How to Trim a String in Java
-
Trim a String Using the
trim()
Method in Java -
Trim a String From Left and Right Separately Using
Character.isWhiteSpace
andwhile
- Trim a String From Left and Right Separately Using Apache Commons
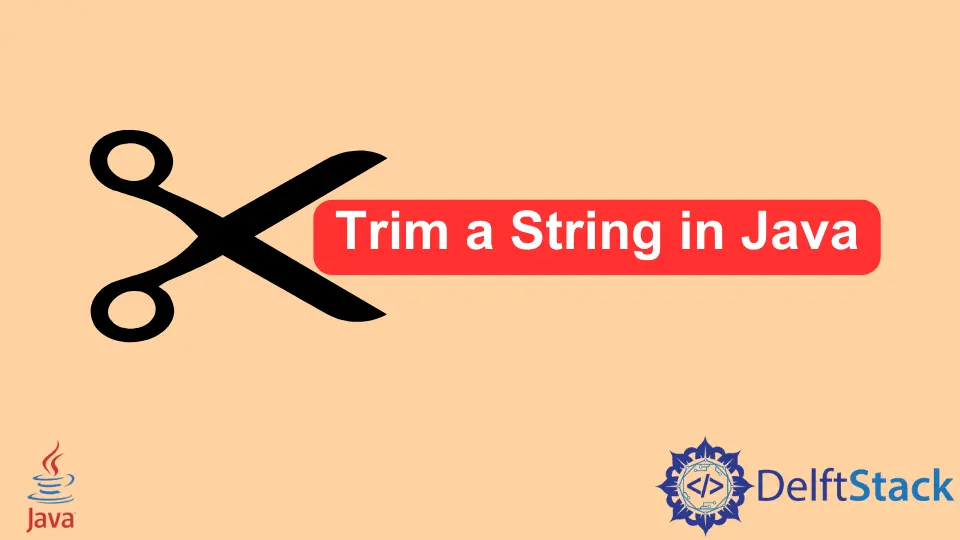
In this article, we will see how to trim a string with whitespaces at the starting or ending points.
Trim a String Using the trim()
Method in Java
The recommended way to trim is to use the trim()
method if we want to trim leading and trailing spaces from a string from both sides. trim()
removes the spaces and returns a string without the leading trailing spaces.
Below, we take a string stringToTrim
that has a string with spaces on both starting and ending points. To check if the spaces were eliminated, we print the string before and after it is trimmed. The plus (+) signs show where the whitespaces are in the string.
When we call stringToTrim.trim()
, we notice that the spaces were removed.
public class TrimString {
public static void main(String[] args) {
String stringToTrim = " This is just an example ";
System.out.println("Before trimming +++" + stringToTrim + "+++");
stringToTrim = stringToTrim.trim();
System.out.println("After trimming +++" + stringToTrim + "+++");
}
}
Output:
Before trimming +++ This is just an example +++
After trimming +++This is just an example+++
Trim a String From Left and Right Separately Using Character.isWhiteSpace
and while
We can trim a string from just a single side, whether it is from the left side or right side. Here we see two examples: one that trims from the left side and another that only removes spaces from the right side.
In the following code, we take a String stringToTrim
with spaces at both ends. We take an int
variable i
and initialize it with 0. Then we run a while
loop that runs until the i
variable is less than the length of stringToTrim
and also check for whitespace characters using Character.isWhitespace()
and inside the method, we pass every character one by one using the i
value. Both the conditions should be met, and if they are true, the value of i
increases by one.
Now we call substring()
to trim the whitespace characters. substring()
starts from the left side of the string, so it trims the left side of the string and returns the string without spaces on the left.
public class TrimString {
public static void main(String[] args) {
String stringToTrim = " This is just an example ";
int i = 0;
while (i < stringToTrim.length() && Character.isWhitespace(stringToTrim.charAt(i))) {
i++;
}
String leftTrim = stringToTrim.substring(i);
System.out.println("Before trimming +++" + stringToTrim + "+++");
System.out.println("After trimming +++" + leftTrim + "+++");
}
}
Output:
Before trimming +++ This is just an example +++
After trimming +++This is just an example +++
In this example, we take the same string, but instead of initializing i
with 0, we initialize it with stringToTrim().length - 1
and then in while
, we check if i
is greater than or equal to 0. The method to check the whitespaces is the same. Now when the conditions meet, we decrease the i
variable by one.
As the substring()
starts from the left side of the string, we pass the starting point as 0, and the second argument is the value that starts from the right i+1
that eliminates all the whitespaces from the right end.
public class TrimString {
public static void main(String[] args) {
String stringToTrim = " This is just an example ";
int i = stringToTrim.length() - 1;
while (i >= 0 && Character.isWhitespace(stringToTrim.charAt(i))) {
i--;
}
String rightTrim = stringToTrim.substring(0, i + 1);
System.out.println("Before trimming +++" + stringToTrim + "+++");
System.out.println("After trimming +++" + rightTrim + "+++");
}
}
Output:
Before trimming +++ This is just an example +++
After trimming +++ This is just an example+++
Trim a String From Left and Right Separately Using Apache Commons
For this example, we use a third party library called Apache Commons. To include the library in our project we use the following dependency.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.11</version>
</dependency>
To trim the string stringToTrim
from the left side, we use StringUtils.stringStart()
and pass the string to trim and the character to trim null
that is treated as whitespace. We use StringUtils.stripEnd()
to trim from the right side and follow the same process.
import org.apache.commons.lang3.StringUtils;
public class TrimString {
public static void main(String[] args) {
String stringToTrim = " This is just an example ";
String leftTrim = StringUtils.stripStart(stringToTrim, null);
String rightTrim = StringUtils.stripEnd(stringToTrim, null);
System.out.println("Before trimming +++" + stringToTrim + "+++");
System.out.println("After left trimming +++" + leftTrim + "+++");
System.out.println("After right trimming +++" + rightTrim + "+++");
}
}
Output:
Before trimming +++ This is just an example +++
After left trimming +++This is just an example +++
After right trimming +++ This is just an example+++
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn