How to Get Today's Date in Java
- Using java.util.Date
- Using java.time.LocalDate
- Using java.time.LocalDateTime
- Using java.time.ZonedDateTime
- Conclusion
- FAQ
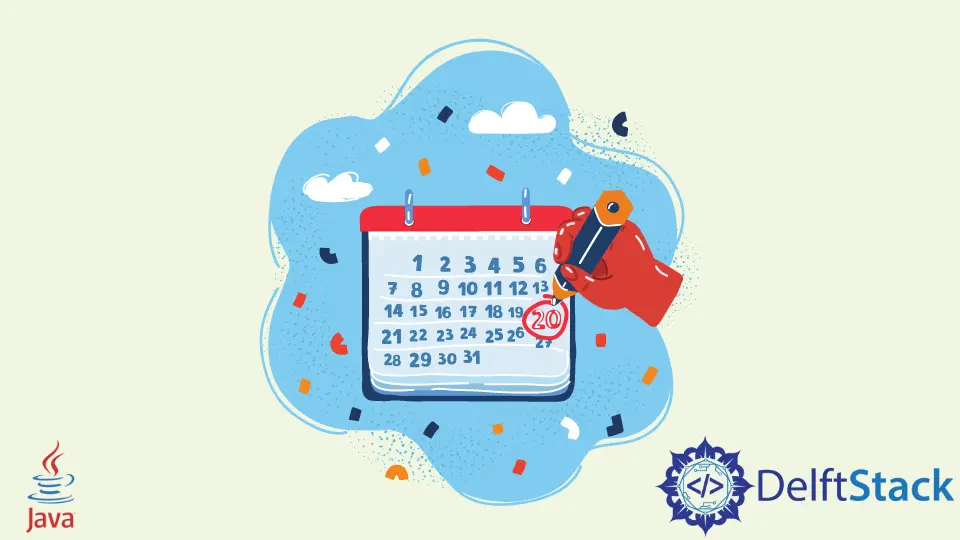
Getting today’s date in Java is a fundamental task that many developers need to accomplish. Whether you’re building a web application, a desktop program, or a mobile app, knowing how to retrieve the current date is essential.
In this tutorial, we’ll explore various methods to get today’s date using Java’s built-in libraries. From the traditional java.util.Date
to the more modern java.time
package introduced in Java 8, we’ve got you covered. By the end of this article, you will have a solid understanding of how to work with dates in Java. Let’s dive in!
Using java.util.Date
The java.util.Date
class is one of the oldest ways to handle dates in Java. While it’s not recommended for new applications due to its limitations, it’s still important to understand how it works. Here’s how you can get today’s date using this class:
import java.util.Date;
public class TodayDate {
public static void main(String[] args) {
Date today = new Date();
System.out.println(today);
}
}
Output:
Mon Oct 23 12:45:30 UTC 2023
In this code, we import the Date
class from the java.util
package. We then create an instance of Date
called today
, which automatically initializes to the current date and time when instantiated. Finally, we print the date to the console. The output format may vary depending on your locale and time zone, but it provides a quick way to see the current date and time.
While java.util.Date
is simple and straightforward, it has some drawbacks, such as being mutable and not thread-safe. For more robust applications, consider using the newer java.time
package.
Using java.time.LocalDate
Introduced in Java 8, the java.time
package provides a more comprehensive and user-friendly way to work with dates and times. The LocalDate
class is specifically designed for representing dates without time zones. Here’s how to get today’s date using LocalDate
:
import java.time.LocalDate;
public class TodayDate {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
System.out.println(today);
}
}
Output:
2023-10-23
In this example, we import the LocalDate
class from the java.time
package. The now()
method retrieves the current date in the system’s default time zone. The output format is ISO-8601, which is a standard format for dates. This method is not only simpler but also more reliable than using java.util.Date
.
LocalDate is immutable, meaning once created, its value cannot be changed. This characteristic makes it safer to use in multi-threaded applications. Additionally, LocalDate
provides various methods for date manipulation, such as adding or subtracting days, months, or years.
Using java.time.LocalDateTime
If you need both date and time, LocalDateTime
is the go-to class. It represents a date-time without a time zone in the ISO-8601 calendar system. Here’s how you can get the current date and time:
import java.time.LocalDateTime;
public class TodayDate {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
System.out.println(now);
}
}
Output:
2023-10-23T12:45:30.123456789
In this code, we use the LocalDateTime
class to fetch the current date and time. The now()
method is again employed, and the output will include both the date and the time, formatted as an ISO-8601 string.
Using LocalDateTime
is particularly useful for applications that require precise time stamps, such as logging events or scheduling tasks. Like LocalDate
, LocalDateTime
is also immutable, ensuring thread safety and reliability in concurrent environments.
Using java.time.ZonedDateTime
For applications that need to handle time zones, ZonedDateTime
is the best choice. This class combines the functionality of LocalDateTime
with time zone information. Here’s how to get today’s date and time with a specific time zone:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TodayDate {
public static void main(String[] args) {
ZonedDateTime zonedNow = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println(zonedNow);
}
}
Output:
2023-10-23T08:45:30.123456789-04:00[America/New_York]
In this example, we import ZonedDateTime
and ZoneId
from the java.time
package. By calling ZonedDateTime.now()
and passing a specific ZoneId
, we can fetch the current date and time for that time zone. The output includes both the local date and time as well as the offset from UTC and the time zone ID.
Using ZonedDateTime
is essential for applications that operate across multiple time zones, such as global scheduling systems or flight tracking apps. This class helps you avoid common pitfalls associated with time zone conversions, ensuring that your application behaves correctly regardless of the user’s location.
Conclusion
In this tutorial, we’ve explored various methods to get today’s date in Java, ranging from the legacy java.util.Date
to the modern and versatile java.time
package. Each method has its own advantages, and understanding them will help you choose the right one for your specific needs. Whether you’re developing a simple application or a complex system, knowing how to work with dates and times is crucial. As you continue your journey in Java programming, keep these methods in your toolkit for effective date handling.
FAQ
-
What is the difference between java.util.Date and java.time.LocalDate?
java.util.Date is an older class that represents a specific instant in time, while java.time.LocalDate is part of the newer Java Date and Time API and represents a date without time zone information. -
Why should I use LocalDate instead of Date?
LocalDate is immutable and thread-safe, making it a better choice for modern applications. It also provides a clearer API for date manipulation. -
Can I get the current date in a specific time zone?
Yes, you can use ZonedDateTime along with ZoneId to get the current date and time in a specific time zone. -
Is LocalDateTime thread-safe?
Yes, LocalDateTime is immutable, which makes it thread-safe and suitable for concurrent applications.
- How do I format the date output in Java?
You can use the DateTimeFormatter class from the java.time.format package to format date and time outputs according to your needs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn