let’s dive into a real-world scenario to better understand the utility of non-nested classes. Imagine developing a multimedia application where you handle both audio and video functionalities.
In this case, having separate non-nested classes for audio and video processing within a single file can offer a clear and modular code structure. Let’s explore this concept through a complete working code example:
// Main class representing the multimedia application
public class MultimediaApp {
public static void main(String[] args) {
// Creating instances of non-nested classes
AudioProcessor audioProcessor = new AudioProcessor();
VideoProcessor videoProcessor = new VideoProcessor();
// Using methods from non-nested classes
audioProcessor.playAudio("background_music.mp3");
videoProcessor.playVideo("intro_video.mp4");
}
}
// Non-nested class for audio processing
class AudioProcessor {
public void playAudio(String audioFile) {
System.out.println("Playing audio: " + audioFile);
// Audio processing logic goes here
}
}
// Non-nested class for video processing
class VideoProcessor {
public void playVideo(String videoFile) {
System.out.println("Playing video: " + videoFile);
// Video processing logic goes here
}
}
In this example, we have a MultimediaApp
class representing our multimedia application. Additionally, we’ve created two non-nested classes within the same file: AudioProcessor
and VideoProcessor
. Each non-nested class encapsulates functionalities related to audio and video processing, respectively.
In the main
method, we create instances of these non-nested classes and invoke their methods. The AudioProcessor
plays an audio file, and the VideoProcessor
plays a video file.
The MultimediaApp
class is the main class for our multimedia application and holds the main
method to start the application. The AudioProcessor
class, a non-nested class, manages audio processing and includes a playAudio
method for playing audio files.
Similarly, the VideoProcessor
class, also non-nested, takes care of video processing and features a playVideo
method for playing video files. Each class has a specific role, contributing to a well-organized and modular code structure.
Output:
The use of non-nested classes within a single Java file is a valuable technique for organizing code, especially when dealing with related functionalities. In the multimedia application example, having separate classes for audio and video processing enhances code modularity and readability.
By adopting this method, you can create a more maintainable and logically structured codebase, simplifying both development and collaboration efforts. Consider integrating non-nested classes into your Java programming practices for improved code organization and clarity.
Best Practices When Having Multiple Classes in One File
Single Responsibility Principle
Each class within the file should have a single responsibility. This ensures that each class is focused on a specific task or functionality, promoting better maintainability and readability.
Cohesiveness
Ensure that the classes grouped together in the same file are related and share a common purpose or functionality. This helps in maintaining a clear and logical structure within the codebase.
Accessibility Modifiers
Use appropriate access modifiers (public, private, protected) to control the visibility of classes. Typically, only one class in the file should be marked as public
, and the others should be package-private or private, depending on the encapsulation needs.
File Naming
The file name should reflect the primary functionality or purpose of the public class within it. Follow Java naming conventions, such as using CamelCase for class names and matching the file name with the public class name.
Class Order
Consider organizing the classes within the file in a logical order, such as placing utility classes or helper classes at the bottom. This can help developers understand the flow of the file more easily.
Documentation
Provide clear and concise comments or documentation for each class, explaining its purpose, usage, and any important details. This helps other developers (or even yourself in the future) understand the intent of each class.
Avoiding Code Smells
Ensure that the file does not violate common code smells like having too many responsibilities in a single class or violating the Single Responsibility Principle. If the file becomes too large or complex, consider refactoring into separate files or classes.
Testing Considerations
If applicable, ensure that your testing strategy aligns with the organization of classes. For example, unit tests should focus on specific classes, and it should be clear which classes are being tested within a given test file.
Maintain Consistency
Follow consistent coding style and formatting throughout the file. Consistency makes the code more readable and helps maintain a professional and unified codebase.
Version Control
Consider the impact on version control. Try to avoid situations where multiple developers need to work on the same file simultaneously, as it may lead to merge conflicts. If necessary, use version control tools efficiently to manage collaborative development.
Conclusion
Mastering the art of organizing multiple classes within a single Java file is pivotal for maintaining clear and efficient code. Whether through the use of nested or non-nested classes, developers can achieve a well-structured and modular codebase.
Adopting best practices, such as adhering to the Single Responsibility Principle, ensuring accessibility modifiers, and maintaining consistent file naming, further enhances code readability and maintainability. By understanding these techniques and principles, developers can strike a balance between simplicity and organization, creating Java applications that are not only functional but also easy to comprehend and maintain.