How to Fix Java.Lang.ClassNotFoundeException: Sun.Jdbc.Odbc.JdbcOdbcDriver
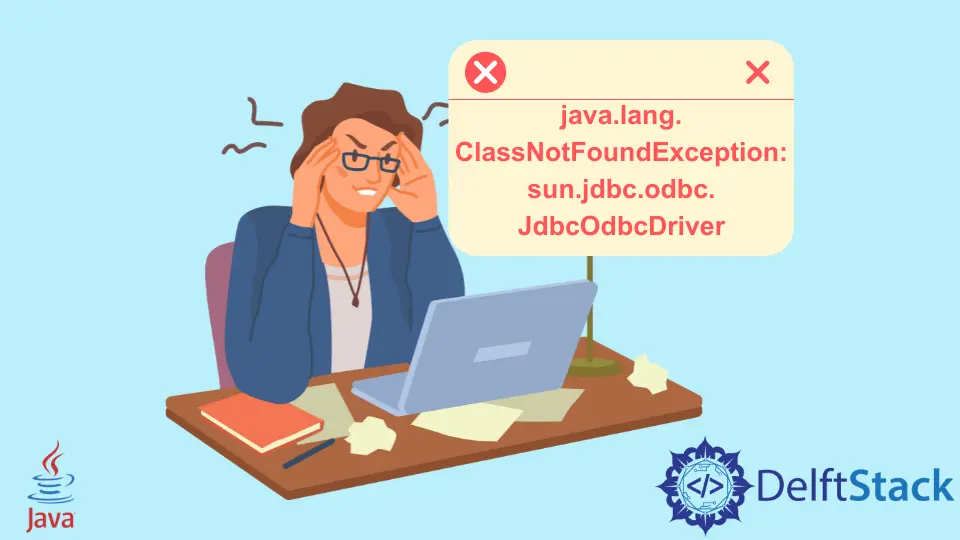
This tutorial demonstrates the java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
error in Java.
Fix java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
in Java
When we use the MS Access database with Java, the error java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
can occur. This error is because Java 8 or JDK 1.8 doesn’t support the JDBC ODBC bridge.
So if you are using Java 8 or JDK 1.8 or the versions after them, the chances are the error java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
will occur.
There are two solutions to solve this error, one is to revert Java to 7 or JDK before 1.8, and the second solution is to use the UCanAccess
jar
s. Both solutions are described below.
Revert to Java or JDK Before the 8 and 1.8 Versions
To use the JDBC ODBC bridge, you must have Java 7 or previous versions because these drivers are only supported. Follow the steps below to revert to previous versions of Java or JDK.
Using Eclipse:
-
Right-click on the project and go to
Properties
. -
Go to
Java Compiler
. -
Check the
Enable project specific settings
box. -
Now select a version of JDK before 1.8 from
Compiler compliance level
. -
Click
Apply and Close
. The JDK is reverted to the selected version.
Using Windows or CMD:
-
You must remove the previous Java version and install the reverted one.
-
Go to the
Control Panel
and add or remove programs. -
Remove Java.
-
Download the Java 7 or previous from here.
-
Install by following the installation process.
After reverting to previous versions of Java, the error java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
will be solved.
Use UCanAccess
Jars
The UCanAccess
jars are used to make the connection between Java and the Access database. Follow the process below to add UCanAccess
jars to solve the java.lang.ClassNotFoundException: sun.jdbc.odbc.JdbcOdbcDriver
error.
-
Download the
UCanAccess
jars from here. -
Add the
jar
to your build path. -
In the case of Maven, the following dependency will be added to the
pom.xml
file.
<dependency>
<groupId>net.sf.ucanaccess</groupId>
<artifactId>ucanaccess</artifactId>
<version>5.0.0</version>
</dependency>
Once the UCanAccess
jar
is added to the build path and Maven dependency is set, you can connect to the MS Access database; see the example.
package delftstack;
import java.sql.Connection;
import java.sql.DriverManager;
public class Example {
public static void main(String args[]) {
String Database_URL = "jdbc:ucanaccess://C:\\Users\\Sheeraz\\Databases\\Demo.accdb";
try {
// making connection
Connection Database_Connection = DriverManager.getConnection(Database_URL);
if (Database_Connection != null) {
System.out.println("The Database is Connected successfully.");
Database_Connection.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
The code above will work properly and connect to the MS Access database named Demo.accdb
. See output:
The Database is Connected successfully.
Another thing should be mentioned here; if the database is large, we must also mention the Xmx
and Xms
parameters or set the memory parameter to false
; otherwise, it will throw the error. Set the memory parameter to false
, as shown below.
String Database_URL = "jdbc:ucanaccess://C:\\Users\\Sheeraz\\Databases\\Demo.accdb";memory=false";
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook