Java Right Shift - >>>
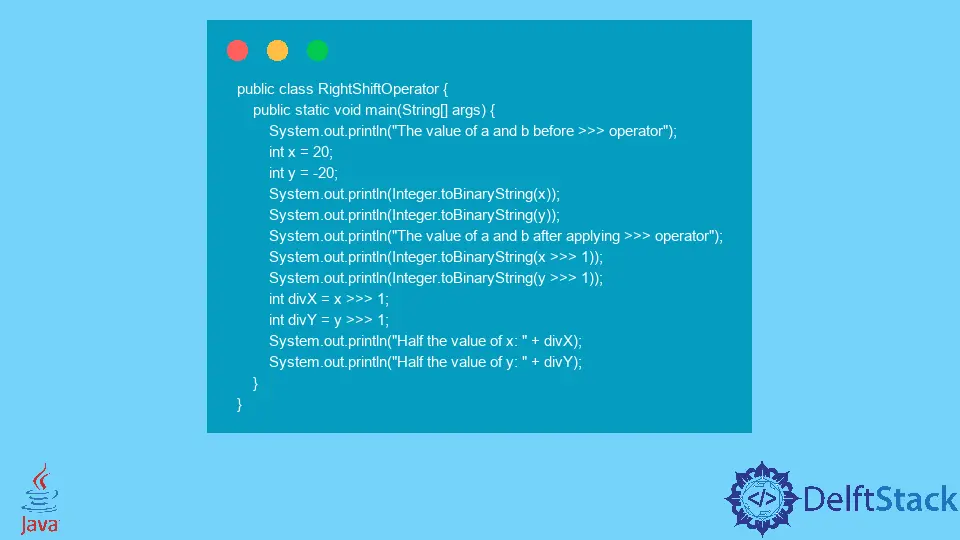
In Java language, >>>
is often known as the unsigned right bitshift operator. Unlike signed operators, it always allows the trailing places to get filled with zero value. Let us understand the following operations with the help of an example.
Consider two numbers that are a and b. The value of the given two is below.
a = 20
b = -20
a = 00000000000000000000000000010100
b = 11111111111111111111111111101100
The use-case of the bitwise right shift operator is value division or variable by 2.
Now let us apply unsigned right shift operator, that is, a>>>1
. The operator internally shifts all bits of the variable towards the right side. It fills the trailing positions with the zero value.
Below is the code block to understand the same.
public class RightShiftOperator {
public static void main(String[] args) {
System.out.println("The value of a and b before >>> operator");
int x = 20;
int y = -20;
System.out.println(Integer.toBinaryString(x));
System.out.println(Integer.toBinaryString(y));
System.out.println("The value of a and b after applying >>> operator");
System.out.println(Integer.toBinaryString(x >>> 1));
System.out.println(Integer.toBinaryString(y >>> 1));
int divX = x >>> 1;
int divY = y >>> 1;
System.out.println("Half the value of x: " + divX);
System.out.println("Half the value of y: " + divY);
}
}
In the above code block, the variables a
and b
gets initialized with value 20 and -20 each.
The toBinaryString()
function of the Integer
class gets applied in the print stream method.
The function of it is to convert the integer variable to convert to binary String. The method is available in the Java 1.2
version.
The Integer
class has functions to convert the primary int
value into the respective wrapper objects and hence acts as a wrapper to primitive values.
The input of the method is an int variable that is to get converted to the String value.
The parameter that gets passed in the function is the variable along with the operator.
Lastly, the variable with the operation performed gets printed.
The output of the code using the >>>
operator is as below.
The value of a and b before >>> operator
00000000000000000000000000010100
11111111111111111111111111101100
The value of a and b after applying >>> operator
00000000000000000000000000001010
01111111111111111111111111110110
Half the value of x: 10
Half the value of y: 2147483638
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn