How to Replace Multiple Characters in String in Java
-
Replace Multiple Characters in a String Using
replaceAll()
in Java -
Replace Multiple Characters in a String Using
String.replace()
in Java -
Replace Multiple Characters in a String Using
String.replaceFirst()
in Java - Conclusion
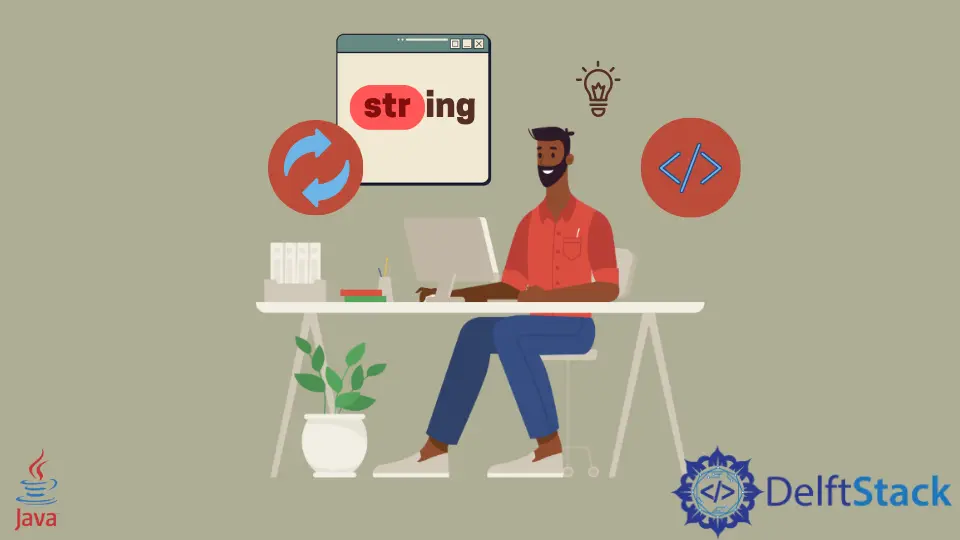
In Java, String.replaceAll()
, String.replace()
, and String.replaceFirst()
are all valuable methods for replacing characters within a string.
This article explores how we can employ these methods to replace multiple characters in a string. While replaceAll()
is capable of accomplishing this task using regular expressions, for those who prefer to avoid regular expressions, the replace()
and the replaceFirst()
methods are suitable alternatives.
Replace Multiple Characters in a String Using replaceAll()
in Java
The replaceAll()
method in Java is used to replace all occurrences of a specified character or a regular expression with another character or string.
Its syntax is as follows:
public String replaceAll(String regex, String replacement)
Parameters:
regex
: A regular expression pattern to match the characters or substrings you want to replace.replacement
: The string to replace the matched characters or substrings.
In the code example below, we have a class named ReplaceAllChars
containing a main
method. Inside this method, we have several strings containing different character patterns that we want to replace.
We then use the replaceAll()
method with regular expressions to achieve this.
Example:
public class ReplaceAllChars {
public static void main(String[] args) {
String stringUnderscoresForward = "j_u_s_t_a_s/t/r/i/n/g";
String stringWithDigits = "abcd12345efgh";
String stringWithWhiteSpaces = "s t r i n g";
String stringWithLowerCase = "This is a Lower Case String";
String finalString1 = stringUnderscoresForward.replaceAll("[_/]", "-");
String finalString2 = stringWithDigits.replaceAll("[\\d]", "");
String finalString3 = stringWithWhiteSpaces.replaceAll("[ ]", "");
String finalString4 = stringWithWhiteSpaces.replaceAll("[\\s]", "-");
String finalString5 = stringWithLowerCase.replaceAll("[\\p{Lower}]", "");
System.out.println("Old String: " + stringUnderscoresForward + " New String: " + finalString1);
System.out.println("Old String: " + stringWithDigits + " New String: " + finalString2);
System.out.println("Old String: " + stringWithWhiteSpaces + " New String: " + finalString3);
System.out.println("Old String: " + stringWithLowerCase + " New String: " + finalString4);
System.out.println("Old String: " + stringWithLowerCase + " New String: " + finalString5);
}
}
Output:
Old String: j_u_s_t_a_s/t/r/i/n/g --New String: j-u-s-t-a-s-t-r-i-n-g
Old String: abcd12345efgh --New String: abcdefgh
Old String: s t r i n g --New String: string
Old String: This is a Lower Case String --New String: s-t-r-i-n-g
Old String: This is a Lower Case String --New String: T L C S
Let’s break down each part of the code and understand how it works.
In the line shown below, we’re replacing underscores and forward slashes in the stringUnderscoresForward
with hyphens ('-'
). The regular expression "[_/]"
matches either an underscore or a forward slash.
String finalString1 = stringUnderscoresForward.replaceAll("[_/]", "-");
Here, we’re removing all digits from the stringWithDigits
. The regular expression "[\\d]"
matches any digit (0-9), and we replace it with an empty string to remove them.
String finalString2 = stringWithDigits.replaceAll("[\\d]", "");
In this line, we’re removing white spaces from the stringWithWhiteSpaces
. The regular expression "[ ]"
matches a single space character, and we replace it with an empty string to remove spaces.
String finalString3 = stringWithWhiteSpaces.replaceAll("[ ]", "");
This line replaces white spaces in stringWithWhiteSpaces
with hyphens ('-'
). The regular expression "[\\s]"
matches any whitespace character (space, tab, newline), and we replace it with a hyphen.
String finalString4 = stringWithWhiteSpaces.replaceAll("[\\s]", "-");
In this line, we’re converting all lowercase characters in stringWithLowerCase
to hyphens ('-'
). The regular expression "[\\p{Lower}]"
matches any lowercase character, and we replace them with an empty string to remove them.
String finalString5 = stringWithLowerCase.replaceAll("[\\p{Lower}]", "");
Replace Multiple Characters in a String Using String.replace()
in Java
The replace()
method in Java is a member of the String
class and is used to replace all occurrences of a specified character or substring within a string with another character or substring. The syntax for the replace()
method is as follows:
public String replace(CharSequence target, CharSequence replacement)
Parameters:
target
: The character or sequence of characters to be replaced.replacement
: The character or sequence of characters to replace the occurrences oftarget
.
This method returns a new string with the specified replacements. It does not modify the original string; instead, it creates a new string with the replacements.
If target
is not found in the string, the original string is returned without any changes.
Let’s analyze the code example below.
Example:
public class ReplaceAllChars {
public static void main(String[] args) {
String stringWithRandomChars = "javbjavcjadakavajavc";
String finalString =
stringWithRandomChars.replace("b", "a").replace("c", "a").replace("d", "v").replace(
"k", "j");
System.out.println(finalString);
}
}
Here, we first create a string that contains the characters we want to modify. We then chain multiple replace()
method calls on the string, each replacing one character with another.
In the provided code example, we have the following replacements:
First replace()
Call:
stringWithRandomChars.replace("b", "a")
This replaces all occurrences of "b"
with "a"
in the stringWithRandomChars
.
Second replace()
Call:
.replace("c", "a")
This replaces all occurrences of "c"
(resulting from the previous replacement) with "a"
.
Third replace()
Call:
.replace("d", "v")
This replaces all occurrences of "d"
(resulting from the previous replacement) with "v"
.
Fourth replace()
Call:
.replace("k", "j");
This replaces all occurrences of "k"
(resulting from the previous replacement) with "j"
.
Output:
javajavajavajavajava
Replace Multiple Characters in a String Using String.replaceFirst()
in Java
The replaceFirst()
method is also a member of the String
class in Java. It takes two parameters: the substring you want to replace and the substring you want to replace it with.
The method replaces only the first occurrence of the specified substring. If the substring is not found, the original string remains unchanged.
Here is the syntax of the replaceFirst()
method:
public String replaceFirst(String regex, String replacement)
Parameters:
regex
: The regular expression to be replaced.replacement
: The string that will replace the first occurrence of the regular expression.
Below is a complete code example.
Example:
import java.lang.String;
public class StringReplacementExample {
public static void main(String[] args) {
String originalString = "This is a sample string.";
originalString = originalString.replaceFirst("i", "1");
originalString = originalString.replaceFirst("s", "2");
System.out.println("Modified string: " + originalString);
}
}
Output:
Modified string: Th12 is a sample string.
First, we declare a String
object that contains the original string in which you want to replace characters.
String originalString = "This is a sample string.";
Now, to replace multiple characters, you’ll call the replaceFirst()
method multiple times. Each time, you’ll replace a specific character or substring.
originalString = originalString.replaceFirst("i", "1");
originalString = originalString.replaceFirst("s", "2");
Here, we’re replacing the first occurrence of 'i'
with '1'
and the first occurrence of 's'
with '2'
. Thus, when the println()
is called, the modified originalString
is shown as the output.
It is good to know the replaceFirst()
method so that you can utilize it in certain instances, but it is not advisable to use it in most cases due to its inefficiency.
Conclusion
The replaceAll()
method in Java is a valuable tool for replacing specific characters or substrings within a string. It utilizes regular expressions as patterns, enabling developers to easily replace multiple occurrences of characters and facilitating versatile string manipulation.
Another option for character or substring replacement is the replace()
method, offering flexibility in handling string transformations. Meanwhile, the replaceFirst()
method is one other way to replace multiple characters in a string, but it is not advisable to use since it is inefficient in most cases.
By comprehending these methods, we can enhance their string manipulation capabilities in Java.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn