How to Reverse a String Recursively in Java
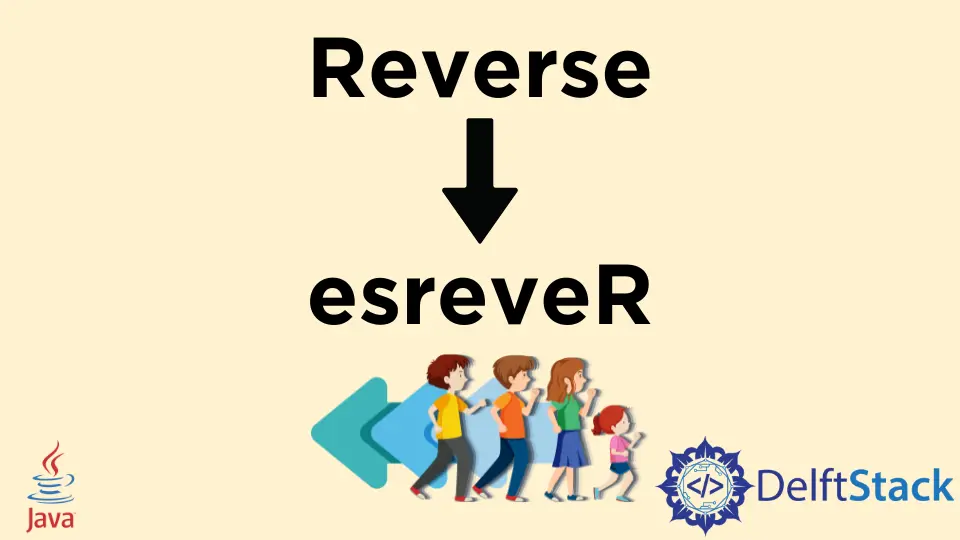
Recursion is the process that calls the function itself repeatedly until a breaking condition meets the criteria. It allows the user to call the function itself from itself. Here are a few conditions that must satisfy to make a function recursive:
- The function must call itself from the same function.
- A terminating condition is needed to break the recursive loop from getting called repeatedly.
- The recursion uses Stack data structure in its course of action. It stores variables and function calls in its storage.
Below is the code block to reverse a String using Recursion in Java.
import java.util.Scanner;
public class StringReverseUsingRecursion {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter any String :");
String str = scanner.nextLine();
System.out.println("The reverse of the String is : " + recursiveReverse(str));
}
static String recursiveReverse(String input) {
if ((input == null) || (input.length() <= 1))
return input;
else {
return recursiveReverse(input.substring(1)) + input.charAt(0);
}
}
}
In the above code block, the class holds a static method and the main
method. The recursiveReverse()
method is the static recursive function that reverses a string using recursion. It takes an input parameter and also returns a String value.
In the main
method, the Scanner class gets instantiated using the new keyword. The constructor of the Scanner class takes the Input Stream as a parameter and produces the input string after scanning from the input source.
It also converts the byte stream in the default character set that is the UTF-8 standard set. The constructor throws IllegalArgumentException
if unable to convert the data to the default character set.
The System is a class java.lang
package it represents the standard input stream instance methods and variables. In
is the instance variable of the input stream that is open and ready to supply input data.
This stream uses the keyboard input or another input source specified by the user.
The nextLine()
function returns the current line, excluding the line separator at the end. Hence this will store a user-defined input line in the str
variable. The String gets later passed to the static recursive method.
The terminating condition in the static recursiveReverse()
method is to check the input is null or less than one, then return the input string itself.
If the condition does not satisfy, it again calls the function itself but with the substring excluding zeroeth character. The substring()
function creates a substring of the actual String and passes that smaller stream to the same method again.
Hence it gets called iteratively, and in the end, starts returning characters in response.
Output:
Enter any String :
Hello
The reverse of the String is : olleH.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn