String Template in Java
-
Replace a Substring That Is Similar to a
velocity template
- An Alternative Way to Replace String in Java
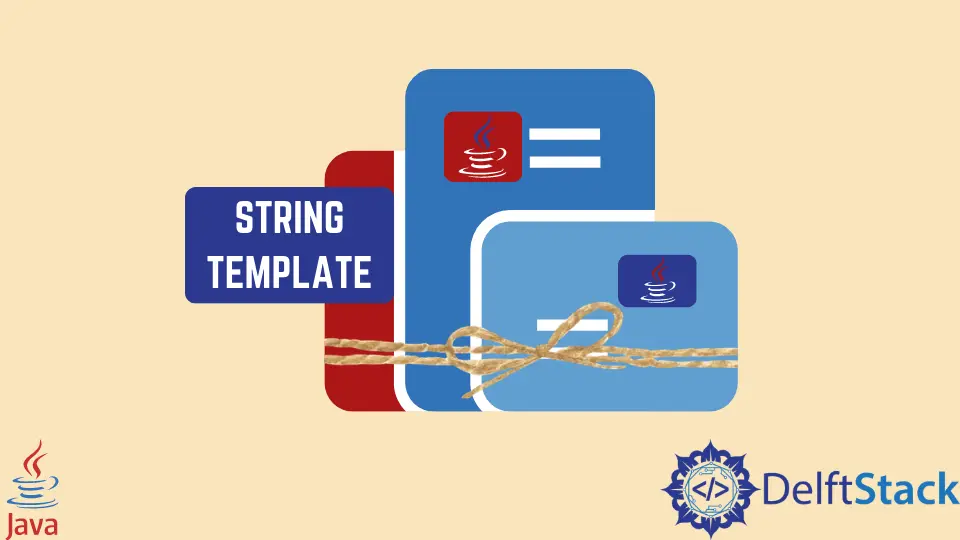
If you are writing a program in Java that can work with strings, you may require some way to replace specific strings.
This article will show how we can replace a string in Java. Also, we will discuss the topic by using necessary examples and explanations to make the topic easier.
We will discuss two different methods in this article to replace substrings.
Replace a Substring That Is Similar to a velocity template
In our below example, we will replace a string that is most similar to the velocity template
. The code for our example will be like the below.
// Importing necessary packages
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.text.StringSubstitutor;
public class StringReplace {
public static void main(String args[]) {
Map<String, String> MyMap = new HashMap<String, String>(); // Declaring a Map
MyMap.put("Name", "Alen Walker"); // Creating a replacement
String MyString = "Good Morning!! ${Name}"; // Our main string
StringSubstitutor SubStr = new StringSubstitutor(MyMap);
String FinalString = SubStr.replace(MyString); // Replacing the string
System.out.println(FinalString);
}
}
In our example above, we first created an object for mapping the string.
Then we put our replacement string to the MyMap
object. Now we initiated a string variable named MyString
.
Then we created an object for StringSubstitutor
and passed our MyMap
object in it. After that, we declared another string variable where we stored our updated string.
Lastly, we just printed the updated string. You will get the output below after running the code.
Good Morning!! Alen Walker
Please note that you need to import the necessary jar
file from Apache
for the package org.apache.commons.text.StringSubstitutor;
, which is in this link. Otherwise, it will show you an error.
An Alternative Way to Replace String in Java
In our example below, we will look at a very easy way to replace a string. You can follow this method if you don’t want to use external jar
files.
The code for our example will be like the below.
public class ReplaceStr {
public static void main(String args[]) {
String MyStr = "Good Morning!!! <YOUR_NAME>"; // Our main string
String replaceString = MyStr.replace("<YOUR_NAME>", "Alen Walker"); // Replacing the string
System.out.println(replaceString);
}
}