Trim() vs Strip() in Java
-
the
trim()
Method in Java -
the
strip()
Method in Java -
Key Differences Between the
trim()
andstrip()
Methods in Java - Conclusion
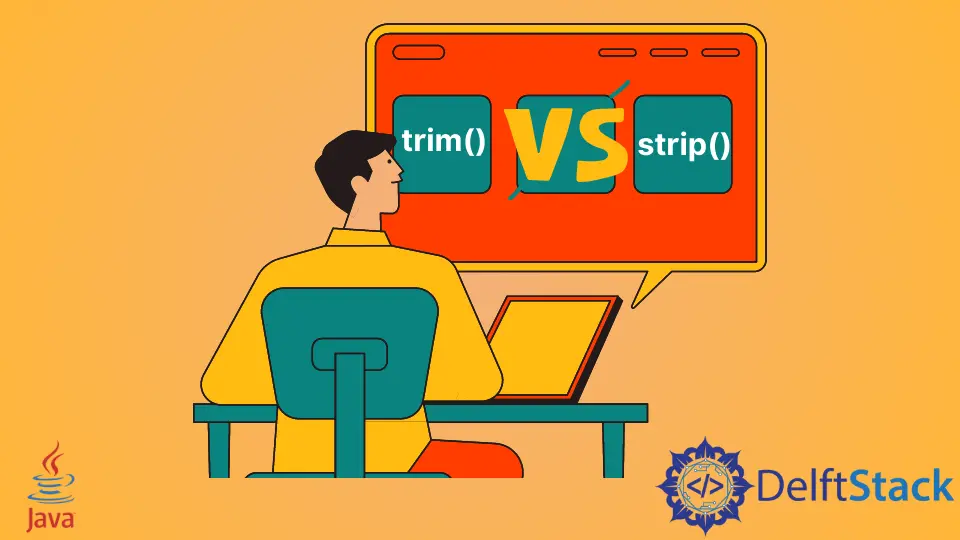
In Java programming, string manipulation is a fundamental aspect. Strings often contain unnecessary white spaces at the beginning or end, which need to be removed for effective processing.
Java provides two methods, trim()
and strip()
, to handle this whitespace removal, but they differ in functionality and application. In this tutorial, we will explore and compare the differences between trim()
and strip()
methods.
the trim()
Method in Java
The trim()
method is a long-standing utility in Java and has been available since the early versions. It is used to remove leading and trailing white spaces (spaces, tabs, and line breaks) from a string.
Below is the syntax of the trim()
method:
public String trim()
The trim()
method does not take any parameters and returns a new string after removing leading and trailing white spaces.
Here’s a simple example demonstrating its usage:
String str = " Hello, World! ";
String trimmedStr = str.trim();
System.out.println("Trimmed String: '" + trimmedStr + "'");
Output:
Trimmed String: 'Hello, World!'
In the example above, the trim()
method is applied to the string str
which contains " Hello, World! "
. The resulting string, trimmedStr
, contains the original string without the leading and trailing spaces.
It’s important to note that the trim()
method will return a new string with the leading and trailing spaces removed. The original string remains unchanged.
The trim()
method only removes spaces (including tabs and line breaks) at the beginning and end of a string. Spaces within the string are not affected.
If the original string consists only of spaces, the trim()
method will return an empty string.
the strip()
Method in Java
Introduced in Java 11 as part of the java.lang.String
class, the strip()
method is designed to remove both leading and trailing white spaces from a string. However, strip()
not only handles the traditional ASCII white spaces (space, tab, line feed, carriage return), but it also removes Unicode whitespace.
Syntax of the strip()
method:
public String strip()
Like trim()
, the strip()
method does not accept any parameters and returns a new string with leading and trailing whitespace removed.
Below is a simple example to illustrate its usage:
String str = " Hello, World! ";
String strippedStr = str.strip();
System.out.println("Stripped String: '" + strippedStr + "'");
Output:
Stripped String: 'Hello, World!'
Here, we initialized the same string str
with leading and trailing spaces. The strip()
method is then applied to str
, removing the spaces.
Lastly, the resulting string, Hello, World!
, is printed without any leading or trailing spaces.
It’s important to note that the strip()
method considers all Unicode whitespace characters for removal. This includes not only the standard ASCII space character but also non-breaking space, various space separators, and other whitespace characters defined in the Unicode standard.
By doing so, strip()
aligns with modern internationalization requirements and ensures a more thorough whitespace removal process.
Since its introduction in Java 11, this method has gained popularity, especially in applications dealing with internationalization and diverse user inputs. However, developers should be mindful of the Java version they are using and ensure compatibility with the minimum required version for strip()
.
Key Differences Between the trim()
and strip()
Methods in Java
The trim()
and strip()
methods are both used for the string type data and somehow perform the same operations. Both methods eliminate the trailing and leading whitespaces from a string.
However, both methods have a few differences. The table below demonstrates the differences between the trim()
and strip()
methods.
trim() |
strip() |
|
---|---|---|
JDK Version | - trim() was added from the JDK version 1.0 |
- strip() was added from the JDK version 11. |
Whitespace Handling | - trim() primarily deals with ASCII whitespace with code point less than or equal to U+0020 , which includes the space character (' ' ), tab ('\t' ), and newline ('\n' ), among others. |
- strip() handles a broader range of characters based on the Unicode standard. This includes Unicode whitespace characters, such as non-breaking space ('\u00A0' ), various space separators, and other Unicode-defined whitespace characters. |
CharSequence Interface | - trim() is specific to the String class and works only on strings. |
- strip() is part of the CharSequence interface, which means it can be used with any class that implements CharSequence , such as String , StringBuilder , StringBuffer , etc. This provides more flexibility in its usage. |
Unicode Considerations | - trim() does not consider Unicode whitespace characters, as it was introduced before comprehensive Unicode support was integrated into Java. |
- strip() was introduced to consider modern internationalization requirements, ensuring that it covers a wider set of whitespace characters based on the Unicode standard. |
Example 1:
public class WhitespaceExample {
public static void main(String[] args) {
String str = " \u2002\u2003 Hello, World! \u2004\u2005 ";
String trimmedStr = str.trim(); // Uses trim()
String strippedStr = str.strip(); // Uses strip()
System.out.println("Original String: \"" + str + "\"");
System.out.println("\nTrimmed String: \"" + trimmedStr + "\"");
System.out.println("\nStripped String: \"" + strippedStr + "\"");
}
}
In this example, the string str
contains various Unicode whitespace characters (\u2002
, \u2003
, \u2004
, \u2005
) in addition to regular spaces.
Based on what we learned above, the trim()
method will only remove the regular spaces (ASCII whitespace), while the strip()
method will remove all leading and trailing whitespace, including Unicode whitespace characters.
Here’s the output:
Original String: " ?? Hello, World! ?? "
Trimmed String: "?? Hello, World! ??"
Stripped String: "Hello, World!"
Let’s try another example to show the use of trim()
and strip()
.
Example 2:
public class Trim_Vs_Strip {
public static void main(String[] args) {
// case 1
final String DemoString1 = " www.delftstack.com ";
System.out.println(DemoString1.trim().equals(DemoString1.strip()));
// case 2
final String DemoString3 = "\t www.delftstack.com \r";
System.out.println(DemoString3.trim().equals(DemoString3.strip()));
// case 3
final String DemoString2 = "www.delftstack.com \u2005";
System.out.println(DemoString2.trim().equals(DemoString2.strip()));
// case 4
final String DemoString4 = "\u2001";
System.out.println(DemoString4.trim().equals(DemoString4.strip()));
}
}
Output:
true
true
false
false
There are four different cases shown in the example code:
Case 1: Simple String With Leading and Trailing Spaces.
final String DemoString1 = " www.delftstack.com ";
System.out.println(DemoString1.trim().equals(DemoString1.strip()));
// return --> true
In this case, a string with leading and trailing spaces is created. Both trim()
and strip()
methods are applied to this string, and they both remove the leading and trailing spaces, resulting in the same trimmed string.
The equals()
method is then used to compare the trimmed strings, and it returns true
.
Case 2: String With Leading \t
and Trailing \r
Spaces.
final String DemoString3 = "\t www.delftstack.com \r";
System.out.println(DemoString3.trim().equals(DemoString3.strip()));
// return --> true
Here, a string with a leading tab and trailing carriage return characters is created. Both trim()
and strip()
methods remove these characters, resulting in the same trimmed string.
The equals()
method returns true
for the comparison.
Case 3: String With Whitespace Unicode \u2005
.
final String DemoString2 = "www.delftstack.com \u2005";
System.out.println(DemoString2.trim().equals(DemoString2.strip()));
// return --> false
In this case, a string with a Unicode whitespace character (\u2005
) at the end is created. The trim()
method can remove regular spaces, but it doesn’t recognize this specific Unicode whitespace character.
However, strip()
does remove this character. As a result, the trimmed strings are not equal, and the equals()
method returns false
.
Case 4: String With Whitespace Unicode \u2001
.
final String DemoString4 = "\u2001";
System.out.println(DemoString4.trim().equals(DemoString4.strip()));
// return --> false
Here, a string with a Unicode whitespace character (\u2001
) is created. Both trim()
and strip()
methods are applied, but since this character is a Unicode whitespace, both methods don’t affect the string.
The trimmed strings are equal, and the equals()
method returns false
.
Conclusion
Understanding the differences between trim()
and strip()
methods is crucial for effective string manipulation in Java. The choice of approach depends on your specific requirements.
If you need to handle only ASCII whitespaces, trim()
is sufficient. However, if you require support for Unicode whitespace or are working with Java 11 or later versions, strip()
is the recommended method to ensure comprehensive whitespace removal.
Choose the appropriate method based on your use case to optimize your code and achieve desired outcomes.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook