JavaFX의 setCellValueFactory 메소드
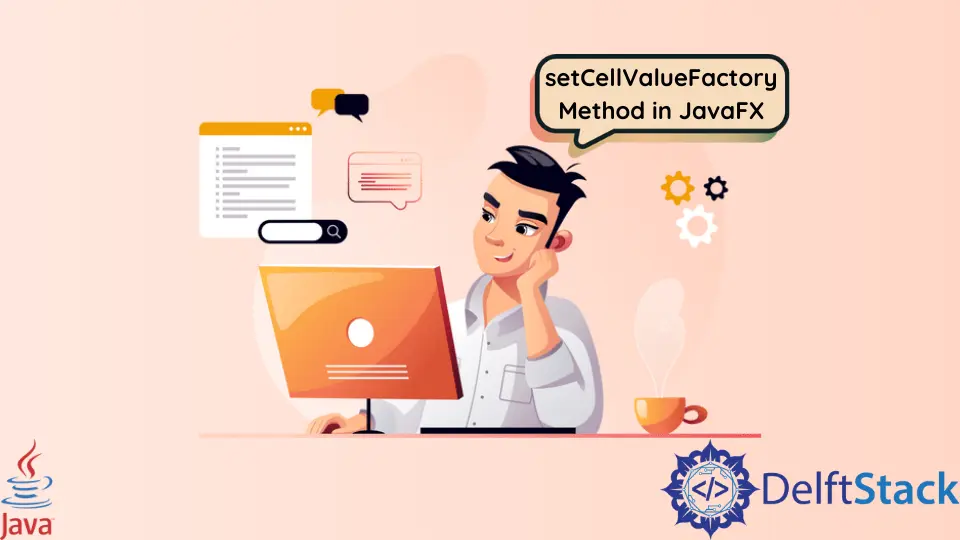
TableView
는 모든 UI에서 매우 중요한 부분입니다. 사용자를 위해 데이터를 시각화하는 데 도움이 됩니다. 테이블 작업을 할 때 테이블에 셀을 생성하기 위해 가장 일반적인 메서드인 setCellValueFactory()
를 사용합니다.
이 기사에서는 이 방법에 대해 설명하고 설명과 함께 예를 볼 것입니다.
JavaFX에서 setCellValueFactory
메서드 사용
아래 예제에서는 일부 데이터가 포함된 간단한 테이블을 만들었습니다. 예제의 코드는 다음과 같습니다.
// Importing all necessary packages
import javafx.application.Application;
import javafx.beans.property.*;
import javafx.collections.*;
import javafx.event.*;
import javafx.geometry.Insets;
import javafx.scene.*;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.VBox;
import javafx.scene.text.Font;
import javafx.stage.Stage;
public class FXtable extends Application {
private TableView table = new TableView(); // Creating a table with a static class "Person"
private final ObservableList data =
FXCollections.observableArrayList(); // Creating an observable list
private void initData() { // Method that set data to table
data.setAll(
// All table datas
new Person("Alen", "Smith", "alen.smith@example.com"),
new Person("Stefen", "Johnson", "stefen@example.com"),
new Person("Uri", "Gagrin", "uri@example.com"),
new Person("Alex", "Jones", "alex@example.com"),
new Person("Hexa", "Brown", "hexa@example.com"));
}
public void start(Stage stage) {
initData(); // Set initial data to table
stage.setTitle("JavaFx Table View"); // Set the title of the table
stage.setWidth(450); // Set the width
stage.setHeight(500); // Set the height
Label label = new Label("Simple Address Table"); // Create a label
label.setFont(new Font("Arial", 20)); // Set the font and font size
TableColumn FirstNameCol = new TableColumn("First Name"); // Create a column named "First Name"
FirstNameCol.setMinWidth(100); // Set the minimum column width to 100
FirstNameCol.setCellValueFactory(
new PropertyValueFactory("firstName")); // Populate all the column data for "First Name"
TableColumn LastNameCol = new TableColumn("Last Name"); // Create a column named "Last Name"
LastNameCol.setMinWidth(100); // Set the minimum column width to 100
LastNameCol.setCellValueFactory(
new PropertyValueFactory("lastName")); // Populate all the column data for "Last Name"
TableColumn EmailColl = new TableColumn("Email"); // Create a column named "Email"
EmailColl.setMinWidth(200); // Set the minimum column width to 200
EmailColl.setCellValueFactory(
new PropertyValueFactory("email")); // Populate all the column data for "Last Name"
table.setItems(data);
table.getColumns().addAll(FirstNameCol, LastNameCol, EmailColl); // Add columns to table
table.setPrefHeight(300); // Set table height
final VBox vbox = new VBox(10); // Create a VBox
vbox.setPadding(new Insets(10, 0, 0, 10)); // Add padding
vbox.getChildren().addAll(label, table); // Organize the VBox with label and table
stage.setScene(new Scene(new Group(vbox))); // Add the VBox to scene
stage.show(); // Visualize the scene
}
public static class Person { // Class for creating the person table
private StringProperty FirstName;
private StringProperty LastName;
private StringProperty email;
private Person(String FName, String LName, String email) {
this.FirstName = new SimpleStringProperty(FName);
this.LastName = new SimpleStringProperty(LName);
this.email = new SimpleStringProperty(email);
}
public String getFirstName() { // Method to get First Name
return FirstName.get();
}
public void setFirstName(String FName) { // Method to set First Name
FirstName.set(FName);
}
public StringProperty FirstNameProperty() { // Method to add First Name property
return FirstName;
}
public String getLastName() { // Method to get Last Name
return LastName.get();
}
public void setLastName(String LName) { // Method to set Last Name
LastName.set(LName);
}
public StringProperty lastNameProperty() { // Method to add Last Name property
return LastName;
}
public String getEmail() { // Method to get Email
return email.get();
}
public void setEmail(String inMail) { // Method to set Email
email.set(inMail);
}
public StringProperty emailProperty() { // Method to add Email property
return email;
}
}
public static void main(String[] args) {
launch(args);
} // Launch the application.
}
우리는 이미 각 줄의 목적에 대해 코드에 주석을 달았습니다. 이제 여기서는 setCellValueFactory()
함수에 대해서만 설명하겠습니다.
FirstNameCol.setCellValueFactory(new PropertyValueFactory("firstName"));
, LastNameCol.setCellValueFactory(new PropertyValueFactory("lastName"));
및 EmailColl.setCellValueFactory(new PropertyValueFactory("email")) 라인을 통해;
에서 테이블에 셀을 생성하기 위해 setCellValueFactory()
메서드를 사용했습니다.
여기에서 firstName
, lastName
및 email
이라는 세 개의 열을 만들었습니다. 이 방법은 단일 테이블 열 내에서 테이블의 셀을 채우는 방법을 지정합니다.
TableColumn.CellDataFeatures
인스턴스를 제공하고 ObservableValue
인스턴스를 반환합니다. 위의 예제 코드를 컴파일하고 환경에서 실행하면 아래 출력이 표시됩니다.
출력:
IDE가 라이브러리 및 패키지의 자동 포함을 지원하지 않는 경우 컴파일하기 전에 이러한 필수 라이브러리 및 패키지를 수동으로 포함해야 할 수 있습니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn