How to Truncate Double in Java
-
Use
DecimalFormat
to Truncate Double in Java -
Use
BigDecimal
to Truncate Double in Java - Use Apache Common Library to Truncate Decimal in Java
-
Use
java.lang.Math
to Truncate Decimal in Java - Conclusion
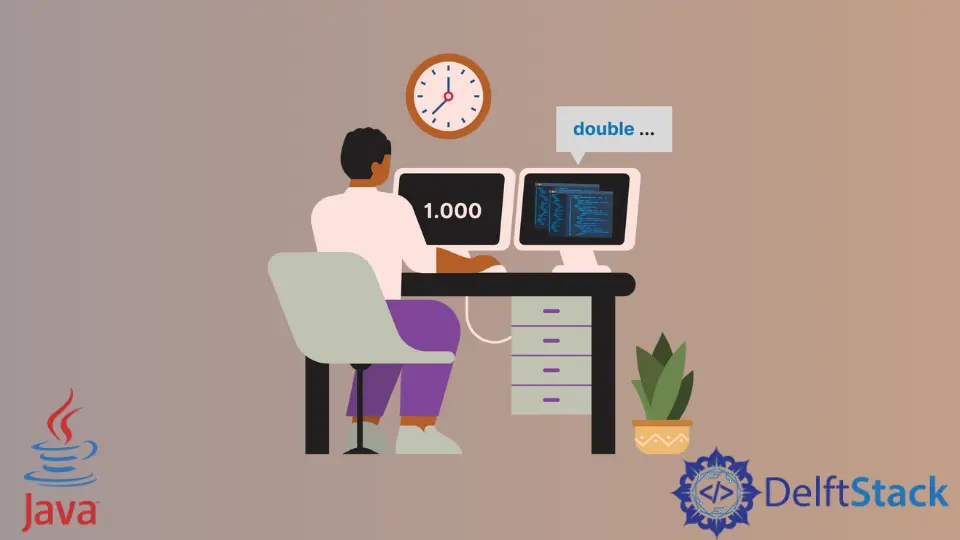
In many real-world applications, precise control over numeric data is essential. Whether you’re working on financial calculations, scientific simulations, or any domain where precision matters, you may encounter the need to truncate or round numbers to a specific number of decimal places.
Java provides various tools and methods to achieve this. In this article, we’ll explore how to truncate or round numbers to N decimal places in Java.
Use DecimalFormat
to Truncate Double in Java
Before we delve into truncating or rounding numbers, let’s understand the basics of the DecimalFormat
class. It is part of the java.text
package and is primarily used for formatting numbers according to specific patterns.
Here’s how you can create a DecimalFormat
object:
import java.text.DecimalFormat;
DecimalFormat df = new DecimalFormat("#.##");
In the example above, #
and 0
in the pattern represent digit placeholders. The #
placeholder allows for optional digits, while 0
represents required digits.
The DecimalFormat
class allows you to round a number to N decimal places by setting the desired pattern and rounding mode. Here’s how you can do it:
import java.math.RoundingMode;
import java.text.DecimalFormat;
public class DecimalFormatExample {
public static void main(String[] args) {
double number = 123.456789;
DecimalFormat df = new DecimalFormat("#.##");
df.setRoundingMode(RoundingMode.HALF_UP);
String result = df.format(number);
System.out.println(result);
}
}
Output:
123.46
This Java code initializes a double
variable number
with the value 123.456789
. Then, it creates a DecimalFormat
object with the pattern #.##
, indicating that the number should be formatted with up to two decimal places, and sets the rounding mode to HALF_UP
.
Finally, it formats the number
using this DecimalFormat
object and prints the result, which will be 123.46
due to the specified rounding mode.
If you need to truncate a number to N decimal places without rounding, you can achieve that with the DecimalFormat
class as well:
import java.text.DecimalFormat;
public class DecimalFormatExample {
public static void main(String[] args) {
double number = 123.456789;
DecimalFormat df = new DecimalFormat("#.00");
String result = df.format(number);
System.out.println(result);
}
}
Output:
123.45
Here, the code defines a double
variable number
with an initial value of 123.456789
. It then creates a DecimalFormat
object with the pattern #.00
, which specifies that the number should be formatted with exactly two decimal places.
Finally, it formats the number
using the DecimalFormat
object and prints the result to the console, resulting in 123.45
.
Sometimes, you may need to format numbers according to a specific locale, such as when dealing with internationalization. You can use the DecimalFormatSymbols
class in combination with DecimalFormat
to achieve this:
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.util.Locale;
public class DecimalFormatLocaleExample {
public static void main(String[] args) {
double number = 1234.56789;
DecimalFormatSymbols symbols = DecimalFormatSymbols.getInstance(Locale.US);
DecimalFormat df = new DecimalFormat("#.##", symbols);
String result = df.format(number);
System.out.println(result);
}
}
Output:
1,234.57
This Java code begins by importing necessary classes and setting up the main
method. Inside the main
method, it defines a double
variable number
with an initial value of 1234.56789
.
It then creates a DecimalFormatSymbols
object with the locale set to Locale.US
, specifying the formatting conventions used in the United States, including a comma as the thousands separator and a period as the decimal separator.
Finally, it creates a DecimalFormat
object with the pattern #.##
and the locale-specific symbols and formats the number
, resulting in 1,234.57,
which is printed to the console.
Use BigDecimal
to Truncate Double in Java
BigDecimal
is a Java class in the java.math
package that provides arbitrary-precision arithmetic. Unlike double
or float
, which are based on IEEE floating-point standards and have limited precision, BigDecimal
can represent and manipulate decimal numbers with high precision, making it suitable for financial calculations, scientific measurements, and other applications where exact numeric representation is critical.
To truncate a double
to N decimal places using BigDecimal
, begin by creating a BigDecimal
object that holds the original number you want to truncate. You can do this using the BigDecimal
constructor or the valueOf
method:
double originalValue = 123.456789;
BigDecimal bd = BigDecimal.valueOf(originalValue);
The scale of a BigDecimal
represents the number of decimal places you want to keep. To truncate the double to N decimal places, you need to set the scale using the setScale
method.
You’ll also specify the rounding mode as RoundingMode.DOWN
to ensure that the extra decimal places are simply discarded:
int decimalPlaces = 2;
bd = bd.setScale(decimalPlaces, RoundingMode.DOWN);
If needed, you can retrieve the truncated value as a BigDecimal
or convert it back to a primitive data type, such as a double
, using the doubleValue
method:
double truncatedValue = bd.doubleValue();
Here’s a complete example:
import java.math.BigDecimal;
import java.math.RoundingMode;
public class BigDecimalTruncationExample {
public static void main(String[] args) {
double originalValue = 123.456789;
BigDecimal bd = BigDecimal.valueOf(originalValue);
int decimalPlaces = 2;
bd = bd.setScale(decimalPlaces, RoundingMode.DOWN);
double truncatedValue = bd.doubleValue();
System.out.println(truncatedValue);
}
}
This code will output the following:
123.46
This code begins by converting the double
to a BigDecimal
, setting the rounding mode to half-up
(which rounds to the nearest value with ties rounding up), and finally, converting the result back to a double
. The output will be 123.46
because the number is rounded to the nearest value with two decimal places.
Use Apache Common Library to Truncate Decimal in Java
While Java’s standard libraries offer some level of support for this, Apache Commons Math, a popular Java library, provides advanced mathematical functions and utilities, including features for precise truncation and rounding of numbers.
To get started, you’ll need to include the Apache Commons Math library in your Java project. You can either download the library JAR file from the Apache Commons Math website or use a dependency management tool like Maven or Gradle to include it in your project.
Here’s an example of how to include it in a Maven project:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-math3</artifactId>
<version>3.6.1</version> <!-- Replace with the latest version -->
</dependency>
Once you have Apache Commons Math in your project, you can proceed with truncating or rounding numbers.
Truncating a number involves removing the decimal places beyond a specified precision. Apache Commons Math provides the Precision.round(double x, int scale)
method to achieve this.
Here’s how you can use it:
import org.apache.commons.math3.util.Precision;
public class TruncateExample {
public static void main(String[] args) {
double numberToTruncate = 12.3456789;
int decimalPlaces = 2; // Set the precision to 2 decimal places
double truncatedNumber = Precision.round(numberToTruncate, decimalPlaces);
System.out.println("Original Number: " + numberToTruncate);
System.out.println("Truncated Number: " + truncatedNumber);
}
}
Output:
Original Number: 12.3456789
Truncated Number: 12.35
In this example, the Precision.round
method takes the number to truncate and the desired precision (number of decimal places). It returns the truncated number, which will have only two decimal places in this case.
Use java.lang.Math
to Truncate Decimal in Java
Another way to truncate or round a number to a specific decimal point is by using Java’s built-in java.lang.Math
library. Specifically, we use the Math.floor()
method in combination with Math.pow()
.
We multiply the original number by 10 to the power of N, truncate the fractional part using Math.floor()
, and then divide by 10 to the power of N to obtain the truncated value.
The Math.floor()
and Math.pow()
methods in Java have the following syntax:
public static double floor(double a)
- It takes a single argument of type
double
, which is the number you want to round down to the nearest integer. - The method returns a
double
value, which is the largest integer that is less than or equal to the given argument.
public static double pow(double a, double b)
- It takes two arguments of type
double
.- The first argument,
a
, is the base. - The second argument,
b
, is the exponent.
- The first argument,
- The method returns a
double
value, which isa
raised to the power ofb
.
Here’s how you can truncate a number using these methods in java.lang.Math
:
public class TruncationExample {
public static void main(String[] args) {
double originalNumber = 123.456789;
int decimalPlaces = 2;
double truncatedValue =
Math.floor(originalNumber * Math.pow(10, decimalPlaces)) / Math.pow(10, decimalPlaces);
System.out.println("Original Number: " + originalNumber);
System.out.println("Truncated Value: " + truncatedValue);
}
}
Output:
Original Number: 123.456789
Truncated Value: 123.45
In this example, originalNumber
is the initial number you want to truncate, set to 123.456789
, and decimalPlaces
represents the number of decimal places to which you want to truncate, set to 2
.
We use Math.pow(10, decimalPlaces)
to get the factor by which we will multiply originalNumber
. In this case, it’s 100
.
Then, Math.floor
is used to round down the result of the multiplication, effectively removing the extra decimal places.
Finally, we divide the result by Math.pow(10, decimalPlaces)
to obtain the truncated value. The result is that originalNumber
is truncated to two decimal places, resulting in 123.45
.
Rounding a number to N decimal places means adjusting the number to the nearest value with the desired precision. You can achieve this with java.lang.Math
as well.
However, we should note that this method may not always give us the expected results due to potential inaccuracies.
Here’s an example:
public class RoundingExample {
public static void main(String[] args) {
double originalNumber = 123.456789;
int decimalPlaces = 2;
double roundedValue =
Math.round(originalNumber * Math.pow(10, decimalPlaces)) / Math.pow(10, decimalPlaces);
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Value: " + roundedValue);
}
}
Output:
Original Number: 123.456789
Rounded Value: 123.46
Here, originalNumber
is the number we want to round, set to 123.456789
, and decimalPlaces
is the number of decimal places you want to round to, set to 2
.
We then use Math.pow(10, decimalPlaces)
to get the factor by which we’ll multiply originalNumber
, which is 100
in this example.
Next, Math.round
is employed to round the result to the nearest integer value.
Finally, we divide the result by Math.pow(10, decimalPlaces)
to obtain the rounded value. The result is that originalNumber
is rounded to two decimal places, yielding 123.46
.
Conclusion
In conclusion, there are several methods to truncate or round a number to N decimal points in Java. The choice of method depends on your specific requirements and coding preferences.
The DecimalFormat
approach provides more control and is suitable for formatting numbers for display, while the other methods offer simpler solutions for basic truncation and rounding tasks.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn