The >> Operator in Java
- Understanding the » Operator
- Practical Examples of the » Operator
- Use Cases for the » Operator
- Conclusion
- FAQ
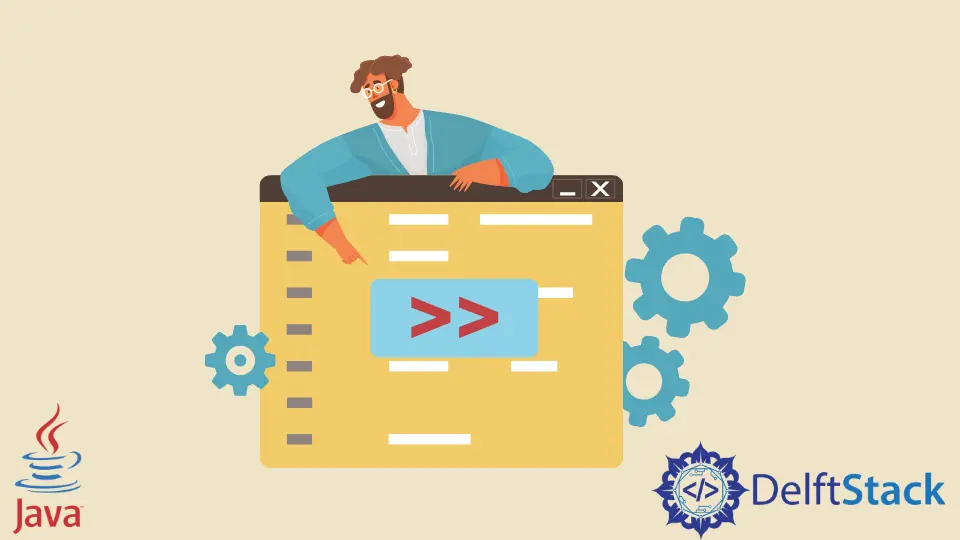
Java is a versatile programming language that offers various operators to manipulate data effectively. One of these operators is the “»” operator, known as the right shift operator.
This article will delve into the intricacies of the » operator in Java, exploring its functionality, use cases, and practical examples. Whether you are a beginner looking to understand Java better or an experienced developer wanting to refine your skills, this guide will provide you with valuable insights. So, let’s dive in and explore how the » operator can enhance your Java programming experience.
Understanding the » Operator
The right shift operator (») in Java is a bitwise operator that shifts the bits of a number to the right by a specified number of positions. This means that it effectively divides the number by 2 for each position it shifts. For example, if we have a binary number and we apply the right shift operator, the bits on the right end will be discarded, and zeros will be filled in from the left.
The syntax for using the right shift operator is straightforward:
int result = value >> numberOfPositions;
Here, value
is the integer you want to shift, and numberOfPositions
indicates how many bits you want to shift to the right. It’s important to note that the » operator works with both positive and negative integers, but the behavior differs slightly for negative numbers due to the way they are represented in binary (two’s complement form).
For instance, if you have the integer value 8 (which is 1000
in binary), shifting it right by one position would yield 4 (0100
in binary). Conversely, shifting a negative number will fill the leftmost bits with 1s, preserving the sign of the number.
Practical Examples of the » Operator
Let’s explore some practical examples to see how the » operator works in Java.
Example 1: Basic Right Shift Operation
Here’s a simple example demonstrating the right shift operation on a positive integer:
public class RightShiftExample {
public static void main(String[] args) {
int value = 16; // 10000 in binary
int result = value >> 2; // Shift right by 2 positions
System.out.println("Result of right shifting 16 by 2: " + result);
}
}
Output:
Result of right shifting 16 by 2: 4
In this example, we start with the integer 16, which is represented as 10000
in binary. By shifting it right by 2 positions, we discard the two rightmost bits, resulting in 0100
, which is equivalent to 4 in decimal.
Example 2: Right Shift with Negative Numbers
Now let’s see how the right shift operator behaves with negative integers:
public class RightShiftNegativeExample {
public static void main(String[] args) {
int value = -8; // 11111000 in binary (two's complement)
int result = value >> 2; // Shift right by 2 positions
System.out.println("Result of right shifting -8 by 2: " + result);
}
}
Output:
Result of right shifting -8 by 2: -2
In this case, the integer -8 is represented as 11111000
in binary (in two’s complement form). When we shift it right by 2 positions, the result is 11111110
, which corresponds to -2 in decimal. Notice how the leftmost bits are filled with 1s, preserving the negative sign.
Use Cases for the » Operator
The right shift operator is commonly used in various programming scenarios. Here are some practical use cases:
-
Performance Optimization: Right shifting can be more efficient than division by powers of two. For example, instead of dividing a number by 4, you can simply shift it right by two positions. This can be particularly useful in performance-critical applications where speed is of the essence.
-
Bit Manipulation: The » operator is essential for bit manipulation tasks, such as encoding and decoding data, cryptographic algorithms, and working with low-level hardware interfaces. Understanding how to manipulate bits can give you a significant advantage in many programming situations.
-
Data Compression: In certain algorithms, right shifting can help compress data by reducing the number of bits used to represent a value. This can be beneficial in applications where memory usage is a concern.
By understanding and utilizing the » operator effectively, you can enhance your Java programming skills and improve the performance and efficiency of your applications.
Conclusion
In conclusion, the right shift operator (») in Java is a powerful tool for manipulating integer values at the bit level. By shifting bits to the right, you can effectively divide numbers, perform bit manipulation, and optimize performance in your applications. Whether you are working with positive or negative integers, understanding how this operator works can help you write more efficient and effective Java code. With the examples provided in this article, you should now have a solid grasp of how to use the » operator in various scenarios. Happy coding!
FAQ
- What does the » operator do in Java?
The » operator shifts the bits of an integer to the right by a specified number of positions, effectively dividing the number by 2 for each position shifted.
-
How does the » operator work with negative numbers?
When applied to negative numbers, the » operator fills the leftmost bits with 1s, preserving the sign of the number. -
Can I use the » operator for performance optimization?
Yes, using the » operator can be more efficient than division when dividing by powers of two, making it useful in performance-critical applications. -
What is the difference between » and »> in Java?
The » operator preserves the sign of the number, while the »> operator (unsigned right shift) fills the leftmost bits with zeros, regardless of the sign. -
Are there any common use cases for the » operator?
Common use cases include bit manipulation, performance optimization, and data compression.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn