How to Fix Java.Security.InvalidKeyException: Illegal Key Size
-
the
java.security.InvalidKeyException: Illegal key size
in Java -
Possible Reasons for
java.security.InvalidKeyException: Illegal key size
-
Eradicate the
java.security.InvalidKeyException: Illegal key size
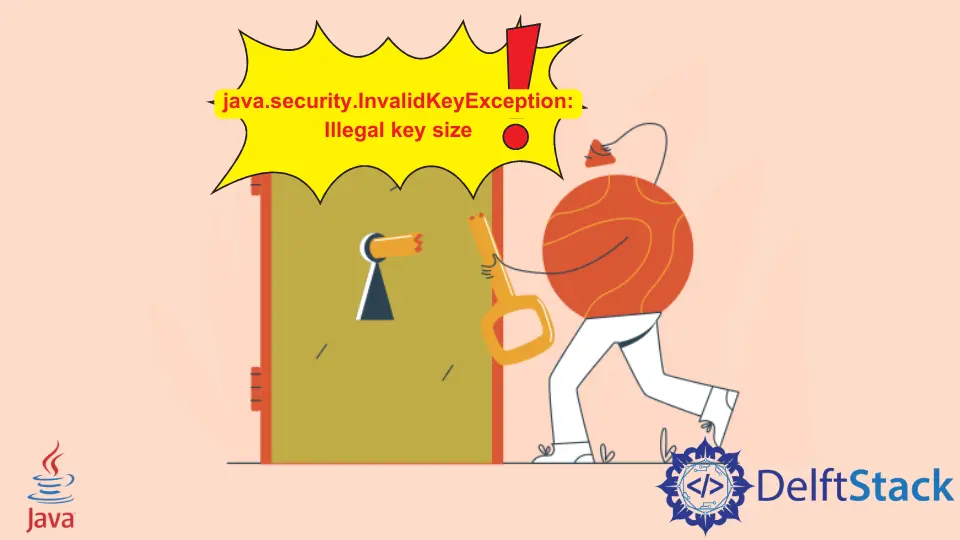
This tutorial presents the Java code that contains the java.security.InvalidKeyException: Illegal key size
. Then, we will learn the possible reasons for it.
Finally, it takes us to the solution by eradicating the specified error.
the java.security.InvalidKeyException: Illegal key size
in Java
Example Code (App1.java
class):
import java.io.UnsupportedEncodingException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.SecretKeySpec;
public class App1 {
private String crypKey = "qkjll5@2md3gs5Q@FDFqf";
public String cryptAString(String str) {
String ret = "";
try {
byte[] crypKeyData = this.crypKey.getBytes();
SecretKeySpec secretKeySpec = new SecretKeySpec(crypKeyData, "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
ret = new String(cipher.doFinal(str.getBytes("UTF-8")));
} catch (NoSuchPaddingException | NoSuchAlgorithmException | InvalidKeyException
| UnsupportedEncodingException | BadPaddingException | IllegalBlockSizeException ex) {
ex.printStackTrace();
}
return ret;
}
}
The crypKey
is as follows:
private String crypKey = "qkjll5@2md3gs5Q@FDFqf";
The function given above accepts a string
type argument. It should encrypt that when we execute this program, but it gives us the java.security.InvalidKeyException: Illegal key size
error.
What does it mean, and what are the reasons? Let’s see in the following section.
Possible Reasons for java.security.InvalidKeyException: Illegal key size
This error means that our Java Virtual Machine (JVM) is using a policy that permits limited cryptography key sizes only due to the US export laws.
Now, the point is, why are we getting this error? Two possibilities are given below.
- First, we are using an algorithm that requires
n-bits
encryption; if we exceed, we will get this error. - We have not yet installed the unlimited strength jurisdiction policy file.
What are these files? These files are Java Cryptography Extensions (JCE
) which enable the Java applications to get the advantage of the powerful version of standard algorithms.
According to the Java documentation, the latest versions of Java Development Kit (JDK
) do not need these kinds of files. They are only required to use an older version of JDK
.
The next question is, which file should we install for what JDK
version? Let’s learn that below.
Java 9 and Higher
As per security updates in the migration guide of Java 9, the unlimited strength jurisdiction policy files are already included in Java 9, and they are used by default.
If we want to execute an application that requires the JCE
unlimited strength jurisdiction policy files with older versions of JDK
, then we do not have to download and install them with Java 9 or later. The reason is that they are already included and used by default.
If we still get this error while working with Java 9, the policy configurations are changed to the more restrictive policy (limited
). But that is not a problem because the limited Java cryptographic policy files are available.
The second reason can be using the wrong algorithm while encrypting the provided string
type argument. Sometimes, the requirements are not satisfied by either policy files provided by default; in that situation, we can customize the policy files to fulfill our needs.
For that, check the security property named crypto.policy
in <java-home>/conf/security/java.security
file, or we can also have a look at the Java Platform, Standard Edition Security Developer’s Guide at Cryptographic Strength Configuration.
Java 8 and Earlier
-
Java 8 Update 161 & Higher - As of Java 8 u161, Java 8 defaults to an unlimited strength jurisdiction policy. If we still get this error, the configuration is changed to
limited
.In the next section, we will see the instructions for changing it to
unlimited
. -
Java 8 Update 151 & Higher - Starting with Java 8 u151, the unlimited strength jurisdiction policy is included in Java 8 but not activated by default.
To activate it, we need to edit our
java.security
file which we can find at<java_home>/jre/lib/security
(forJDK
) or at<java_home>/lib/security
(forJRE
). Uncomment/include the following line:crypto.policy = unlimited
Make sure that we edit this file using an editor running as administrator. Do not forget to restart the Java Virtual Machine because the policy will only take effect after restarting it.
It is particularly necessary for the long-running server processes, for instance, Tomcat. Considering the backward compatibility, following the instructions in the upcoming section to install policy files will also work.
-
Before Java 8 Update 151 - For Java 8 u144 and earlier, we have to install the Java Cryptography Extension (
JCE
) unlimited strength jurisdiction policy files that are available on the official website of Oracle.
Follow the steps given below to install these files:
-
Download the Java Cryptography Extension unlimited strength jurisdiction policy files.
We can go here and here to download Java Cryptography Extension Unlimited Strength Jurisdiction Policy Files for Java 6 and 7, respectively.
-
Un-compress them and extract them to the downloaded file. As a result, a subdirectory named
jce
will be created.This directory will have the following files:
README.txt local_policy.jar US_export_policy.jar
-
Install an unlimited strength policy
JAR
files. Remember, if we want to revert to an originalstrong
butlimited
policy version, then make a copy of the original Java Cryptography Extension policy files (US_export_policy.jar
andlocal_policy.jar
). -
Replace
strong
policy files with an unlimited strength version we extracted in the previous step. The standard locations for Java Cryptography Extension jurisdiction policyJAR
files are as follows:4.1
<java-home>/lib/security
for Unix Operating System.
4.2<java-home>\lib\security
for Windows Operating System.
4.3 ForJDK
, it is in thejre/lib/security
.
Eradicate the java.security.InvalidKeyException: Illegal key size
Example Code (App1.java
class):
import java.io.UnsupportedEncodingException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.SecretKeySpec;
public class App1 {
private String crypKey = "qkjll5@2md3gs5Q@FDFqf";
public String cryptAString(String str) {
String ret = "";
try {
byte[] crypKeyData = this.crypKey.getBytes();
SecretKeySpec secretKeySpec = new SecretKeySpec(crypKeyData, "ARCFOUR");
Cipher cipher = Cipher.getInstance("ARCFOUR");
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
ret = new String(cipher.doFinal(str.getBytes("UTF-8")));
} catch (NoSuchPaddingException | NoSuchAlgorithmException | InvalidKeyException
| UnsupportedEncodingException | BadPaddingException | IllegalBlockSizeException ex) {
ex.printStackTrace();
}
return ret;
}
}
Example Code (Test.java
class):
public class Test {
public static void main(String[] args) {
App1 app = new App1();
String str = "ThisIsFoundation";
String cryptedStr = app.cryptAString(str);
System.out.println(cryptedStr);
System.out.println(cryptedStr.chars().count());
}
}
It will print the encrypted string as ??c[D]???J??n?
and the count of it is 15
characters. How did we solve this?
We can get rid of InvalidKeyException
in two ways.
- We can also solve it by installing Java Cryptography Extension (
JCE
) policy files (all details are given in the previous section). - We can also change the algorithm name.
In our case, the issue is due to the size of crypKey
, which is greater than 16 characters. So, the Cipher
class does not let us encrypt with a key size of more than 128-bits
( where 128 bits == 16 Bytes == 16 Chars
).
So, the value of crypKey
can’t exceed the 16 Chars
limit.
To exceed more than 16 characters, we either install JCE
policy files or update the algorithm name. We will change the algorithm name because we want to keep the built-in key size restriction.
So, we update the algorithm name from AES
to ARCFOUR
. It is because the ARCFOUR
algorithm can be used with various key sizes.