How to Compress Strings in Java
Sheeraz Gul
Feb 02, 2024
Java
Java String
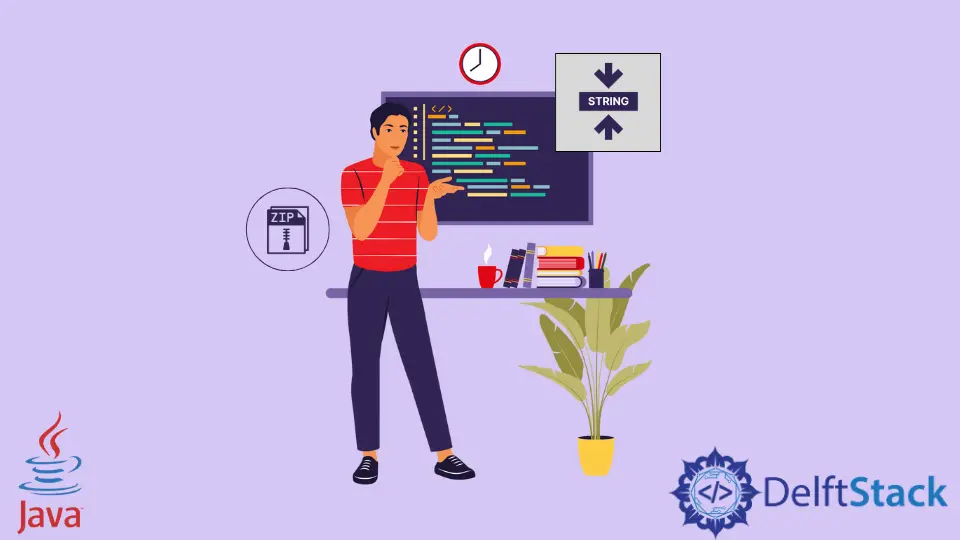
Compressing the string is required when we want to save the memory. In Java, the deflater
is used to compress strings in bytes.
This tutorial will demonstrate how to compress strings in Java.
Use deflater
to Compress Strings in Java
The deflater
is an object creator in Java that compresses the input data and fills the specified buffer with compressed data.
Example:
import java.io.UnsupportedEncodingException;
import java.util.zip.*;
class Compress_Class {
public static void main(String args[]) throws UnsupportedEncodingException {
// Using the deflater object
Deflater new_deflater = new Deflater();
String Original_string = "This is Delftstack ", repeated_string = "";
// Generate a repeated string
for (int i = 0; i < 5; i++) {
repeated_string += Original_string;
}
// Convert the repeated string into bytes to set the input for the deflator
new_deflater.setInput(repeated_string.getBytes("UTF-8"));
new_deflater.finish();
// Generating the output in bytes
byte compressed_string[] = new byte[1024];
// Storing the compressed string data in compressed_string. the size for compressed string will
// be 13
int compressed_size = new_deflater.deflate(compressed_string, 5, 15, Deflater.FULL_FLUSH);
// The compressed String
System.out.println("The Compressed String Output: " + new String(compressed_string)
+ "\n Size: " + compressed_size);
// The Original String
System.out.println("The Original Repeated String: " + repeated_string
+ "\n Size: " + repeated_string.length());
new_deflater.end();
}
}
The above code uses deflater
to set the input of the string converted to bytes and compress it.
Output:
The Compressed String Output: xœ
ÉÈ,V
Size: 15
The Original Repeated String: This is Delftstack This is Delftstack This is Delftstack This is Delftstack This is Delftstack
Size: 95
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook