How to Get Word Count of a String in Java
-
Use
StringTokenizer
to Count Words in a String in Java -
Use
split()
and Regular Expression to Count Words in a String in Java - Get Number of Times a Word Is Repeated in a String in Java
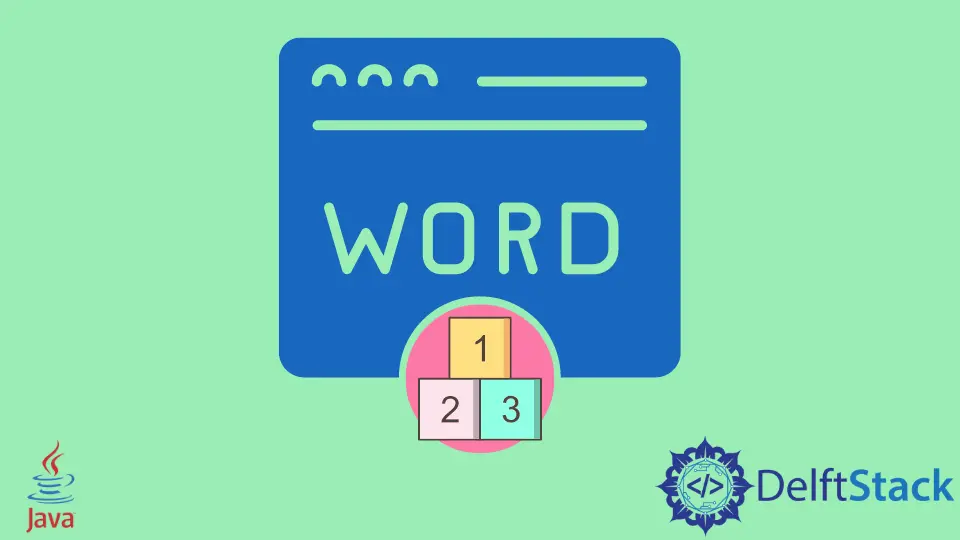
In this article, we are going to shed some light on how to count the number of words in a string in Java and different ways to achieve this.
Use StringTokenizer
to Count Words in a String in Java
The StringTokenizer
class in Java allows us to break a string into multiple tokens. The methods in this class do not differentiate among quoted strings, identifiers, and numbers, nor do they recognize or skip comments. The characters that separate tokens (delimiters) may be specified either at the time of creation or on a per-token basis.
The StringTokenizer
class does not count the whitespace and tabs automatically hence it is handled on its own.
import java.util.StringTokenizer;
public class WordCount {
public static void main(String args[]) {
String mydelim = " - ";
String myString = "The sky - - is blue!";
StringTokenizer stringTokenizer1 = new StringTokenizer(myString);
StringTokenizer stringTokenizer2 = new StringTokenizer(myString, mydelim);
int tokensCount1 = stringTokenizer1.countTokens();
int tokensCount2 = stringTokenizer2.countTokens();
System.out.println("Word count without delimiter: " + String.valueOf(tokensCount1));
System.out.println("Word count: " + String.valueOf(tokensCount2));
}
}
Output:
Word count without delimiter: 6
Word count: 4
Here, we have created stringTokenizer1
with passing a string in the constructor, it takes default delimiter whereas for stringTokenizer2
custom delimiter to separate tokens is passed. The first approach does count hyphens while the second doesn’t.
Use split()
and Regular Expression to Count Words in a String in Java
The split(String regex)
method in Java takes a regular expression and breaks the given string matching the regex and returns an array of strings.
The regular expression we use here is \\s+
which separates the whitespace from the string or in other words it splits the given string around whitespace. The number of words in the string is equal to the length of string array words
which we can get by calling length
on it. The output shows the number of words in myStr
.
public class WordCount {
public static void main(String args[]) {
String myStr = "the butcher's wife, she was from Montreal";
String[] words = myStr.split("\\s+");
System.out.println("Word Count is: " + words.length);
}
}
Output:
Word Count is: 7
Get Number of Times a Word Is Repeated in a String in Java
We can calculate the number of times a word is repeated in the given string. Here randomText
is a string in which we need to find how many times the word blue
is repeated. For this, we take a variable times
of type int
and initialize it to 0. Run a for
loop from 0 to the length of the randomText
.
The startsWith()
method compares if the string starts with the characters of the given string. For i = 0
the randomText.substring(0)
method gets substring at the index 0 and checks if it startsWith("blue")
and if it matches then it will increment times
by 1; otherwise, it will not increase.
Similarly, it checks for the rest of the length and prints the number of times the word was repeated in the given string as output.
public class WordCount {
public static void main (String args[]){
String randomText = "The sky is blue it meets the sea which is also blue";
String text = "blue";
int times = 0;
for (int i = 0; i < randomText.length(); i++) {
if (randomText.substring(i).startsWith(text)) {
times ++;
}
}
System.out.println(randomText + " contains " + text + " " + times + " times");
}
}
Output:
The sky is blue it meets the sea which is also blue contains blue 2 times
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn