Comparison Between string.equals() vs == in Java
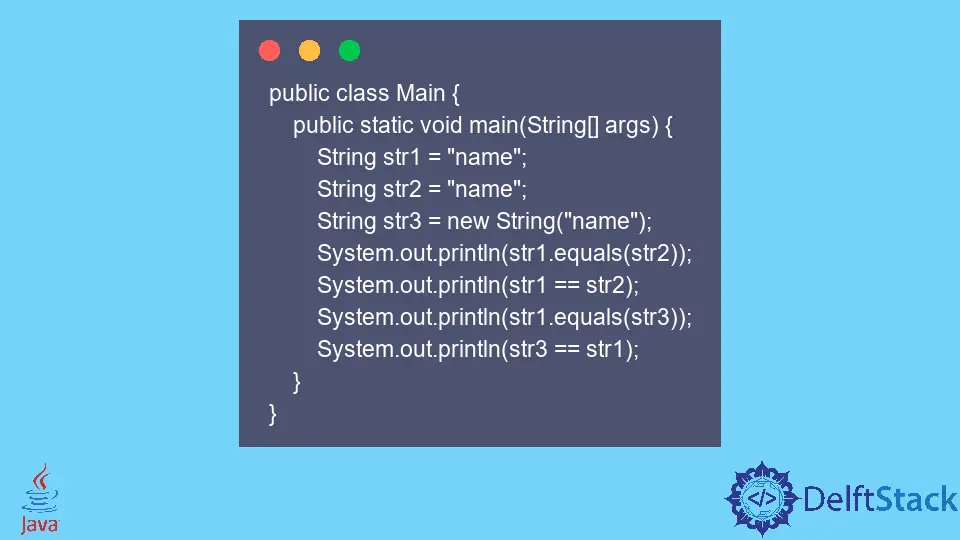
Java, a widely-used programming language, has its own unique way of handling strings. When it comes to comparing strings, developers often find themselves at a crossroads between using string.equals()
and the ==
operator. While both methods may seem similar at first glance, they serve very different purposes and can lead to unexpected results if not understood properly.
In this article, we’ll delve into the distinctions between these two approaches to string comparison in Java. By the end, you’ll have a clearer understanding of when to use each method, helping you write more efficient and error-free code. Let’s dive into the world of Java string comparisons!
Understanding string.equals()
The string.equals()
method is designed specifically for comparing the content of two strings. This means that when you use string.equals()
, Java checks whether the actual characters within the strings are identical. This method is case-sensitive, meaning “Hello” and “hello” would not be considered equal.
Here’s how you can use string.equals()
in your Java code:
public class StringComparison {
public static void main(String[] args) {
String str1 = "Java";
String str2 = "Java";
String str3 = new String("Java");
boolean result1 = str1.equals(str2);
boolean result2 = str1.equals(str3);
System.out.println("str1 equals str2: " + result1);
System.out.println("str1 equals str3: " + result2);
}
}
Output:
str1 equals str2: true
str1 equals str3: true
In this example, both result1
and result2
return true
. This is because string.equals()
compares the actual content of the strings, not their memory addresses. Regardless of how the strings are created, as long as the content is the same, string.equals()
will confirm their equality.
Understanding == Operator
On the other hand, the ==
operator compares the memory addresses of the string objects. This means that it checks whether both references point to the same object in memory. If they do, the ==
operator returns true
; otherwise, it returns false
. This can lead to confusion, especially for new Java developers who might expect ==
to compare string content.
Here’s how the ==
operator works in Java:
public class StringComparison {
public static void main(String[] args) {
String str1 = "Java";
String str2 = "Java";
String str3 = new String("Java");
boolean result1 = (str1 == str2);
boolean result2 = (str1 == str3);
System.out.println("str1 == str2: " + result1);
System.out.println("str1 == str3: " + result2);
}
}
Output:
str1 == str2: true
str1 == str3: false
In this case, result1
is true
because str1
and str2
refer to the same string literal in the string pool. However, result2
is false
because str3
is created using the new
keyword, which allocates a new memory location. Thus, even though the content is the same, the references are different.
When to Use Each Method
Understanding when to use string.equals()
versus ==
is crucial for effective Java programming. If your goal is to compare the actual content of strings, always opt for string.equals()
. This method is reliable and ensures that you are checking for equality based on the string’s characters.
On the other hand, use the ==
operator when you need to check if two references point to the same object. This is less common in string comparisons but can be useful in certain situations, such as when you want to verify if two variables are actually the same instance.
In summary, for string content comparison, string.equals()
is your go-to method. For reference comparison, use ==
. Misunderstanding the difference between these two can lead to subtle bugs in your code, so it’s essential to choose wisely based on your needs.
Conclusion
In conclusion, the comparison between string.equals()
and ==
in Java is a fundamental concept that every Java developer should grasp. While string.equals()
focuses on the content of strings, the ==
operator checks for reference equality. By understanding these differences, you can avoid common pitfalls and write more robust Java applications. Remember, when in doubt, prefer string.equals()
for content comparison to ensure accurate results in your string operations.
FAQ
-
What is the main difference between string.equals() and == in Java?
string.equals() compares the content of two strings, while == compares their memory addresses. -
Is string.equals() case-sensitive?
Yes, string.equals() is case-sensitive. “Hello” and “hello” are not considered equal. -
Can I use == to compare string content?
It’s not recommended, as == checks for reference equality, which may lead to incorrect results. -
When should I use string.equals() instead of ==?
Use string.equals() when you want to compare the actual content of two strings. -
Are string literals treated differently than string objects?
Yes, string literals can be interned in the string pool, while string objects created with new are stored in different memory locations.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn