How to Fix Java.IO.StreamCorruptedException: Invalid Stream Header
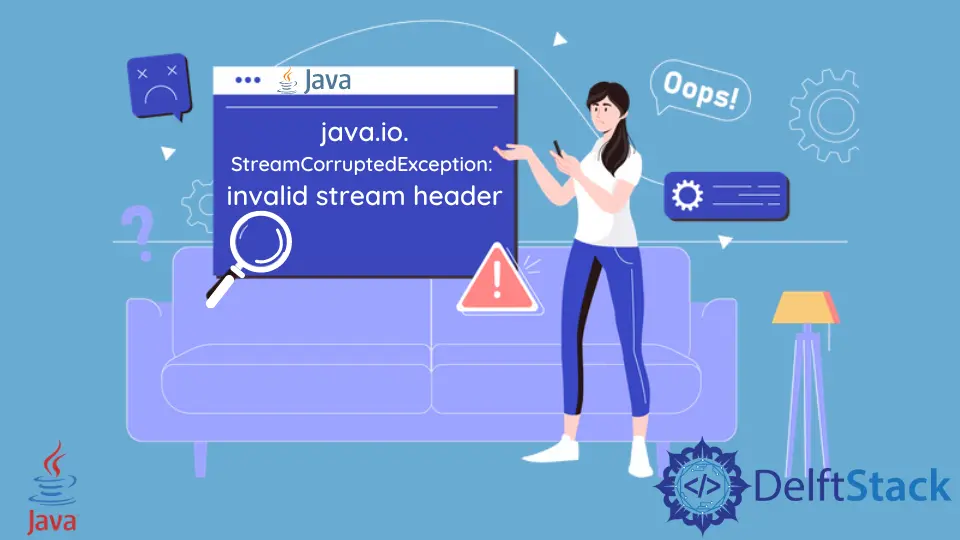
This tutorial demonstrates how to fix Java’s java.io.StreamCorruptedException: invalid stream header
exception.
java.io.StreamCorruptedException: invalid stream header
in Java
The java.io.StreamCorruptedException: invalid stream header
is relatively a common exception. To understand the reason for this exception, we need to see the invalid stream header in the exception because it can explain the reason behind the issue.
The StreamCorruptedException
has two constructors, the first with one argument and the other without arguments. The one who accepts the arguments describes the reasons for the exception.
The java.io.StreamCorruptedException: invalid stream header: XXXXXX
is the exception with the argument where XXXXXX
represents the invalid header details.
This exception commonly occurs when we are working with ObjectInputStream
. Because, as per the Javadoc
, only those objects can be read from streams that support the java.io.Serializable
or java.io.Externalizable
.
So whenever we use the ObjectInputStream
, it should be used with the serialized data. Most of the StreamCorruptedException: invalid stream header
occurs when a file such as text, HTML, XML, JSON, etc., is directly passed to the ObjectInputStream
constructor rather than a serializable file.
Here are some commonly occurred java.io.StreamCorruptedException: invalid stream header
with corresponding ASCII values.
HEX Value for Invalid Stream Header | Integers for HEX | ASCII Values for HEX |
---|---|---|
54657374 | 084 101 115 116 | Test |
48656C6C | 072 101 108 108 | Hell |
54656D70 | 084 101 109 112 | Temp |
4920616D | 073 032 097 109 | I am |
54686973 | 084 104 105 115 | This |
20646520 | 032 100 101 032 | de |
30313031 | 048 049 048 049 | 0101 |
32303138 | 050 048 049 056 | 2018 |
41434544 | 065 067 069 068 | ACED |
64617364 | 100 097 115 100 | dasd |
72657175 | 114 101 113 117 | requ |
7371007E | 115 113 000 126 | sq ~ |
70707070 | 112 112 112 112 | pppp |
77617161 | 119 097 113 097 | waqa |
7B227061 | 123 034 112 097 | {"pa |
3C21444F | 060 033 068 079 | <!DO |
3C787364 | 060 120 115 100 | <xsd |
0A0A3C68 | 010 010 060 104 | <h |
3c48544d | 060 072 084 077 | <HTM |
3C6F626A | 060 111 098 106 | <obj |
00000000 | 000 000 000 000 | |
0A0A0A0A | 010 010 010 010 |
Let’s try an example that will throw the java.io.StreamCorruptedException: invalid stream header
exception. See example:
package delftstack;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.lang.ClassNotFoundException;
import java.net.ServerSocket;
import java.net.Socket;
public class Example {
private static ServerSocket Server_Socket;
private static int Port_Number = 5000;
public static void main(String args[]) throws IOException, ClassNotFoundException {
Server_Socket = new ServerSocket(Port_Number);
while (true) {
System.out.println("Waiting for client request");
// create socket and then wait for client connection
Socket DemoSocket = Server_Socket.accept();
// read from socket to the ObjectInputStream object
ObjectInputStream Object_Input_Stream = new ObjectInputStream(DemoSocket.getInputStream());
// convert the ObjectInputStream to the String
String DemoMessage = (String) Object_Input_Stream.readObject();
System.out.println("The Received Message is: " + DemoMessage);
// ObjectOutputStream object
ObjectOutputStream Object_Output_Stream =
new ObjectOutputStream(DemoSocket.getOutputStream());
// write object to the Socket
Object_Output_Stream.writeObject("Hello Client " + DemoMessage);
// close
Object_Input_Stream.close();
Object_Output_Stream.close();
DemoSocket.close();
// terminate the server on exit from the client
if (DemoMessage.equalsIgnoreCase("exit"))
break;
}
System.out.println("Shut down Socket server!!");
// close the ServerSocket
Server_Socket.close();
}
}
The code above is trying to read a string sent from the client. The output for this code is the java.io.StreamCorruptedException: invalid stream header
exception.
See output:
Exception in thread "main" java.io.StreamCorruptedException: invalid stream header: 54657374
at java.io.ObjectInputStream.readStreamHeader(ObjectInputStream.java:803)
at java.io.ObjectInputStream.<init>(ObjectInputStream.java:298)
at Example.main(SocketServerExample.java:29)
The reason for this exception is we are not sending the data using the ObjectOutputStream
because we cannot expect the ObjectOutputStream
to convert text to objects automatically; the hexadecimal value 54657374
is converted to Test
as text, and we must be sending it directly as bytes.
We can use the methods readObject()
and writeObject()
to solve this problem.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook