Difference Between Long and Int Data Types in Java
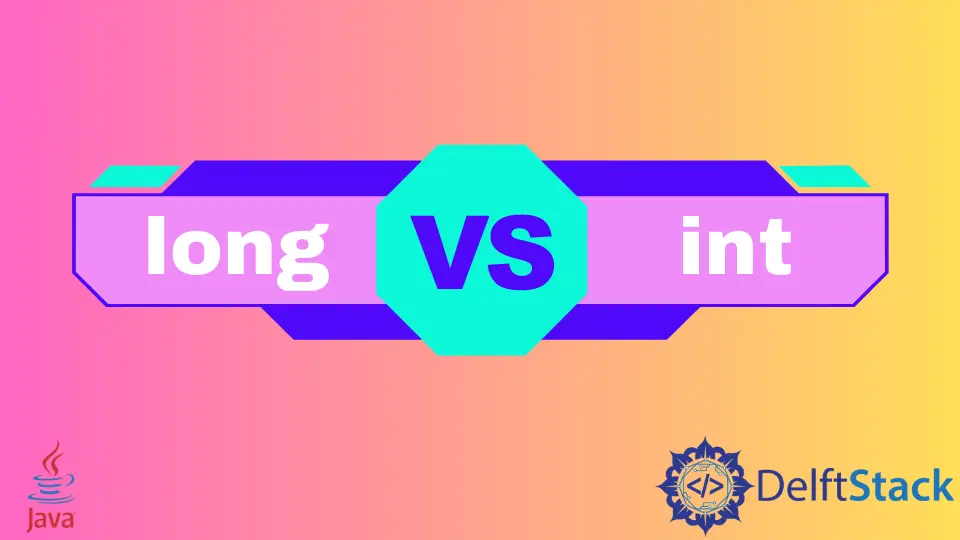
This article will discuss the differences between the int
and long
data types in Java. We will understand the differences between these data types and will be able to decide which one to use according to the scenario.
the int
and long
Data Types in Java
In the realm of Java programming, choosing the right data type is crucial for effective memory utilization and accurate representation of values. Among the various primitive data types, int
and long
are two commonly used types for handling integer values.
This article delves into the distinctions between these two data types, exploring aspects such as size, range of values, default literals, and appropriate usage.
In Java, int
and long
are both primitive data types used to represent integer values, but they differ in terms of size and the range of values they can store. Here are the key differences between int
and long
data types in Java:
Aspect | int |
long |
---|---|---|
Size | 32 bits | 64 bits |
Range of Values | -2^31 to 2^31-1 | -2^63 to 2^63-1 |
Default Literals | Integer literals without suffix default to int . |
To specify a literal as long , append L or l . Example: long longValue = 123L; |
Usage | Conserve memory for smaller values. | Accommodate larger values beyond int range. |
Keep in mind that using long
for all integer values is unnecessary and can lead to increased memory usage. It’s generally a good practice to choose the smallest data type that can accommodate the range of values you expect to work with.
Code Example that Illustrates the Difference between int
and long
in Java:
public class IntVsLongExample {
public static void main(String[] args) {
// Using int
int intValue = 100;
// Using long with "L" suffix
long longValue = 10000000000L;
// Performing arithmetic operations
int result = intValue * 2;
long longResult = longValue * 2;
// Displaying results
System.out.println("Result (int): " + result);
System.out.println("Result (long): " + longResult);
}
}
Let’s dissect the code step by step, unraveling the intricacies of int
and long
.
In the code example provided, we begin by declaring an int
variable named intValue
and initializing it with the value 100
. Similarly, we declare a long
variable named longValue
and set its value to 10000000000L
, with the L
suffix indicating it as a long
literal.
Moving on to arithmetic operations, we conduct simple calculations. For the int
variable, we double its value, and for the long
variable, we perform the same operation of doubling the value.
These operations serve to illustrate the fundamental usage of int
and long
data types.
Finally, the results of these operations are displayed. The output provides a clear demonstration of how the chosen data type influences the computed values. This straightforward and concise code walkthrough aims to enhance understanding of the distinctions between int
and long
in Java programming.
The output of the provided code will be:
Result (int): 200
Result (long): 20000000000
This output affirms the expected behavior, highlighting the multiplication of the respective integer values. The int
result remains within its range, while the long
result comfortably handles larger values.
This practical example solidifies the understanding of when to opt for int
and when to leverage the capabilities of long
in Java programming.
the Integer
and Long
Wrapper Class in Java
In Java, Integer
and Long
are wrapper classes for primitive data types int
and long
. They serve as objects that encapsulate these primitives, providing additional methods and allowing integration with object-oriented features.
While int
and long
are primitive data types representing integers, Integer
and Long
offer more functionality, including methods for conversions and operations. The key distinction lies in their use as objects, offering advantages such as compatibility with Java collections and additional utility methods compared to the simpler, memory-efficient primitive counterparts int
and long.
In Java, Integer
and Long
are wrapper classes for the primitive data types int
and long
, respectively. Here are the key differences between Integer
and Long
:
Aspect | Integer |
Long |
---|---|---|
Underlying Primitive Type | Wraps the primitive data type int . |
Wraps the primitive data type long . |
Size | 32 bits, encapsulating the int data type. |
64 bits, encapsulating the larger long data type. |
Range of Values | Can represent integer values in the range of -2^31 to 2^31-1. | Can represent integer values in the range of -2^63 to 2^63-1. |
Methods and Usage | Offering functions for string conversion, arithmetic operations, and additional utilities, Integer is commonly chosen for smaller integer values and interactions with APIs expecting objects. |
Provide methods for converting to and from strings, performing arithmetic operations, and other utility functions. Long is employed when dealing with larger integer values that exceed the range of int or when precise representation of long integers is required. |
Literal Values | Literal values of type int can be automatically converted to Integer through autoboxing. |
Literal values of type long require an L or l suffix, and autoboxing is applied to convert them to Long . |
Example:
// Integer Example
Integer intWrapper = 42;
int intValue = intWrapper.intValue(); // Extracting the primitive int value
// Long Example
Long longWrapper = 123456789L;
long longValue = longWrapper.longValue(); // Extracting the primitive long value
The provided Java code demonstrates the creation of Integer
and Long
wrapper objects, followed by extracting their respective primitive values.
These lines will print the extracted primitive values of intWrapper
and longWrapper
.
Understanding these differences is important when choosing between Integer
and Long
based on the size and range of the integer values you need to work with in your Java code.
Conclusion
The distinction between int
and long
lies in their size and range, with int
being 32 bits for smaller values and long
being 64 bits for larger integers. On the other hand, Integer
and Long
are wrapper classes, providing additional functionality and object-oriented features, advantageous in scenarios requiring objects instead of primitives.
The choice between them depends on the specific requirements of a program, balancing memory efficiency with the need for extended numerical capacity.