Gridpane Alignment in JavaFX
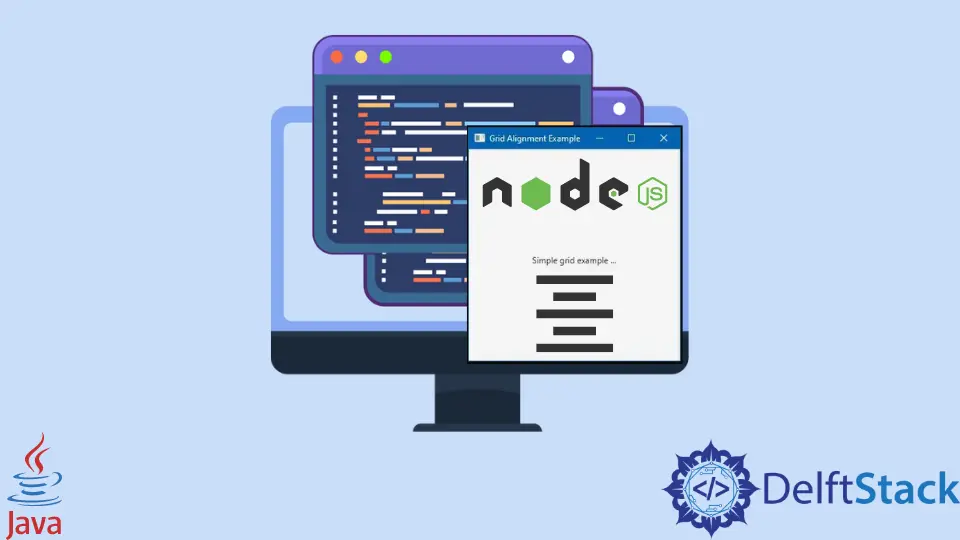
In JavaFX, there is a UI component named GridPane
. Through this UI component, all child nodes are arranged in the form of a grid of columns and rows.
JavaFX provides a powerful layout manager called GridPane
that allows developers to create sophisticated user interfaces by organizing components into a grid of rows and columns. While it offers great flexibility, one of the key aspects of effective grid-based layouts is proper alignment.
In this section, we’ll delve into the GridPane
alignment methods—setValignment
, setHalignment
, setRowSpan
, and setColumnSpan
—and explore how they can be used to control the positioning of nodes within the grid.
GridPane
Alignment in JavaFX
GridPane
alignment in JavaFX refers to the positioning of nodes within a grid layout.
The GridPane
is a versatile grid-based layout that allows developers to organize UI components in rows and columns. Alignment properties in GridPane
include setting the horizontal and vertical alignment for individual nodes or entire rows and columns.
This precise control over alignment ensures a clean and organized presentation of UI elements in JavaFX applications, contributing to a visually appealing user interface.
The four methods—setValignment
, setHalignment
, setRowSpan
, and setColumnSpan
—empower developers to achieve pixel-perfect layouts within the GridPane
.
In JavaFX, the GridPane
layout allows you to create a flexible grid of rows and columns, and you can control the alignment of nodes within the cells. Here are the methods related to alignment in GridPane
:
Method | Description | Parameters | Example Usage |
---|---|---|---|
setHalignment(Node child, HPos value) |
Sets the horizontal alignment of the child node within its grid cell. | - Node child : The node whose alignment you want to set.- HPos value : The horizontal alignment value (HPos.LEFT , HPos.CENTER , or HPos.RIGHT ). |
java GridPane.setHalignment(node, HPos.CENTER); |
setValignment(Node child, VPos value) |
Sets the vertical alignment of the child node within its grid cell. | - Node child : The node whose alignment you want to set.- VPos value : The vertical alignment value (VPos.TOP , VPos.CENTER , or VPos.BOTTOM ). |
java GridPane.setValignment(node, VPos.CENTER); |
setAlignment(Pos value) |
Sets both horizontal and vertical alignment for all children of the GridPane . |
- Pos value : The position value, a combination of horizontal and vertical alignment (e.g., Pos.CENTER ). |
java gridPane.setAlignment(Pos.CENTER); |
setRowSpan(Node child, int value) |
Sets the number of rows that the child node will span. | - Node child : The node whose row span you want to set.- int value : The number of rows to span. |
java GridPane.setRowSpan(node, 2); |
setColumnSpan(Node child, int value) |
Sets the number of columns that the child node will span. | - Node child : The node whose column span you want to set.- int value : The number of columns to span. |
java GridPane.setColumnSpan(node, 3); |
These methods provide a flexible way to control the alignment and positioning of nodes within a GridPane
in JavaFX. You can use them individually or in combination to achieve the desired layout for your application.
Listed below contains GridPane
’s VPos
and HPos
alignments along with their descriptions:
Alignment Method | Description |
---|---|
GridPane.setHalignment(node, HPos value) |
Sets the horizontal alignment of the specified node within its grid cell. Options include HPos.LEFT , HPos.CENTER , or HPos.RIGHT . |
GridPane.setValignment(node, VPos value) |
Sets the vertical alignment of the specified node within its grid cell. Options include VPos.TOP , VPos.CENTER , or VPos.BOTTOM . |
Below is an example JavaFX application that uses GridPane
with setValignment
, setHalignment
, setRowSpan
, and setColumnSpan
. The code includes comments to explain each part:
import javafx.application.Application;
import javafx.geometry.HPos;
import javafx.geometry.Pos;
import javafx.geometry.VPos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class GRIDPANE extends Application {
@Override
public void start(Stage primaryStage) {
// Create a GridPane
GridPane gridPane = new GridPane();
// Set horizontal and vertical gaps between cells
gridPane.setHgap(10);
gridPane.setVgap(10);
// Set grid lines to be visible (for debugging)
gridPane.setStyle("-fx-grid-lines-visible: true;");
// Create labels
Label label1 = new Label("Node 1");
Label label2 = new Label("Node 2");
Label label5 = new Label("Node 5");
Label label3 = new Label("Node 3 (CSpan)");
// Add labels to the GridPane
gridPane.add(label1, 0, 0, 1, 1);
gridPane.add(label2, 1, 0, 1, 1);
gridPane.add(label5, 2, 0, 1, 1);
gridPane.add(label3, 0, 1, 2, 1); // Spanning two columns
// Set alignment for Node 1 (label1)
GridPane.setHalignment(label1, HPos.RIGHT);
GridPane.setValignment(label1, VPos.BOTTOM);
// Set alignment for Node 2 (label2)
GridPane.setHalignment(label2, HPos.CENTER);
GridPane.setValignment(label2, VPos.TOP);
// Set alignment for Node 5 (label5)
GridPane.setHalignment(label5, HPos.CENTER);
GridPane.setValignment(label5, VPos.TOP);
// Set alignment for Node 3 (label3) and span across two columns
GridPane.setHalignment(label3, HPos.CENTER);
GridPane.setValignment(label3, VPos.CENTER);
GridPane.setColumnSpan(label3, 2);
// Add a new label with row span and column span
Label label4 = new Label("Node 4 (CRSpan)");
gridPane.add(label4, 3, 0, 2, 2); // Spanning two column and two row
// Set alignment for Node 4 (label4) and span across two columns and two rows
GridPane.setHalignment(label4, HPos.CENTER);
GridPane.setValignment(label4, VPos.BOTTOM);
GridPane.setColumnSpan(label4, 2);
GridPane.setRowSpan(label4, 2);
// Set the overall alignment of the GridPane
gridPane.setAlignment(Pos.CENTER);
// Create the scene and set it on the stage
Scene scene = new Scene(gridPane, 300, 200);
primaryStage.setScene(scene);
// Set the title of the stage and show it
primaryStage.setTitle("GridPane Alignment Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
The JavaFX code follows a structured process for creating a graphical user interface using a GridPane
. It starts by importing necessary JavaFX classes.
The class GRIDPANE
extends the Application
class, indicating its role as a JavaFX application.
The overridden start
method serves as the entry point for JavaFX applications. Within this method, a GridPane
is created, and the setHgap
and setVgap
methods set horizontal and vertical gaps between cells.
In order to aid in debugging, grid lines are made visible.
Four Label
nodes are then created and added to the grid with specific row and column indices.
Labels are aligned using setHalignment
and setValignment
to control horizontal and vertical alignment. The setColumnSpan
and setRowSpan
methods are employed to make specific labels span multiple columns or rows.
Finally, the overall alignment of the GridPane
is set to the center, and the application window is displayed with the specified title.
When you run this code, you will see a JavaFX window with a grid of labels perfectly aligned based on the specified parameters.
The labels demonstrate the precise control over alignment and spanning capabilities that the GridPane
provides.
Conclusion
Mastering the setValignment
, setHalignment
, setRowSpan
, and setColumnSpan
methods in JavaFX’s GridPane
allows developers to create visually appealing and well-organized user interfaces. These methods are essential tools for achieving responsive layouts in JavaFX applications.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn