How to Fix java.util.date to java.sql.date in Java
-
Why Convert
java.util.Date
tosql.Date
? - Introduction to Java Date Conversion
-
Standard Method for Converting
java.util.Date
tojava.sql.Date
- Alternative Conversion Methods
- Best practices for Date Conversion in Java
- Common Pitfalls and How to Avoid Them
- Conclusion
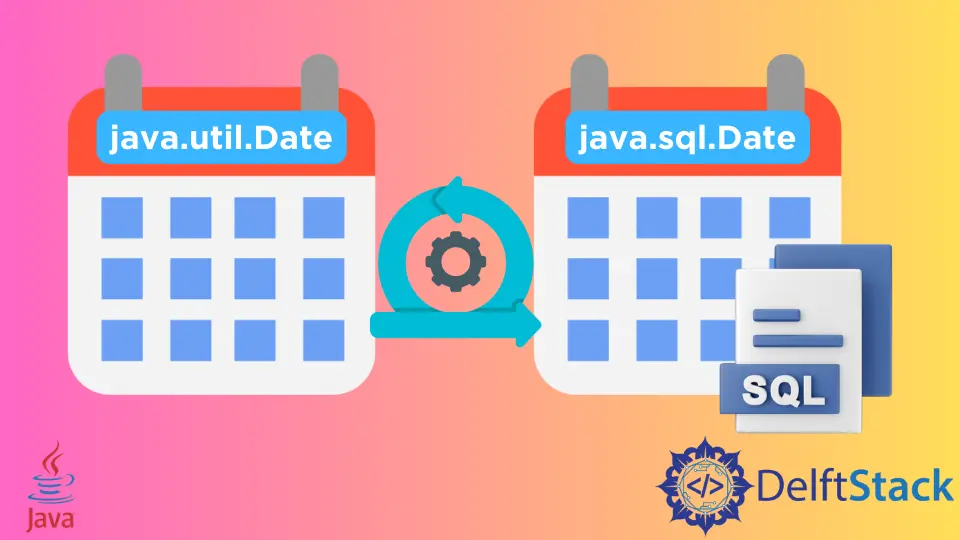
This article navigates through the standard approaches of converting java.util.Date
to java.sql.Date
, such as getTime()
and SimpleDateFormat
with valueOf()
, ensuring precision in date conversion. Uncover alternative methods like java.sql.Timestamp
and the java.time
package, providing newer perspectives.
Discover best practices for seamless conversions and gain insights into common pitfalls, along with strategies to evade them. Whether you’re a Java enthusiast or a seasoned developer, this concise guide equips you with essential tools to handle date-related operations effectively.
Why Convert java.util.Date
to sql.Date
?
In java program to convert java.util.Date
to java.sql.Date
holds significance, especially in database interactions.
While java.util.Date
encapsulates both date and time, databases often mandate precision in date values, excluding the time aspect. This precision aligns with SQL standards and necessitates the conversion to java.sql.Date
.
Tailored for database storage, java.sql.Date
discards time-related details, meeting SQL expectations. This conversion ensures compatibility between Java applications and SQL databases, enabling accurate handling of date values without incorporating unnecessary time complexities.
In essence, it streamlines the communication of date information, adhering to SQL conventions and optimizing the interaction between Java and databases.
Introduction to Java Date Conversion
Understanding java.util.Date
In Java programming, the java.util.Date
class is a fundamental component for handling date and time information. It belongs to the java.util
package and serves as a versatile representation of both date and time.
This date class is crucial for various time-related operations within Java applications. It allows developers to work with specific dates, manipulate time-related aspects, and perform essential calculations.
The Java util date
class has been an integral part of Java since its early versions and continues to play a central role in managing temporal data within Java applications.
Understanding java.sql.Date
In Java, the java.sql.Date
class is a specialized implementation within the java.sql
package, designed specifically for handling date information. Unlike its counterpart, java.sql.Date
focuses solely on representing date values, excluding any associated time details, making it particularly suitable for scenarios where precision to only a date component is required.
Commonly used in Java applications that interact with databases, java.sql.Date
ensures seamless integration with SQL date requirements, allowing developers to store and retrieve accurate SQL date value.
Developers often use this date class to convert Java util date
object to a format compatible with SQL databases, offering a streamlined approach to handle date-related operations within Java applications and facilitating efficient calendar management. The use of java.sql.Date
is particularly beneficial when dealing with scenarios involving sql date system, date java, and date data type in SQL server environments.
Standard Method for Converting java.util.Date
to java.sql.Date
Using getTime()
Method
Using getTime()
in converting Java util date
to SQL date is crucial for precision and simplicity. It directly retrieve the millisecond values, ensuring accurate representation and seamless conversion.
This method is efficient, providing a direct and reliable way to handle date-related operations, making it a preferred choice over alternative approaches.
Code Example:
import java.util.Date;
public class DateConverter {
public static java.sql.Date convertUtilDateToSqlDate(Date utilDate) {
// Use getTime() to get the millisecond value from java.util.Date
long millis = utilDate.getTime();
// Convert the millisecond value to java.sql.Date
return new java.sql.Date(millis);
}
public static void main(String[] args) {
// Example usage
Date utilDate = new Date();
java.sql.Date sqlDate = convertUtilDateToSqlDate(utilDate);
// Display the converted date
System.out.println("Converted SQL Date: " + sqlDate);
}
}
The conversion involves two main steps. First, the getTime()
method retrieves the milliseconds since the epoch from the given java.util.Date
(utilDate
). The value of millisecond is stored in millis
. Next, this value creates a new java.sql.Date
, discarding the time component and preserving only the date information.
In the main
method, we demonstrate the conversion by creating a new util date
java, using getTime()
, and displaying the resulting java.sql.Date
. The output shows a successful conversion based on the current time and date.
When you run this program, the output will display the java program to convert into SQL Date is displayed.
Using getTime()
provides a simple and precise method for converting java.util.Date
to java.sql.Date
, especially when only the date information is needed. It’s an effective technique for handling date conversions in Java applications, ensuring accuracy up to milliseconds.
Using SimpleDateFormat
and valueOf()
Method
Utilizing SimpleDateFormat
and valueOf()
to convert java.util.Date
to java.sql.Date
provides clarity and ease.
SimpleDateFormat
defines the date format, ensuring accurate parsing. valueOf()
then directly converts the formatted date string to a java.sql.Date
, streamlining the process with simplicity and readability.
This approach is user-friendly and maintains precision in date handling, making it a favorable choice over other complex methods.
Code Example:
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConverter {
public static java.sql.Date convertUtilDateToSqlDate(Date utilDate) {
// Define the date format
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
// Format java.util.Date using SimpleDateFormat
String formattedDate = dateFormat.format(utilDate);
// Convert formatted date to java.sql.Date using valueOf()
return java.sql.Date.valueOf(formattedDate);
}
public static void main(String[] args) {
// Example usage
Date utilDate = new Date();
java.sql.Date sqlDate = convertUtilDateToSqlDate(utilDate);
// Display the converted date
System.out.println("Converted SQL Date: " + sqlDate);
}
}
To convert a java.util.Date
to a java.sql.Date
in Java, using SimpleDateFormat
and valueOf()
, follow these steps.
Firstly, define the desired date format with SimpleDateFormat
, like SimpleDateFormat dateFormat = new SimpleDateFormat(yyyy-MM-dd)
. Next, apply this format to the original java.util.Date
using dateFormat.format(utilDate)
, resulting in a formatted date string stored in formattedDate
.
Finally, convert this formatted string to a java sql date with java.sql.Date.valueOf(formattedDate)
. This method retains only the date information, excluding time. This process is handy for customizing date formats when interacting with databases.
The main
method exemplifies this, generating a new java util date, applying the conversion, and printing the resulting java.sql.Date
.
When you run this program, the output will display the converted SQL Date.
The format of the output will be based on the current date and time java when the program is executed, following the yyyy-MM-dd
format specified in the SimpleDateFormat
. This approach provides a clear and concise method for date conversions in Java, leveraging the simplicity and flexibility of SimpleDateFormat
and valueOf()
.
Alternative Conversion Methods
Using java.sql.Timestamp
Leveraging java.sql.Timestamp
in converting java.util.Date
to java.sql.Date
offers finer time precision. This subclass of java.util.Date
directly captures millisecond values, ensuring accurate representation.
Using Timestamp
simplifies the conversion process, making it a preferred choice for scenarios requiring detailed time information. This approach is efficient and straightforward, enhancing the precision of date-related operations in Java programming.
Code Example:
import java.sql.Timestamp;
import java.util.Date;
public class DateConverter {
public static java.sql.Date convertUtilDateToSqlDate(Date utilDate) {
// Convert java.util.Date to java.sql.Timestamp
Timestamp timestamp = new Timestamp(utilDate.getTime());
// Convert java.sql.Timestamp to java.sql.Date
return new java.sql.Date(timestamp.getTime());
}
public static void main(String[] args) {
// Example usage
Date utilDate = new Date();
java.sql.Date sqlDate = convertUtilDateToSqlDate(utilDate);
// Display the converted date
System.out.println("Converted SQL Date: " + sqlDate);
}
}
In the conversion process, we first convert a java.util.Date
to a java.sql.Timestamp
using Timestamp timestamp = new Timestamp(utilDate.getTime())
. This creates a Timestamp
object with finer-grained time precision.
Subsequently, we convert the obtained java.sql.Timestamp
to a java.sql.Date
with new java.sql.Date(timestamp.getTime())
, extracting only the date portion. The main
method illustrates this conversion by creating a new java.util.Date
, applying the conversion, and printing the resulting java.sql.Date
.
This concise demonstration emphasizes the straightforward application of the conversion logic, showcasing a seamless way to move from java.util.Date
to java.sql.Date
through an intermediary java.sql.Timestamp
.
When you run this Java program, it will display the converted SQL Date, showcasing the successful conversion of a java.util.Date
to a java.sql.Date
using the java.sql.Timestamp
method. The output will be based on the current time and date.
This approach not only simplifies the code but also provides flexibility and precision when handling date and time conversion in Java application. It leverages the java.sql.Timestamp
class as a thin wrapper around the value of millisecond, making it an effective tool for date-related operations, especially in database interactions.
Leveraging java.time
Package
The java.time
package in Java offers a modern and efficient approach to convert java.util.Date
to java.sql.Date
. It provides classes like LocalDate
for seamless date manipulation, promoting clearer and more concise code.
Unlike traditional methods, it ensures better handling of time zones and supports finer time precision. Utilizing the java.time
package enhances readability, simplifies date-related operations, and aligns with current best practices in Java programming.
Code Example:
import java.sql.Date;
import java.time.LocalDate;
import java.time.ZoneId;
import java.util.Date;
public class DateConverter {
public static Date convertUtilDateToSqlDate(Date utilDate) {
// Convert java.util.Date to java.time.LocalDate
LocalDate localDate = utilDate.toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
// Convert java.time.LocalDate to java.sql.Date
return java.sql.Date.valueOf(localDate);
}
public static void main(String[] args) {
// Example usage
Date utilDate = new Date();
Date sqlDate = convertUtilDateToSqlDate(utilDate);
// Display the converted date
System.out.println("Converted SQL Date: " + sqlDate);
}
}
Demonstrating the conversion process in the main
method, we initially convert a java.util.Date
to a java.time.LocalDate
. This includes using the toInstant()
method to transform the java.util.Date
into a specific Instant
object, representing a moment in time.
Next, with atZone(ZoneId.systemDefault())
, we associate this Instant
with the system’s default time zone, creating a ZonedDateTime
object. Finally, by calling toLocalDate()
, we extract the date part, resulting in a LocalDate
object.
Afterward, the java.time.LocalDate
is converted to a java.sql.Date
using the java.sql.Date.valueOf(localDate)
method, which performs a standard conversion.
The provided main
method exemplifies the practical application of this standard conversion, emphasizing its simplicity and effectiveness.
When you run this Java program, it will display the converted SQL Date, demonstrating the successful conversion of a java.util.Date
to a java.sql.Date
. The output will be based on the current date and the system’s default time zone.
This approach not only simplifies the code but also ensures compatibility with modern date and time handling in Java, making it a recommended method for date conversions in contemporary Java applications.
Best practices for Date Conversion in Java
Understanding Date Classes in Java
When working with dates in Java, it’s essential to be familiar with the java.util.Date
and Java SQL date classes. While java.util.Date
represents both date and time, java.sql.Date
focuses solely on the value of date, making it suitable for SQL database interactions.
Choosing the Right Date Type
Select the appropriate date type based on your needs. If your application deals primarily with date information and requires compatibility with SQL databases, prefer using java.sql.Date
to store only the date component.
Utilizing Time-Related Methods
Leverage time-related methods available in the java.util.Date
class when dealing with both date and time information. This instant class provides versatile methods for manipulating temporal data.
Using SimpleDateFormat
for Custom Formatting
For custom date formatting, use SimpleDateFormat
in the java.text
package. This is particularly useful when displaying dates in a specific format or parsing date strings.
Converting java.util.Date
to java.sql.Date
When interfacing with databases, convert java.util.Date
to java.sql.Date
using the java.sql.Date.valueOf()
method. This ensures compatibility with SQL date requirements.
Handling Time Zones Appropriately
Be mindful of time zones, especially in distributed systems or applications serving users in different regions. Consider using the TimeZone
class to handle particular time zone-specific requirements.
Maintaining Consistency in Code
Maintain consistency in date representation throughout your codebase. Choose a standard format and adhere to it to avoid confusion and potential issues in date-related operations.
Ensuring Millisecond Precision
When working with date and time information, be aware of the millisecond precision offered by java.util.Date
. If precision is crucial, this information may be significant for certain operations.
Understanding JDBC Drivers
When dealing with databases, understand the JDBC drivers being used. Different databases may have specific requirements or nuances regarding date handling.
Common Pitfalls and How to Avoid Them
Misuse of Deprecated Classes
Avoid using deprecated classes like java.util.Date
and favor the newer java.time
classes introduced in Java 8. The old classes are error-prone and lack the functionality and precision provided by the new classes.
Confusion Between java.util.Date
and java.sql.Date
Be cautious when dealing with both java.util.Date
and java.sql.Date
. Understand the differences and use the appropriate class based on your requirements. Misusing them can lead to unexpected results, especially in database interactions.
Inadequate Handling of Time Zones
Always consider time zones when working with dates. Neglecting time zone considerations can result in inaccurate date calculations, especially in applications serving users in different regions. Use ZoneId
and ZonedDateTime
when necessary.
String to Date Conversion Without Format
When converting strings to dates, always provide a format to avoid parsing issues. Incorrect or missing formats can lead to parsing errors, making it crucial to specify the expected date string format using SimpleDateFormat
or DateTimeFormatter
.
Using Milliseconds for Time Calculations
While java.util.Date
provides precision in milliseconds, be cautious when using it for time-related calculations. Consider using the more precise classes in java.time
for accurate and reliable time manipulations.
Not Considering Database-Specific Date Formats
When interacting with databases, be aware of the date formats they expect. Different databases may have specific requirements, and failing to adhere to these formats can result in errors. Use java.sql.Date
appropriately for seamless integration.
Neglecting JDBC Driver Compatibility
Ensure compatibility between your Java application and the JDBC driver used for database connectivity. Incompatibility issues may arise if the versions or configurations are mismatched.
Ignoring the Need for Error Handling
When converting or manipulating dates, incorporate error-handling mechanisms. Failure to do so can result in unexpected runtime exceptions. Handle exceptions gracefully to enhance the robustness of your application.
Overlooking Leap Year Considerations
When dealing with specific dates or calculations involving years, be mindful of leap years. Ignoring leap year considerations can lead to inaccuracies in date-related operations.
Using Deprecated SQL Date Constructors
When creating java.sql.Date
instances, avoid using deprecated constructors. Instead, prefer using the java.sql.Date.valueOf(LocalDate)
constructor for improved readability and adherence to modern practices.
Conclusion
Converting java.util.Date
to java.sql.Date
in Java is a critical operation addressed through standard methods like getTime()
and SimpleDateFormat
with valueOf()
. Additionally, alternative approaches, such as using java.sql.Timestamp
and the java.time
package, were explored.
The article delved into best practices for date conversion, emphasizing precision and simplicity. Common pitfalls were also highlighted, providing insights on how to avoid errors.
Whether utilizing the established methods in the java util package and java sql
package or exploring newer options in the java.time
package, understanding the nuances ensures effective handling of date and time in Java programming.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn