How to Create Unsigned Long in Java
-
Create Unsigned Long in Java Using the
BigInteger
Class -
Create Unsigned Long in Java Using
ULong
of thejOOU
Library - Conclusion
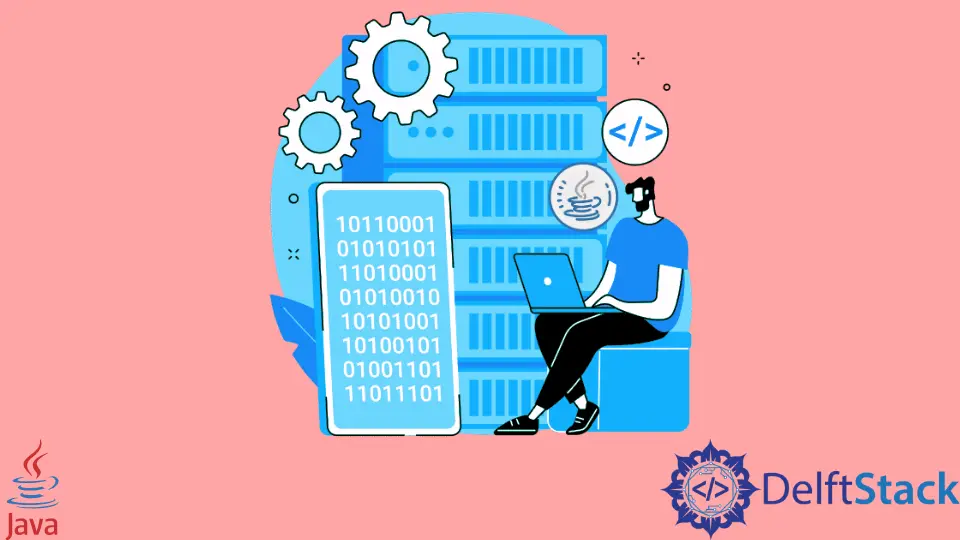
Java, by default, does not support native unsigned data types, including unsigned long. However, developers often encounter scenarios where working with unsigned values is necessary.
In this article, we’ll explore multiple approaches to creating an unsigned long in Java, including using BigInteger
, the ULong
class from the jOOU
library, and bitwise operations.
Create Unsigned Long in Java Using the BigInteger
Class
The BigInteger
class in Java is found in the java.math package
. It provides a flexible solution for working with arbitrarily large integers, including unsigned long values.
To create an unsigned long using BigInteger
, you can follow a simple process. Begin by creating a BigInteger
object and initialize it with the maximum value representable by a signed long
using Long.MAX_VALUE
.
BigInteger bigInteger = BigInteger.valueOf(Long.MAX_VALUE);
Next, use the add()
method to add the specific value you want to store as an unsigned long.
bigInteger = bigInteger.add(BigInteger.valueOf(25634));
Finally, convert the BigInteger
back to a signed long
using longValue()
. Then, use Long.toUnsignedString()
to obtain the string representation of the unsigned value.
long longValue = bigInteger.longValue();
String longAsUnsignedString = Long.toUnsignedString(longValue);
System.out.println(longAsUnsignedString);
Putting it all together:
import java.math.BigInteger;
public class UnsignedLongExample {
public static void main(String[] args) {
BigInteger bigInteger = BigInteger.valueOf(Long.MAX_VALUE);
bigInteger = bigInteger.add(BigInteger.valueOf(25634));
long longValue = bigInteger.longValue();
String longAsUnsignedString = Long.toUnsignedString(longValue);
System.out.println(longAsUnsignedString);
}
}
In this example, we start by creating a BigInteger
object (bigInteger
) initialized with the maximum signed long value. We then add a specific value (in this case, 25634
) to it.
The resulting BigInteger
is converted back to a signed long
using bigInteger.longValue()
. Finally, to obtain the unsigned string representation, we use Long.toUnsignedString()
.
The printed output confirms the successful creation of an unsigned long in Java.
This code, when executed, will output:
9223372036854801441
Note that if we print the longValue
directly, we will get a negative value because long
is still a signed variable. The function toUnsignedString()
converts it to a string with the unsigned value.
import java.math.BigInteger;
public class UnsignedLongExample {
public static void main(String[] args) {
BigInteger bigInteger = BigInteger.valueOf(Long.MAX_VALUE);
bigInteger = bigInteger.add(BigInteger.valueOf(25634));
long longValue = bigInteger.longValue();
System.out.println(longValue);
}
}
Output:
-9223372036854750175
Create Unsigned Long in Java Using ULong
of the jOOU
Library
While Java lacks a native unsigned long data type, the jOOU
library offers a convenient solution with its ULong
class residing in the org.jooq.tools.unsigned
package.
To leverage ULong
for an unsigned long, we start by importing the library into our project. Maven users can include the following dependencies:
<dependency>
<groupId>org.jooq</groupId>
<artifactId>joou</artifactId>
<version>0.9.4</version>
</dependency>
Once the library is imported, we can use the ULong
class to create an unsigned long. In the code example, we create a BigInteger
object and then utilize the ULong.valueOf()
method.
This method takes a signed long value obtained from the BigInteger
and returns a ULong
object, effectively representing an unsigned long.
Here’s a Java code example demonstrating the creation of an unsigned long using ULong
:
import java.math.BigInteger;
import org.joou.ULong;
public class UnsignedLongExample {
public static void main(String[] args) {
BigInteger maxSignedLong = BigInteger.valueOf(Long.MAX_VALUE);
BigInteger unsignedBigInteger = maxSignedLong.add(BigInteger.valueOf(25634));
ULong uLong = ULong.valueOf(unsignedBigInteger.longValue());
System.out.println(uLong);
}
}
In this example, we start by creating a BigInteger
object (maxSignedLong
) with the maximum value representable by a signed long. Subsequently, we add a specific value to this BigInteger
, resulting in unsignedBigInteger
.
To convert this BigInteger
to a signed long, we use unsignedBigInteger.longValue()
. The crucial step follows, where we utilize ULong.valueOf()
to convert the signed long to a ULong
object, effectively creating an unsigned long.
Finally, we print the ULong
representation.
When executed, this code will output:
9223372036854801441
The output confirms the successful creation of an unsigned long using ULong
from the jOOU
library. This approach provides an alternative to using BigInteger
and aligns with scenarios where external libraries can be integrated to address specific programming requirements.
Conclusion
Creating unsigned long integers in Java involves selecting an approach based on the specific requirements and constraints of the project. Whether using BigInteger
for flexibility, leveraging the jOOU
library for convenience, or employing bitwise operations for a manual approach, each method has its strengths and trade-offs.
Carefully consider the specific needs of your application and choose the method that best aligns with those requirements.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn