Formato de número de campo de texto JavaFX
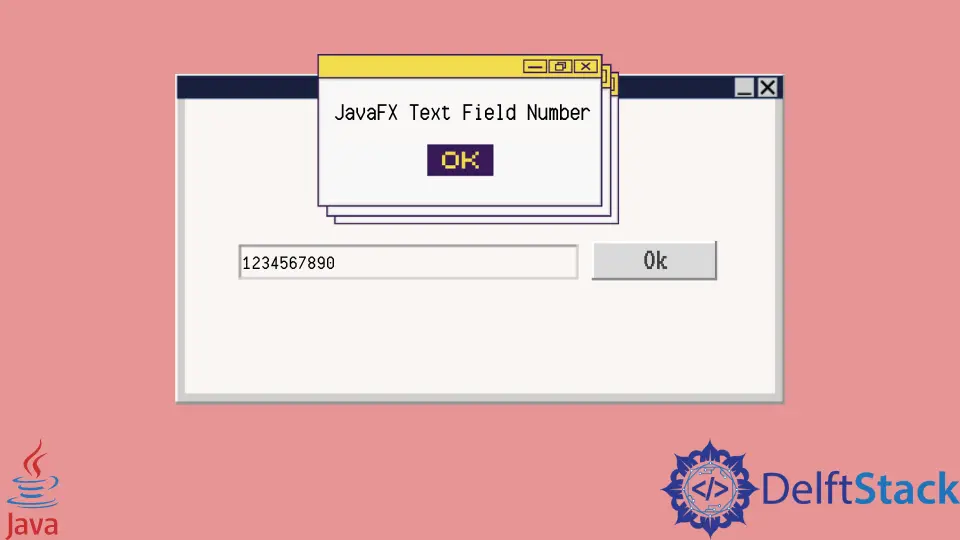
La clase TextFormatter
se utiliza para crear campos con determinados formatos. También se puede usar para crear un campo de texto numérico en JavaFX.
Este tutorial demuestra cómo crear un campo de texto numérico en JavaFX.
Formato numérico de campo de texto JavaFX
JavaFX.scene.control.TextFormatter<V>
se usa para crear campos con ciertos formatos. El Formatter
describe un formato de texto TextInputControl
con dos estructuras diferentes.
Uno es un filtro getFilter()
para modificar la entrada del usuario, y el otro es un convertidor de valores getValueConverter()
que se utiliza para proporcionar un formato especial que representa un valor de tipo V
. El TextFormatter
se puede crear solo con el filtro y el convertidor de valores.
La sintaxis de TextFormatter
es:
TextFormatter<Integer> Text_Formatter = new TextFormatter<Integer>(String_Converter, 0, Integer_Filter);
Numeric_Field.setTextFormatter(Text_Formatter);
El TextFormatter
puede ser un número entero o cualquier otro tipo, y el método setTextFormatter
se usa para aplicar el TextFormatter
al campo.
Probemos un ejemplo para crear un campo de texto numérico:
package delftstack;
import java.util.function.UnaryOperator;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.control.TextFormatter;
import javafx.scene.control.TextFormatter.Change;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.util.StringConverter;
import javafx.util.converter.IntegerStringConverter;
public class Numeric_TextField extends Application {
@Override
public void start(Stage Demo_Stage) {
TextField Numeric_Field = new TextField();
UnaryOperator<Change> Integer_Filter = change -> {
String Demo_Text = change.getControlNewText();
if (Demo_Text.matches("-?([1-9][0-9]*)?")) {
return change;
} else if ("-".equals(change.getText())) {
if (change.getControlText().startsWith("-")) {
change.setText("");
change.setRange(0, 1);
change.setCaretPosition(change.getCaretPosition() - 2);
change.setAnchor(change.getAnchor() - 2);
return change;
} else {
change.setRange(0, 0);
return change;
}
}
return null;
};
StringConverter<Integer> String_Converter = new IntegerStringConverter() {
@Override
public Integer fromString(String s) {
if (s.isEmpty())
return 0;
return super.fromString(s);
}
};
TextFormatter<Integer> Text_Formatter =
new TextFormatter<Integer>(String_Converter, 0, Integer_Filter);
Numeric_Field.setTextFormatter(Text_Formatter);
// demo listener:
Text_Formatter.valueProperty().addListener(
(obs, oldValue, newValue) -> System.out.println(newValue));
VBox VBox_Root = new VBox(5, Numeric_Field, new Button("Click Here"));
VBox_Root.setAlignment(Pos.CENTER);
Scene scene = new Scene(VBox_Root, 300, 120);
Demo_Stage.setScene(scene);
Demo_Stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
El código anterior usa un Operador Unario para el filtro y StringConverter
como valor. El código usa TextFormatter
para crear un campo de texto que solo tomará los valores enteros.
Ver salida:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook