How to Format Text Field Number Format in JavaFX
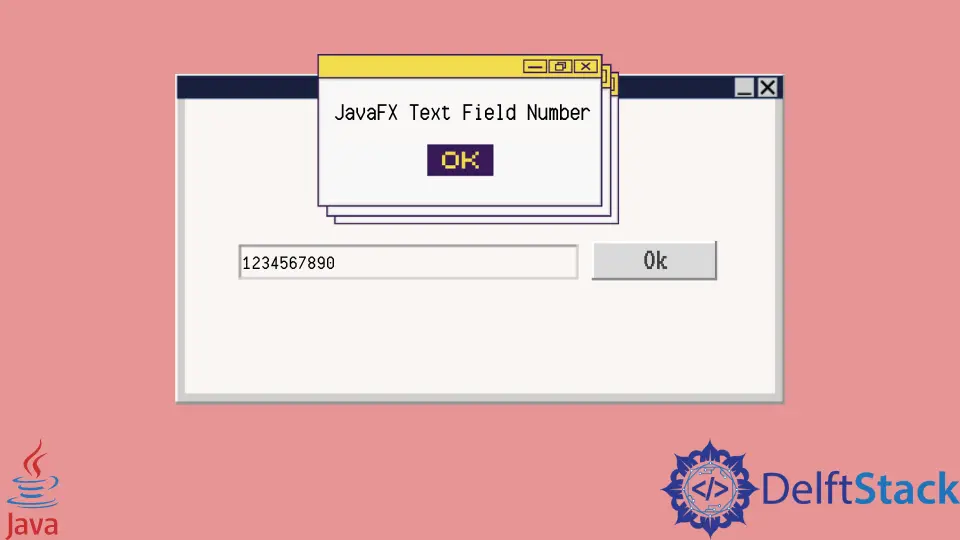
The TextFormatter
class is used to create fields with certain formats. It can also be used to create a numeric text field in JavaFX.
This tutorial demonstrates how to create a numeric text field in JavaFX.
JavaFX Text Field Number Format
JavaFX.scene.control.TextFormatter<V>
is used to create fields with certain formats. The Formatter
describes a format TextInputControl
text with two different structures.
One is a filter getFilter()
to modify the user input, and the other is a value converter getValueConverter()
used to provide a special format that represents a value of type V
. The TextFormatter
can be created with just the filter and value converter.
The syntax for TextFormatter
is:
TextFormatter<Integer> Text_Formatter = new TextFormatter<Integer>(String_Converter, 0, Integer_Filter);
Numeric_Field.setTextFormatter(Text_Formatter);
The TextFormatter
can be an integer or any other type, and the setTextFormatter
method is used to apply the TextFormatter
to the field.
Let’s try an example to create a numeric text field:
package delftstack;
import java.util.function.UnaryOperator;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.control.TextFormatter;
import javafx.scene.control.TextFormatter.Change;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.util.StringConverter;
import javafx.util.converter.IntegerStringConverter;
public class Numeric_TextField extends Application {
@Override
public void start(Stage Demo_Stage) {
TextField Numeric_Field = new TextField();
UnaryOperator<Change> Integer_Filter = change -> {
String Demo_Text = change.getControlNewText();
if (Demo_Text.matches("-?([1-9][0-9]*)?")) {
return change;
} else if ("-".equals(change.getText())) {
if (change.getControlText().startsWith("-")) {
change.setText("");
change.setRange(0, 1);
change.setCaretPosition(change.getCaretPosition() - 2);
change.setAnchor(change.getAnchor() - 2);
return change;
} else {
change.setRange(0, 0);
return change;
}
}
return null;
};
StringConverter<Integer> String_Converter = new IntegerStringConverter() {
@Override
public Integer fromString(String s) {
if (s.isEmpty())
return 0;
return super.fromString(s);
}
};
TextFormatter<Integer> Text_Formatter =
new TextFormatter<Integer>(String_Converter, 0, Integer_Filter);
Numeric_Field.setTextFormatter(Text_Formatter);
// demo listener:
Text_Formatter.valueProperty().addListener(
(obs, oldValue, newValue) -> System.out.println(newValue));
VBox VBox_Root = new VBox(5, Numeric_Field, new Button("Click Here"));
VBox_Root.setAlignment(Pos.CENTER);
Scene scene = new Scene(VBox_Root, 300, 120);
Demo_Stage.setScene(scene);
Demo_Stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
The code above uses a UnaryOperator
for the filter and StringConverter
as a value. The code uses TextFormatter
to create a text field that will only take the integer values.
See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook