How to Copy a String in Java
- Copy a String in Java by Assigning One String to Another
-
Copy a String in Java Using the
copyValueOf
Method - Copy a String in Java Using the String Constructor
-
Copy a String in Java Using the
valueOf
Method -
Copy a String in Java Using the
Arrays.copyOf
Method -
Copy a String in Java Using the
concat
Method -
Copy a String in Java Using the
substring
Method -
Copy a String in Java Using
StringBuilder
orStringBuffer
- Conclusion
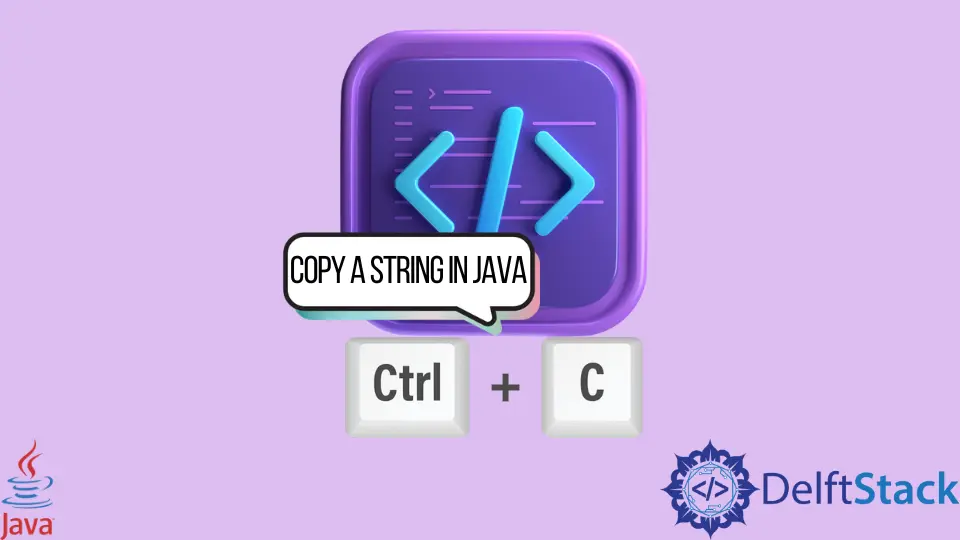
In Java, copying a string is a common operation that developers encounter frequently. Strings in Java are immutable, meaning their values cannot be changed once they are assigned.
Therefore, when you want to create a copy of a string, you need to consider different methods that align with your specific use case. In this article, we will explore various methods to copy a string in Java, each offering unique advantages and use cases.
Copy a String in Java by Assigning One String to Another
In Java, copying a String can be achieved through a straightforward assignment of one string variable to another. This approach relies on the immutability of strings, meaning that once a string is created, its value cannot be changed.
When one string variable is assigned to another in Java, what actually happens is that the reference to the string object is passed. Since strings are immutable, any operation that appears to modify a string actually creates a new string.
Therefore, when we assign one string to another, both variables will refer to the same string object. This creates the illusion of copying the string, but it’s essential to understand that both variables point to the same underlying immutable string.
Code Example:
public class StringCopyAssignmentExample {
public static void main(String[] args) {
String original = "Java Assignment";
String copy = original;
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
In the provided code snippet, we begin by declaring an original String variable containing the text Java Assignment
. The process of copying the string involves a simple assignment where the value of the original
string is assigned to another variable named copy
.
As mentioned earlier, both original
and copy
now reference the same underlying string object.
Output:
Original String: Java Assignment
Copied String: Java Assignment
The output confirms that the copy
String has successfully captured the content of the original
String by simply assigning one string to another. This method is easy to implement, but it’s crucial to be mindful of the immutability of strings and the shared reference to the same string object when using this approach.
Copy a String in Java Using the copyValueOf
Method
Java provides the copyValueOf
method as another option for copying a String. This method is part of the String
class and allows us to create a new String by copying the characters of an existing char array or another CharSequence
.
The copyValueOf
method in Java can be invoked on the String
class and takes a char array or another CharSequence
as its argument. It creates a new String containing the characters of the specified array or CharSequence
.
In the context of copying a String, we can use copyValueOf
to create a new String with the same content as the original one.
Code Example:
public class StringCopyValueOfExample {
public static void main(String[] args) {
String original = "Programming in Java";
String copy = String.copyValueOf(original.toCharArray());
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
Here, we begin with the declaration of an original String variable containing the text Programming in Java
. The copyValueOf
method is then applied by invoking it on the String
class and passing the result of original.toCharArray()
as its argument.
This creates a new String named copy
, effectively duplicating the content of the original string.
Output:
Original String: Programming in Java
Copied String: Programming in Java
This method provides a concise and effective approach for creating string copies in Java, particularly when dealing with char arrays or other CharSequence
instances.
Copy a String in Java Using the String Constructor
Another straightforward approach to copying a String in Java is to use the String
constructor. The String
constructor allows you to create a new String object by providing an existing string as its argument.
When you pass another String to this constructor, it effectively creates a new copy of the original string. This is possible because strings in Java are immutable.
The String
constructor exploits this immutability, producing a new instance with the same content as the original.
Code Example:
public class StringCopyExample {
public static void main(String[] args) {
String original = "Hello, World!";
String copy = new String(original);
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
In the code above, we first declare an original String variable containing the text Hello, World!
. Then, we use the String
constructor to create a new String called copy
and pass the original
String as its argument, which creates a separate copy of the original string.
Output:
Original String: Hello, World!
Copied String: Hello, World!
In this output, you can observe that the copy
String has successfully duplicated the content of the original
String using the String
constructor.
Copy a String in Java Using the valueOf
Method
The valueOf
method serves as a versatile tool, not only for converting other data types into strings but also for creating copies of existing strings. This method, part of the String
class, takes an argument and produces a new string representation of that argument.
When applied to a String
object, the valueOf
method effectively creates a new string with the same content as the specified object. This makes it a concise and efficient means of copying strings.
In this context, by invoking String.valueOf(original)
, we obtain a new string (copy
) that duplicates the content of the original string. It’s important to note that this method is not exclusive to strings; it can be used with a variety of data types.
Code Example:
public class StringCopyValueOfExample {
public static void main(String[] args) {
String original = "Java Essentials";
String copy = String.valueOf(original);
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
In this code, we initiate the process by declaring an original String variable containing the text Java Essentials
. The valueOf
method is then employed by invoking it on the String
class and passing the original
String as its argument.
This results in the creation of a new String named copy
, which effectively duplicates the content of the original string.
Output:
Original String: Java Essentials
Copied String: Java Essentials
The copy
String successfully captures content from the original
String using the valueOf
method.
Copy a String in Java Using the Arrays.copyOf
Method
Java also offers the Arrays.copyOf
method as an effective means to copy arrays, including character arrays representing strings.
The Arrays.copyOf
method is part of the java.util
package and is used to create a new array with a specified length. When applied to a character array representing a string, it allows us to copy a portion or the entire array, effectively creating a copy of the original string.
This method is particularly useful when combined with the toCharArray
method of the String
class to obtain the character array representation of the string.
Code Example:
import java.util.Arrays;
public class StringCopyArraysCopyOfExample {
public static void main(String[] args) {
String original = "Java Arrays";
char[] charArray = original.toCharArray();
String copy = new String(Arrays.copyOf(charArray, charArray.length));
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
In the provided code, we have the original String variable containing the text Java Arrays
. We then use the toCharArray
method on the original
String to obtain its character array representation.
The Arrays.copyOf
method is then applied to create a copy of the character array with the same length. Finally, a new String named copy
is created by passing the copied character array to the String
constructor.
Output:
Original String: Java Arrays
Copied String: Java Arrays
The output above confirms that the copy
String has successfully captured the content of the original
String using the Arrays.copyOf
method. This method provides an efficient approach for copying strings in Java, allowing for customization of the copied portion by adjusting the length parameter.
Copy a String in Java Using the concat
Method
Another method to copy a String in Java is by utilizing the concat
method. This approach involves concatenating an empty string with the original string, effectively creating a copy.
The concat
method is a member of the String
class in Java. When invoked, it concatenates the specified string to the end of the invoking string and returns a new string.
In this case, by concatenating an empty string (""
) with the original string, we obtain a new string that is essentially a copy of the original.
Code Example:
public class StringCopyConcatExample {
public static void main(String[] args) {
String original = "Java Programming";
String copy = "".concat(original);
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
Here, we begin with the declaration of an original String variable containing the text Java Programming
. Subsequently, we utilize the concat
method by invoking it on an empty string (""
) and passing the original
String as its argument.
This code creates a new String called copy
, which duplicates the content of the original string.
Output:
Original String: Java Programming
Copied String: Java Programming
Copy a String in Java Using the substring
Method
In Java, the substring
method offers yet another way to create a copy of a String. This method in Java is used to extract a part of a string.
By invoking this method without specifying any indices, we effectively obtain a substring that starts at the beginning of the original string and extends to the end.
In this context, when we apply substring(0)
to the original string, it creates a new string that is essentially a copy of the original. This happens because strings in Java are immutable, and the substring
method takes advantage of this immutability by producing a new string with the same content.
Code Example:
public class StringCopySubstringExample {
public static void main(String[] args) {
String original = "Programming in Java";
String copy = original.substring(0);
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
In the code snippet, we initiate the process with the declaration of an original String variable containing the text Programming in Java
. Subsequently, we utilize the substring
method by invoking it on the original
String without specifying any indices.
This results in the creation of a new String named copy
, effectively duplicating the entire content of the original string.
Output:
Original String: Programming in Java
Copied String: Programming in Java
The output above demonstrates that the copy
String has successfully captured the content of the original
String using the substring
method.
Copy a String in Java Using StringBuilder
or StringBuffer
In Java, when efficient manipulation of strings is required, the StringBuilder
and StringBuffer
classes are helpful tools. Unlike the previously discussed methods, these classes provide mutable sequences of characters, allowing for more dynamic string operations.
Both the StringBuilder
and StringBuffer
classes provide a toString
method that can be used to create a copy of the content. These classes allow for in-place modifications of the character sequence, making them more efficient for scenarios involving frequent string manipulations.
By initializing a StringBuilder
or StringBuffer
with the original string and converting it back to a string using the toString
method, we effectively create a copy.
Code Example:
public class StringCopyStringBuilderExample {
public static void main(String[] args) {
String original = "Java Programming";
StringBuilder stringBuilder = new StringBuilder(original);
String copy = stringBuilder.toString();
System.out.println("Original String: " + original);
System.out.println("Copied String: " + copy);
}
}
We first declare an original String variable containing the text Java Programming
. A StringBuilder
is then initialized with the original
String as its argument.
The toString
method is subsequently applied to the StringBuilder
instance, creating a new String named copy
that effectively duplicates the content of the original string.
Output:
Original String: Java Programming
Copied String: Java Programming
Conclusion
In this article, we explored several methods, including using straightforward assignment of one string to another, the String
constructor, concat
method, substring
method, valueOf
method, StringBuilder
or StringBuffer
, copyValueOf
method, and Arrays.copyOf
method. Each of these methods has its unique characteristics, leveraging the immutability of strings or providing mutable alternatives for efficient string manipulations.
The choice of a particular method depends on the specific requirements of the task at hand. If simplicity and readability are prioritized, using the String
constructor or the concat
method might be suitable.
For scenarios where mutable sequences are beneficial, StringBuilder
or StringBuffer
can be powerful tools. The copyValueOf
and Arrays.copyOf
methods provide flexibility when dealing with character arrays.
Choose the most appropriate technique based on the specific needs of your projects.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn