How to Fix Java.SQL.SQLException: Access Denied for User Root@Localhost
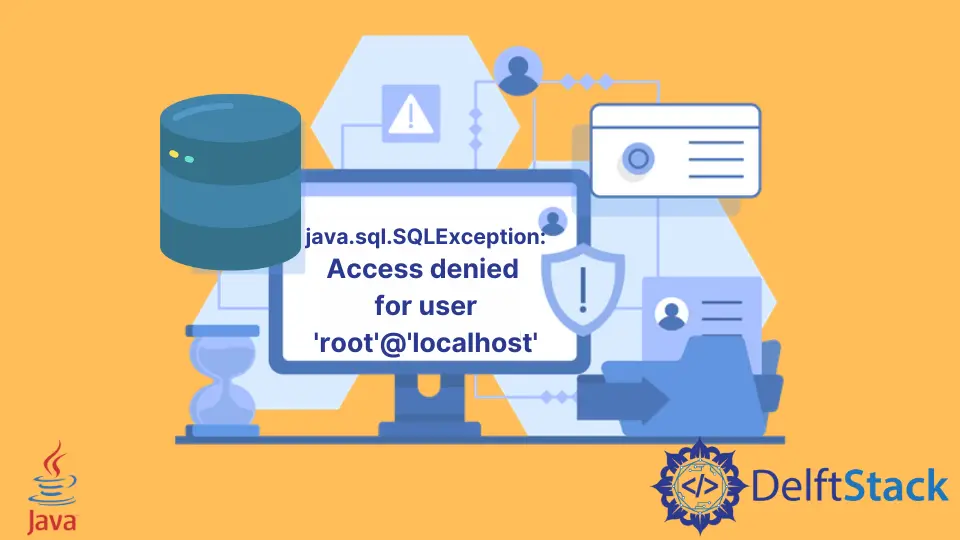
This tutorial demonstrates how to solve the java.sql.SQLException: Access denied for user 'root'@'localhost'
error in Java.
Fix java.sql.SQLException: Access denied for user 'root'@'localhost' (using password: YES)
in Java
This error occurs when we try to uninstall and install a new or previous version of MySQL. The error occurs because when we uninstall MySQL, the MySQL uninstaller wizard
doesn’t perform the complete clean-up.
Manually cleaning the remaining junk will solve this problem. The error which occurs is below.
To solve this error, follow a few simple steps.
-
First, uninstall the MySQL server with
Uninstall Wizard
. -
The installation folder will still be present; delete everything from the folder. For example, the folder is:
C:\Program Files (x86)\MySQL
-
Now, re-install the MySQL Server.
The above process will delete any previously saved passwords and other information causing this error.
There are also some other reasons for this error. For example, creating a new database from scratch using Java and MySQL can throw the same error.
See example:
package delftstack;
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class Example {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
try {
Connection Database_Connection = (Connection) DriverManager.getConnection(
"jdbc:mysql://localhost/?user=root&password=rootpassword");
Statement Demo_Statement = (Statement) Database_Connection.createStatement();
int result = Demo_Statement.executeUpdate("CREATE New Database");
}
catch (Exception e) {
e.printStackTrace();
}
}
}
The code above will throw the same error, and the reason it throws the error is that we are not properly creating the new database. The solution for the above code is given below.
package delftstack;
import com.mysql.jdbc.PreparedStatement;
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class Example {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
try {
Connection Database_Connection =
DriverManager.getConnection("jdbc:mysql://localhost/?user=root&password=rootpassword");
PreparedStatement Demo_Statement =
(PreparedStatement) Database_Connection.prepareStatement("CREATE DATABASE databasename");
int result = Demo_Statement.executeUpdate("CREATE New Database");
}
catch (Exception e) {
e.printStackTrace();
}
}
}
This code will successfully create a new database without any error.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook