How to Subtract Dates in Java
-
Use
java.util.Date
to Subtract Two Dates in Java -
Use
java.time.Duration
andjava.time.Period
to Subtract Two Dates in Java -
Use
java.time.temporal.ChronoUnit
to Subtract Two Dates in Java -
Use the
java.time.temporal.Temporal
until()
Method to Subtract Two Dates in Java -
Use
java.util.Calendar
to Subtract Two Dates in Java - Use Third-Party Libraries Method to Subtract Two Dates in Java
- Conclusion
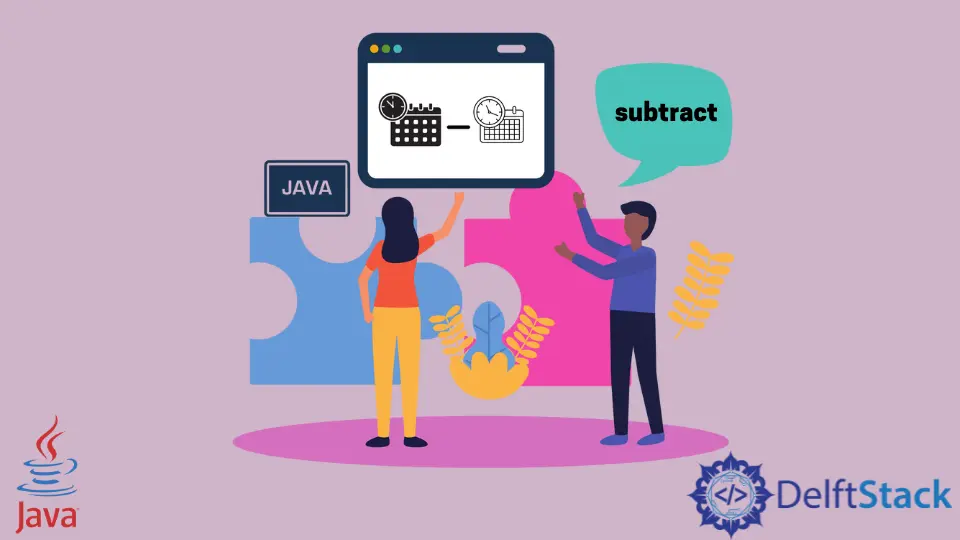
Manipulating and calculating differences between dates is a fundamental task in many Java applications.
This article delves into various methods to subtract two dates, providing a comprehensive overview of approaches available in Java.
Use java.util.Date
to Subtract Two Dates in Java
Java provides a robust set of tools for managing date-related operations, and the java.util.Date
class provides a straightforward way to subtract two dates. This class in Java represents a specific instant in time with millisecond precision.
While java.util.Date
has been largely superseded by the java.time
package in Java 8 and later versions, understanding date subtraction using the older class can still be useful in certain scenarios.
To subtract two dates, you need to obtain the values of each date and then find the difference between them. Here’s a code example:
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.concurrent.TimeUnit;
public class DateSubtractionExample {
public static void main(String[] args) throws Exception {
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy", Locale.ENGLISH);
Date firstDate = sdf.parse("04/22/2020");
Date secondDate = sdf.parse("04/27/2020");
long timeDifferenceInMillis = secondDate.getTime() - firstDate.getTime();
TimeUnit time = TimeUnit.DAYS;
long differenceInDays = time.convert(timeDifferenceInMillis, TimeUnit.MILLISECONDS);
System.out.println("The difference in days is : " + differenceInDays);
}
}
In this example, we begin by importing necessary packages, including java.text.SimpleDateFormat
, java.util.Date
, java.util.Locale
, and java.util.concurrent.TimeUnit
. The SimpleDateFormat
class is used to parse dates with a specific format.
Next, we create instances of SimpleDateFormat
for date parsing and parse two dates, 04/22/2020
and 04/27/2020
. The getTime()
method is then applied to each Date
object to retrieve the time in milliseconds.
Subsequently, we calculate the time difference between the two dates in milliseconds by subtracting the first date’s time from the second date’s time. This difference is stored in the variable timeDifferenceInMillis
.
To convert the time difference to days, we use the TimeUnit
class and its convert()
method. The result, stored in the variable differenceInDays
, represents the absolute difference between the two dates in days.
Output:
The difference in days is : 5
While java.util.Date
is considered legacy, it still serves its purpose in certain situations. However, for more advanced date and time operations, it’s recommended to use the java.time
package introduced in Java 8 and later.
Use java.time.Duration
and java.time.Period
to Subtract Two Dates in Java
With the introduction of the java.time
package in Java 8, working with dates and times has become more flexible and feature-rich. Here, we will see how to subtract two dates in Java using the java.time.Duration
and java.time.Period
classes.
These classes provide a more sophisticated and precise way of handling time intervals and date differences compared to the older java.util.Date
class.
Duration
represents a duration of time, such as 5 hours or 30 seconds, with a focus on the amount of time in terms of seconds and nanoseconds. On the other hand, Period
represents a period of time-based values, such as 3 months or 2 years, with a focus on date-based components like years, months, and days.
Here’s a simple example demonstrating the use of Duration
and Period
:
import java.time.Duration;
import java.time.LocalDate;
import java.time.Period;
import java.time.format.DateTimeFormatter;
public class DateSubtractionJavaTimeExample {
public static void main(String[] args) throws Exception {
LocalDate d1 = LocalDate.parse("2020-05-06", DateTimeFormatter.ISO_LOCAL_DATE);
LocalDate d2 = LocalDate.parse("2020-05-30", DateTimeFormatter.ISO_LOCAL_DATE);
LocalDate d3 = LocalDate.parse("2018-05-06", DateTimeFormatter.ISO_LOCAL_DATE);
LocalDate d4 = LocalDate.parse("2020-01-23", DateTimeFormatter.ISO_LOCAL_DATE);
Duration diff = Duration.between(d1.atStartOfDay(), d2.atStartOfDay());
Period period = Period.between(d3, d4);
long diffDays = diff.toDays();
int years = Math.abs(period.getYears());
int months = Math.abs(period.getMonths());
int days = Math.abs(period.getDays());
System.out.println("Difference between dates is : " + diffDays + " days");
System.out.println(
"Difference is : " + years + " year, " + months + " months, " + days + " days");
}
}
This example also begins by importing the necessary packages, including java.time.Duration
, java.time.LocalDate
, java.time.Period
, and java.time.format.DateTimeFormatter
. The DateTimeFormatter
is used to parse dates in the ISO_LOCAL_DATE
format.
We then parse two pairs of local dates: d1
and d2
, as well as d3
and d4
, for illustration purposes.
For the first set of dates (d1
and d2
), we calculate the duration between two instants using Duration.between(d1.atStartOfDay(), d2.atStartOfDay())
. This gives us the time difference in seconds and nanoseconds.
For the second set of dates (d3
and d4
), we compute the period between them using Period.between(d3, d4)
. This gives us the difference in years, months, and days.
Next, we convert the duration (diff
) to days using diff.toDays()
. Additionally, we extract the individual components (years, months, days) from the period using methods like period.getYears()
.
Output:
Difference between dates is : 24 days
Difference is : 1 year, 8 months, 17 days
In this output, the first line represents the time difference in days between the dates 2020-05-06
and 2020-05-30
. The second line shows the difference in years, months, and days between the dates 2018-05-06
and 2020-01-23
.
Using java.time.Duration
and java.time.Period
provides a more modern and feature-rich approach to date subtraction compared to the older java.util.Date
class. This method allows for more granular control over different units of time and is particularly useful for handling diverse date-related scenarios in Java applications.
Use java.time.temporal.ChronoUnit
to Subtract Two Dates in Java
In Java 8 and later, the java.time.temporal.ChronoUnit
class provides a versatile and convenient way to subtract two dates. Unlike the previous examples, which utilized Duration
and Period
, ChronoUnit
directly calculates the time difference in terms of specific units.
ChronoUnit
is an enumeration that represents a unit of time within the context of the broader java.time.temporal
package. It provides a set of predefined constants for common units such as days, months, years, hours, minutes, and seconds.
Using ChronoUnit
simplifies date subtraction by allowing us to specify the desired unit directly.
Below is a simple example demonstrating the use of ChronoUnit
:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
public class DateSubtractionChronoUnitExample {
public static void main(String[] args) throws Exception {
LocalDate dBefore = LocalDate.parse("2018-05-06", DateTimeFormatter.ISO_LOCAL_DATE);
LocalDate dAfter = LocalDate.parse("2018-05-30", DateTimeFormatter.ISO_LOCAL_DATE);
long diff = ChronoUnit.DAYS.between(dBefore, dAfter);
System.out.println("Difference is : " + diff + " days");
}
}
This example begins by importing the necessary packages, including java.time.LocalDate
, java.time.format.DateTimeFormatter
, and java.time.temporal.ChronoUnit
. The DateTimeFormatter
is used to parse dates in the ISO_LOCAL_DATE
format.
We then parse two local dates, dBefore
and dAfter
.
The crucial step is the calculation of the difference in days using ChronoUnit.DAYS.between(dBefore, dAfter)
. The ChronoUnit
class provides a set of predefined units, and in this case, we use the DAYS
enum to calculate the difference in days between the two dates.
Output:
Difference is : 24 days
In this output, the line indicates the difference in days between the dates 2018-05-06
and 2018-05-30
. Using ChronoUnit
simplifies the process, allowing us to specify the unit of time directly and obtain precise results.
This method is particularly beneficial when you need to calculate differences in specific units and prefer a more straightforward solution compared to working with Duration
and Period
classes.
Use the java.time.temporal.Temporal
until()
Method to Subtract Two Dates in Java
The Temporal
interface serves as the base interface for most classes in the java.time
package, including LocalDate
and LocalDateTime
. It defines various methods for manipulating date and time values.
The until()
method allows us to calculate the amount of time between two temporal objects, providing another flexible way to subtract dates.
Take a look at the example below demonstrating the use of the Temporal
interface’s until()
method:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
import java.time.temporal.Temporal;
public class DateSubtractionTemporalExample {
public static void main(String[] args) throws Exception {
LocalDate dBefore = LocalDate.parse("2018-05-21", DateTimeFormatter.ISO_LOCAL_DATE);
LocalDate dAfter = LocalDate.parse("2018-05-30", DateTimeFormatter.ISO_LOCAL_DATE);
long diff = dBefore.until(dAfter, ChronoUnit.DAYS);
System.out.println("Difference is : " + diff + " days");
}
}
This example begins by importing the necessary packages, including java.time.LocalDate
, java.time.format.DateTimeFormatter
, java.time.temporal.ChronoUnit
, and java.time.temporal.Temporal
. The DateTimeFormatter
is used to parse dates in the ISO_LOCAL_DATE
format.
We then parse two local dates, dBefore
and dAfter
.
The crucial step is the calculation of the difference in days using the until()
method of the Temporal
interface: dBefore.until(dAfter, ChronoUnit.DAYS)
. The ChronoUnit.DAYS
enum is specified as the unit of time.
Output:
Difference is : 9 days
In this output, the line indicates the difference in days between the dates 2018-05-21
and 2018-05-30
. Using the until()
method of the Temporal
interface allows us to directly specify the unit of time and obtain precise results.
Use java.util.Calendar
to Subtract Two Dates in Java
In Java, the java.util.Calendar
class, although considered less intuitive than the newer java.time
package, provides a way to manipulate dates and times. It supports various calendar systems and time zones.
This class has been a traditional choice for handling date and time operations before the introduction of the more modern java.time
package in Java 8.
Consider the example below:
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DateSubtractionCalendarExample {
public static void main(String[] args) throws Exception {
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
Date date1 = sdf.parse("04/22/2020");
Date date2 = sdf.parse("04/27/2020");
Calendar calendar1 = Calendar.getInstance();
calendar1.setTime(date1);
Calendar calendar2 = Calendar.getInstance();
calendar2.setTime(date2);
long timeDifferenceInMillis = calendar2.getTimeInMillis() - calendar1.getTimeInMillis();
long differenceInDays = timeDifferenceInMillis / (24 * 60 * 60 * 1000);
System.out.println("Difference is : " + differenceInDays + " days");
}
}
This example demonstrates how to subtract two dates using java.util.Calendar
. We start by importing the necessary classes, including SimpleDateFormat
, Calendar
, and Date
.
Two date strings (04/22/2020
and 04/27/2020
) are parsed using SimpleDateFormat
. Calendar instances (calendar1
and calendar2
) are then created and set to the parsed dates.
The difference between the two dates is calculated in milliseconds using calendar2.getTimeInMillis() - calendar1.getTimeInMillis()
. This difference is then converted to days by dividing it by the number of milliseconds in a day (24 * 60 * 60 * 1000
).
Output:
Difference is : 5 days
In this output, the line indicates the difference in days between the dates 04/22/2020
and 04/27/2020
.
While java.util.Calendar
might be considered less elegant than the newer date and time APIs, it still provides a viable option for date manipulations in certain scenarios. However, if possible, it’s recommended to use the more modern java.time
package for improved functionality and readability.
Use Third-Party Libraries Method to Subtract Two Dates in Java
While Java’s standard library provides powerful tools for date manipulation, third-party libraries such as Joda-Time offer additional features and flexibility.
Joda-Time is an open-source date and time library for Java, designed to overcome some of the limitations and complexities present in the original java.util.Date
and java.util.Calendar
classes. Joda-Time provides a rich set of classes for date, time, and duration calculations.
To use Joda-Time in your project, you need to include the Joda-Time library. If you’re using Maven, you can add the following dependency to your pom.xml
file:
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
<version>2.10.13</version>
</dependency>
If you are using a different build tool or manually managing dependencies, ensure that the Joda-Time JAR file is included.
Here’s a code example demonstrating the use of Joda-Time to subtract two dates:
import org.joda.time.DateTime;
import org.joda.time.Days;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
public class DateSubtractionJodaTimeExample {
public static void main(String[] args) {
String dateString1 = "2020-05-06";
String dateString2 = "2020-05-30";
DateTimeFormatter formatter = DateTimeFormat.forPattern("yyyy-MM-dd");
DateTime date1 = DateTime.parse(dateString1, formatter);
DateTime date2 = DateTime.parse(dateString2, formatter);
int differenceInDays = Days.daysBetween(date1, date2).getDays();
System.out.println("Difference is : " + differenceInDays + " days");
}
}
This example demonstrates how to use Joda-Time to subtract two dates. We start by importing necessary classes from the Joda-Time library, including DateTime
, Days
, and DateTimeFormatter
.
Two date strings (dateString1
and dateString2
) are then parsed using a specified date format. We use the DateTimeFormatter
to define the format as yyyy-MM-dd
and parse each date string into Joda-Time DateTime
objects.
The difference in days is calculated using Days.daysBetween(date1, date2).getDays()
, and the result is stored in the variable differenceInDays
.
Output:
Difference is : 24 days
In this output, the line indicates the difference in days between the dates 2020-05-06
and 2020-05-30
. Using Joda-Time provides an alternative to Java’s standard date and time APIs, offering additional features and a different syntax for date calculations.
Conclusion
In this article, we’ve covered various methods to subtract two dates in Java. Depending on your Java version and project requirements, you can choose the approach that best suits your needs.
Whether it’s the modern java.time
package, third-party libraries like Joda-Time, or the traditional java.util.Date
and java.util.Calendar
, these methods offer flexibility and functionality for diverse date manipulation scenarios.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn