Java에서 하나의 파일에 있는 여러 클래스
Siddharth Swami
2023년10월12일
Java
Java Class
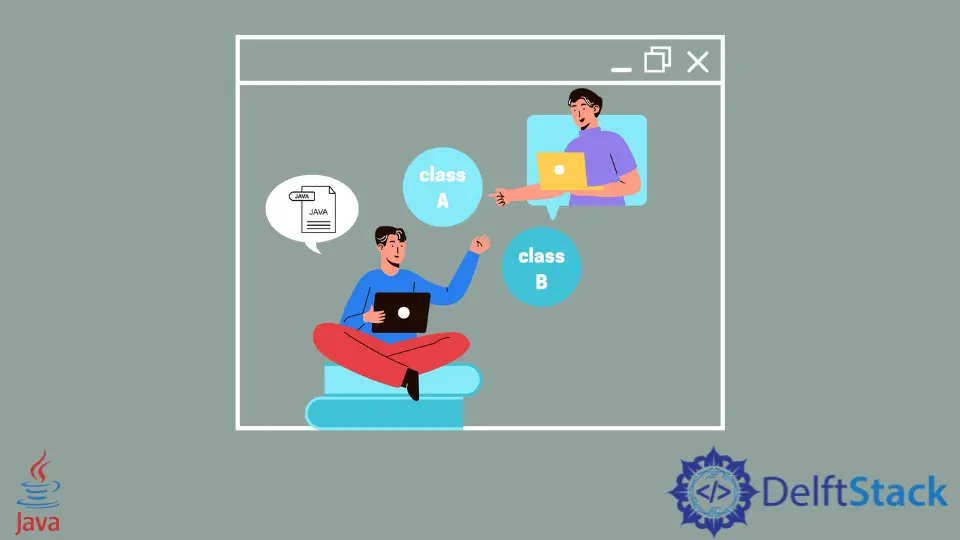
이 기사에서는 Java의 단일 파일에 있는 여러 클래스에 대해 설명합니다.
하나의 단일 파일에 여러 클래스를 선언하는 방법에는 여러 가지가 있습니다. 이를 구현하는 두 가지 표준 방법에 대해 논의할 것입니다.
중첩 클래스를 사용하여 Java의 단일 파일에 여러 클래스 포함
이 방법에서 클래스는 클래스 내에서 정의됩니다. 이 방법을 사용하면 한 곳에서만 사용해야 하는 클래스를 논리적으로 그룹화할 수 있습니다.
이들은 두 가지 범주로 나뉩니다. 첫 번째는 static으로 선언된 정적 중첩 클래스이고, 다른 하나는 비정적 유형의 내부 클래스입니다.
아래 코드는 이 방법을 보여줍니다.
class Outer_Class {
// static member
static int x = 20;
// instance(non-static) member
int y = 20;
private static int outer_private = 40;
// static nested class
static class StaticNestedClass {
void display() {
System.out.println("outer_x = " + x);
System.out.println("outer_private = " + outer_private);
}
}
}
public class NestedDemo {
public static void main(String[] args) {
// accessing a static nested class
Outer_Class.StaticNestedClass nestedObject = new Outer_Class.StaticNestedClass();
nestedObject.display();
}
}
출력:
outer_x = 20
outer_private = 40
Java의 단일 파일에서 여러 개의 중첩되지 않은 클래스 사용
Java의 단일 파일에 여러 개의 중첩되지 않은 클래스를 가질 수도 있습니다.
다음 예를 통해 이를 더 잘 이해할 수 있습니다.
public class Phone {
Phone() {
System.out.println("Constructor of phone class.");
}
void phone_method() {
System.out.println("Phone battery is low");
}
public static void main(String[] args) {
Phone p = new Phone();
Laptop l = new Laptop();
p.phone_method();
l.laptop_method();
}
}
class Laptop {
Laptop() {
System.out.println("Constructor of Laptop class.");
}
void laptop_method() {
System.out.println("Laptop battery is low");
}
}
출력:
Constructor of phone class.
Constructor of Laptop class.
Phone battery is low
Laptop battery is low
위의 예에서 프로그램은 두 개의 클래스로 구성되어 있습니다. 첫 번째는 Phone
클래스이고 두 번째는 Laptop
클래스입니다.
두 클래스 모두 함수를 실행하기 위한 생성자와 메서드가 있습니다. 프로그램을 실행할 때 두 클래스에 대해 두 개의 .class
파일이 생성됩니다. 코드를 다시 컴파일하지 않고도 .class
파일을 다시 사용할 수 있습니다. 출력에서 코드가 성공적으로 실행되었음을 확인할 수 있습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다