How to Fix Java.Net.SocketException: Broken Pipe Error in Java
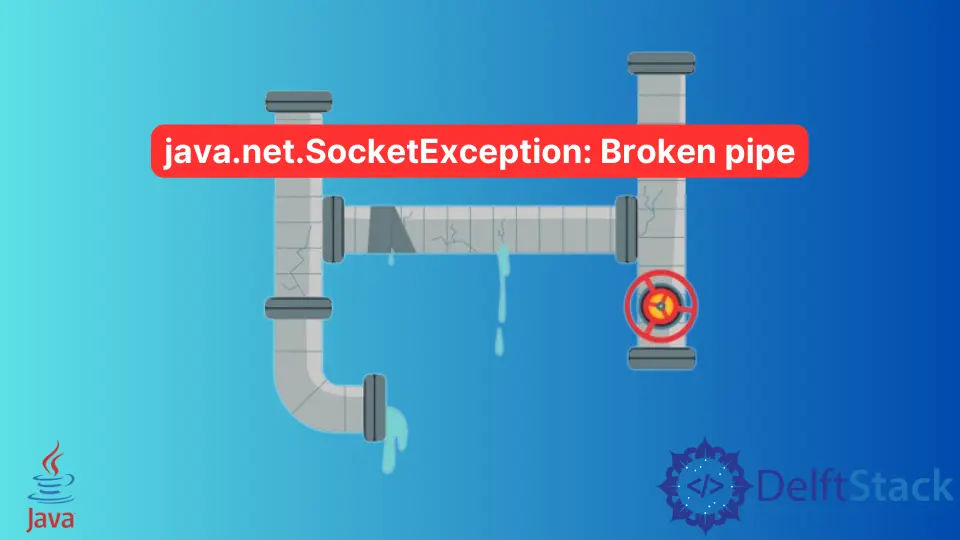
This tutorial demonstrates the java.net.SocketException: Broken pipe
error using Java programming and highlights its possible causes and solutions.
Error’s Description, Reasons and Solutions
It is important to know the error before digging out its reasons and finding solutions. So, let’s start with the error demonstration where we need to send the dynamic buffer size to a socket stream, which works fine.
The error occurs when we try to send more than one buffer with a size that is bigger than the int myBufferSize = 18 * 1024;
(it is an indicative value).
Example Code:
byte[] bs = new byte[myBufferSize];
while (...) {
fileInputStream.read(bs);
byte[] bufferToSend = new byte[sizeBuffer];
DataOutputStream dataOutputStream = new DataOutputStream(client.getoutputStream());
dataOutputStream.writeInt(bufferToSend.length);
dataOutputStream.write(bufferToSend);
dataOutputStream.flush();
}
Error Description:
java.net.SocketException: Broken pipe
at java.net.SocketOutputStream.socketWrite0(Native Method)
What does the broken pipe mean? The broken pipe means that one machine is trying to write/read data to/from a pipe while a machine on the other side of a pipe has been terminated.
Since the connection is terminated, any new connection must be established to transfer the data. Otherwise, data transfer would be ceased.
Remember that Java does not have BrokenPipeException
particularly.
This kind of error would be visibly wrapped in the different exceptions, for instance, IOException
, and SocketException
. In our case, the broken pipe is wrapped in SocketException
.
Why are we getting this problem, and how to resolve this? Some of the common causes are given in the following section.
Reasons for the java.net.SocketException: Broken pipe
Error in Java
We can face this error if any of the following happens.
- This error happens intermittently when multiple clients connect to one server and multiple clients close their connection before the response is fully served/transferred.
- Most of the time, it happens when we write to a connection already closed on the other end.
- It can also occur when a peer closes a connection without reading the whole data, pending at his end. In another case, the peer takes deliberate action to reset instead of properly closing the connection.
- The user closes the browser before a page is loaded fully, which results in unexpected disconnection of the client session for a server.
- Or the users navigate to another page before the current page loaded fully.
- We also get this error when our internet connection fails while loading.
- Another situation for this error is where a browser timed a connection out for a request connection has been finished. Mostly, it occurs when we try to upload large resources.
Possible Solutions to Eradicate the java.net.SocketException: Broken pipe
Error in Java
We can go various ways to eliminate this error while doing client-server programming. Do we want to ignore or handle the root cause to let the developers know?
-
Generally, handling this type of error for server applications is necessary so that our server is not affected by other clients. Yes, as the support person, we can ignore it because the client is already disconnected before the data/response is fully transferred.
-
If the error occurs while uploading huge resources, we must make our API fine with a long request time-out.
-
Sometimes, port scanners do their job by opening a connection and immediately closing it. Our server is not programmed to handle the connection failure.
It is because we didn’t code for this situation. Here, we need to use
try-catch
to handle this situation. -
The broken pipe issue lies on the other side, not in the code. Probably, the other end (it can be the client or server) does not understand our length-word protocol.
For instance, does not implement that correctly. If it is anything similar to this code (provided in the error's demonstration), it won't.
We ignore the response returned by the `read()`. Further, assume that it fills the buffer; it is not specified to do that, only to send/transfer one byte.
-
Another solution is to check the code where the Input/Output exception occurs and wrap that with a
try-catch
block to catch theIOException
. Then, it is up to us to decide how we handle this semi-valid scenario.Remember, this case only applies if the broken pipe is wrapped around the
IOException
.