How to Create Unsigned Int in Java
- Understanding Unsigned Integers
- Method 1: Using Long to Represent Unsigned Int
- Method 2: Bit Manipulation Techniques
- Method 3: Custom Unsigned Integer Class
- Conclusion
- FAQ
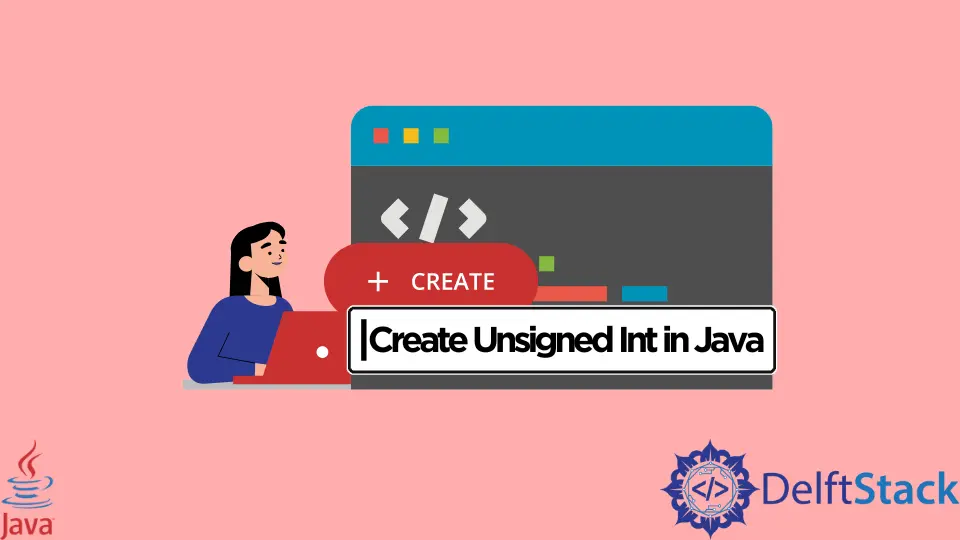
Creating an unsigned integer in Java can be a bit of a challenge, especially since Java doesn’t have a built-in unsigned integer type. However, there are ways to work around this limitation using existing data types and techniques.
In this article, we will explore how to create unsigned integers in Java, focusing on practical methods that can be applied in real-world scenarios. Whether you are working with large numbers or need to ensure that integers remain non-negative, this guide will provide you with the knowledge you need. Let’s dive in and uncover the various approaches to handle unsigned integers in Java.
Understanding Unsigned Integers
Before we jump into the solutions, let’s clarify what unsigned integers are. Unlike signed integers, which can represent both positive and negative values, unsigned integers can only represent non-negative values. In Java, the primitive types such as int
, long
, short
, and byte
are signed, meaning they can hold negative values.
To work with unsigned integers in Java, we often use larger signed types or manipulate the values to ensure they stay within the desired range. For example, using a long
to represent an unsigned integer allows us to handle larger values without running into the negative range.
Method 1: Using Long to Represent Unsigned Int
One of the simplest methods to create an unsigned integer in Java is by using the long
data type. This approach allows you to store larger values that would exceed the maximum limit of a signed integer while ensuring you only work with non-negative values.
Here’s how you can implement this:
public class UnsignedIntExample {
public static void main(String[] args) {
long unsignedInt = 4294967295L; // Maximum value for unsigned int
System.out.println("Unsigned Int: " + unsignedInt);
}
}
Output:
Unsigned Int: 4294967295
In this code snippet, we declare a variable of type long
to hold the maximum unsigned integer value, which is 4294967295. By using long
, we effectively bypass the limitations of the int
type. This method is particularly useful when you need to perform calculations or store values that might exceed the standard integer range. However, it’s essential to remember that while long
can hold larger values, it’s still crucial to ensure that the values you assign are within the unsigned range to avoid unexpected results.
Method 2: Bit Manipulation Techniques
Another effective method to create and handle unsigned integers in Java is through bit manipulation techniques. This approach allows you to work with signed integers while treating them as unsigned. By using bitwise operations, you can manipulate the bits directly to achieve the desired results.
Here’s an example of how to use bit manipulation to create an unsigned integer:
public class UnsignedIntBitManipulation {
public static void main(String[] args) {
int signedInt = -1; // Example signed integer
long unsignedInt = signedInt & 0xFFFFFFFFL; // Convert to unsigned
System.out.println("Unsigned Int: " + unsignedInt);
}
}
Output:
Unsigned Int: 4294967295
In this example, we start with a signed integer -1
, which, when treated as an unsigned integer, represents the maximum unsigned value. By performing a bitwise AND operation with 0xFFFFFFFFL
, we effectively convert the signed integer to its unsigned equivalent. This method is powerful because it allows you to manipulate integers at the bit level, making it suitable for applications that require precise control over data representation.
Method 3: Custom Unsigned Integer Class
If you find yourself working extensively with unsigned integers, creating a custom class might be the best solution. This approach allows you to encapsulate the behavior and properties of an unsigned integer, providing a clean interface for users of your class.
Here’s how you can create a simple custom unsigned integer class in Java:
public class UnsignedInt {
private long value;
public UnsignedInt(long value) {
if (value < 0 || value > 4294967295L) {
throw new IllegalArgumentException("Value must be between 0 and 4294967295");
}
this.value = value;
}
public long getValue() {
return value;
}
@Override
public String toString() {
return Long.toString(value);
}
public static void main(String[] args) {
UnsignedInt unsignedInt = new UnsignedInt(3000000000L);
System.out.println("Unsigned Int: " + unsignedInt);
}
}
Output:
Unsigned Int: 3000000000
In this code, we define a class UnsignedInt
that encapsulates a long
value. The constructor checks if the provided value is within the valid range for an unsigned integer. The getValue()
method allows you to retrieve the stored value, while the toString()
method provides a string representation of the object. This custom class approach not only makes your code cleaner but also adds a layer of abstraction, making it easier to manage unsigned integers throughout your application.
Conclusion
Creating unsigned integers in Java may not be straightforward due to the absence of a native unsigned type. However, by using long
, bit manipulation, or even a custom class, you can effectively manage unsigned integers in your Java applications. Each method has its advantages, and the choice depends on your specific use case. By understanding these techniques, you can ensure that your applications handle non-negative integers correctly and efficiently.
FAQ
-
What is an unsigned integer?
An unsigned integer is a data type that can represent only non-negative values, unlike signed integers, which can represent both positive and negative values. -
Why doesn’t Java have an unsigned int type?
Java was designed with simplicity and safety in mind, focusing on signed integers to avoid complications associated with negative values. -
Can I use a byte or short as an unsigned integer in Java?
While you can usebyte
orshort
, they are still signed types. You would need to use bit manipulation to treat them as unsigned. -
What is the maximum value of an unsigned integer in Java?
The maximum value for an unsigned integer in Java is 4294967295, which corresponds to the maximum value of a 32-bit unsigned integer. -
How do I handle arithmetic operations on unsigned integers in Java?
You can perform arithmetic operations usinglong
to avoid overflow issues. Just ensure that the results remain within the unsigned range.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn