The setCellValueFactory Method in JavaFX
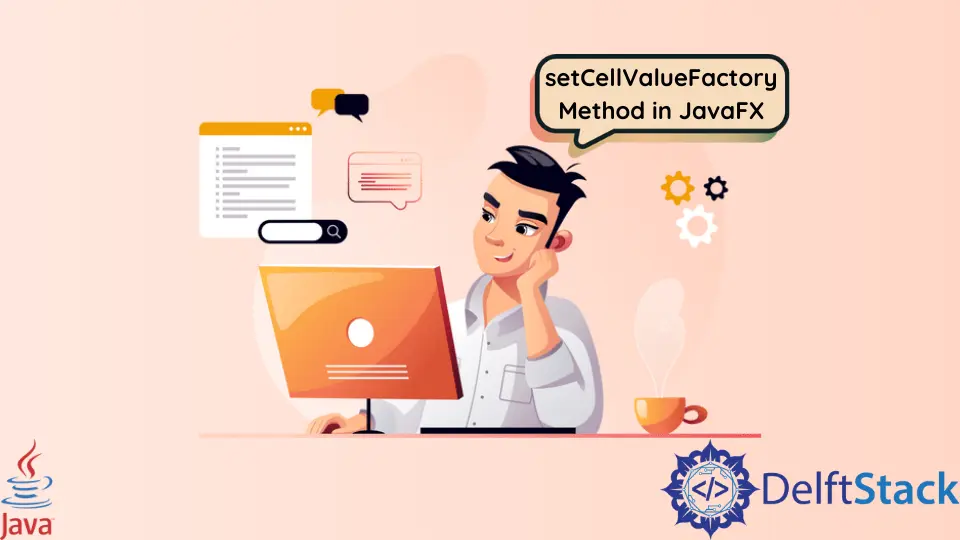
TableView
is a very important part of any UI; it helps to visualize data for users. When working with the table, we use the most common method, setCellValueFactory()
, for creating a cell on the table.
In this article, we will discuss this method and see an example with an explanation.
Use the setCellValueFactory
Method in JavaFX
In our below example, we have created a simple table with some data. The code for our example will look like the following.
// Importing all necessary packages
import javafx.application.Application;
import javafx.beans.property.*;
import javafx.collections.*;
import javafx.event.*;
import javafx.geometry.Insets;
import javafx.scene.*;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.VBox;
import javafx.scene.text.Font;
import javafx.stage.Stage;
public class FXtable extends Application {
private TableView table = new TableView(); // Creating a table with a static class "Person"
private final ObservableList data =
FXCollections.observableArrayList(); // Creating an observable list
private void initData() { // Method that set data to table
data.setAll(
// All table datas
new Person("Alen", "Smith", "alen.smith@example.com"),
new Person("Stefen", "Johnson", "stefen@example.com"),
new Person("Uri", "Gagrin", "uri@example.com"),
new Person("Alex", "Jones", "alex@example.com"),
new Person("Hexa", "Brown", "hexa@example.com"));
}
public void start(Stage stage) {
initData(); // Set initial data to table
stage.setTitle("JavaFx Table View"); // Set the title of the table
stage.setWidth(450); // Set the width
stage.setHeight(500); // Set the height
Label label = new Label("Simple Address Table"); // Create a label
label.setFont(new Font("Arial", 20)); // Set the font and font size
TableColumn FirstNameCol = new TableColumn("First Name"); // Create a column named "First Name"
FirstNameCol.setMinWidth(100); // Set the minimum column width to 100
FirstNameCol.setCellValueFactory(
new PropertyValueFactory("firstName")); // Populate all the column data for "First Name"
TableColumn LastNameCol = new TableColumn("Last Name"); // Create a column named "Last Name"
LastNameCol.setMinWidth(100); // Set the minimum column width to 100
LastNameCol.setCellValueFactory(
new PropertyValueFactory("lastName")); // Populate all the column data for "Last Name"
TableColumn EmailColl = new TableColumn("Email"); // Create a column named "Email"
EmailColl.setMinWidth(200); // Set the minimum column width to 200
EmailColl.setCellValueFactory(
new PropertyValueFactory("email")); // Populate all the column data for "Last Name"
table.setItems(data);
table.getColumns().addAll(FirstNameCol, LastNameCol, EmailColl); // Add columns to table
table.setPrefHeight(300); // Set table height
final VBox vbox = new VBox(10); // Create a VBox
vbox.setPadding(new Insets(10, 0, 0, 10)); // Add padding
vbox.getChildren().addAll(label, table); // Organize the VBox with label and table
stage.setScene(new Scene(new Group(vbox))); // Add the VBox to scene
stage.show(); // Visualize the scene
}
public static class Person { // Class for creating the person table
private StringProperty FirstName;
private StringProperty LastName;
private StringProperty email;
private Person(String FName, String LName, String email) {
this.FirstName = new SimpleStringProperty(FName);
this.LastName = new SimpleStringProperty(LName);
this.email = new SimpleStringProperty(email);
}
public String getFirstName() { // Method to get First Name
return FirstName.get();
}
public void setFirstName(String FName) { // Method to set First Name
FirstName.set(FName);
}
public StringProperty FirstNameProperty() { // Method to add First Name property
return FirstName;
}
public String getLastName() { // Method to get Last Name
return LastName.get();
}
public void setLastName(String LName) { // Method to set Last Name
LastName.set(LName);
}
public StringProperty lastNameProperty() { // Method to add Last Name property
return LastName;
}
public String getEmail() { // Method to get Email
return email.get();
}
public void setEmail(String inMail) { // Method to set Email
email.set(inMail);
}
public StringProperty emailProperty() { // Method to add Email property
return email;
}
}
public static void main(String[] args) {
launch(args);
} // Launch the application.
}
We already commented in the code on the purpose of each line. Now, we will just discuss about the setCellValueFactory()
function here.
Through the lines FirstNameCol.setCellValueFactory(new PropertyValueFactory("firstName"));
, LastNameCol.setCellValueFactory(new PropertyValueFactory("lastName"));
, and EmailColl.setCellValueFactory(new PropertyValueFactory("email"));
, we used the setCellValueFactory()
method for creating cells in our table.
Here, we created three columns named firstName
, lastName
, and email
. This method specifies how to populate a table’s cells within a single table column.
It provides the TableColumn.CellDataFeatures
instance and returns the ObservableValue
instance. After compiling the above example code and running it in your environment, you will get the below output.
Output:
Remember, if your IDE doesn’t support the automatic inclusion of libraries and packages, you may need to manually include these necessary libraries and packages before compiling.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn