Question Mark and Colon in Java
- Question Mark and Colon Operator
- Use Question Mark and Colon Operator in Java
- Use Nested Question Mark and Colon Operator in Java
- Conclusion
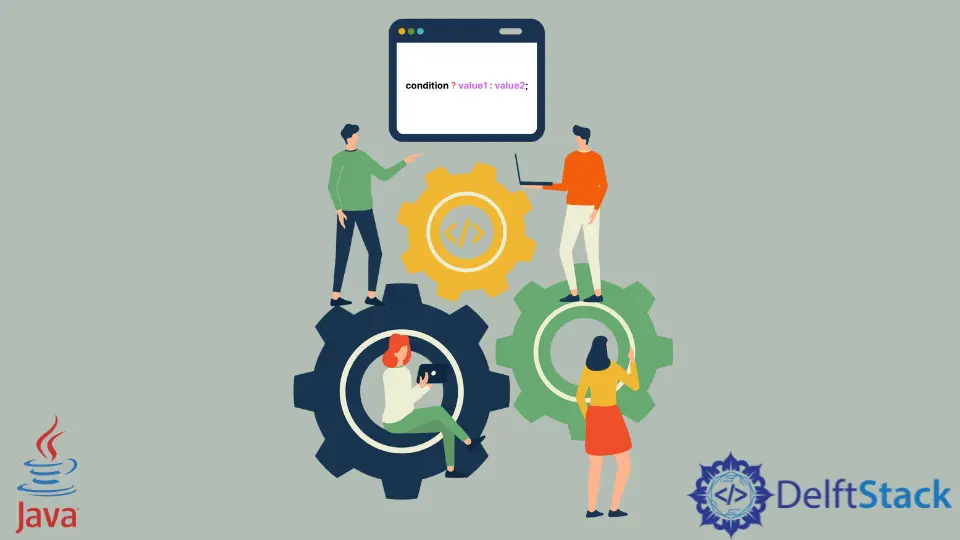
This article explores the versatile applications of the question mark (?
) and colon (:
) operators, collectively known as the ternary conditional operator, along with insights into their usage and the efficiency of nested ternary operators in Java programming.
Question Mark and Colon Operator
The question mark (?
) and colon (:
) operators in Java are collectively called the ternary conditional operator. They provide a concise way to express conditional logic, serving as a shorthand for an if-else
statement.
This helps in writing more compact and readable code by handling simple conditions in a single line. The ternary operator is particularly useful for assigning values or choosing expressions based on a condition, enhancing code efficiency and clarity.
Syntax:
result = (condition) ? expressionIfTrue : expressionIfFalse;
If the condition
is true, the expression before the colon is evaluated and assigned to the result
; otherwise, the expression after the colon is evaluated and assigned. It’s a concise way to make decisions based on a condition.
Usage | Description | Example |
---|---|---|
Conditional Assignment | Assigns a value based on a condition. | int x = (a > b) ? a : b; |
Return Statements |
Determines a value to be returned based on a condition. | int max = (num1 > num2) ? num1 : num2; |
Print Statements |
Prints different messages based on a condition. | System.out.println((age >= 18) ? "Adult" : "Minor"); |
Method Arguments | Passes different values to a method based on a condition. | displayMessage((status == 1) ? "Enabled" : "Disabled"); |
Nested Ternary Operators | Allows nesting of ternary operators for more complex conditions. | int result = (x > y) ? ((x > z) ? x : z) : ((y > z) ? y : z); |
The table above provides a clear and organized overview of the usage, description, and examples of the Java ternary conditional operator in different contexts.
Use Question Mark and Colon Operator in Java
In the realm of Java programming, mastering concise and effective ways to express conditional logic is a crucial skill. The question mark (?
) and colon (:
) operators, collectively known as the ternary conditional operator, offer a powerful tool for achieving just that.
Let’s dive straight into a practical example to grasp the versatility of the ternary conditional operator. Consider a scenario where we need to determine the eligibility of an individual based on their age.
public class TernaryOperatorExample {
public static void main(String[] args) {
int age = 22;
String eligibilityStatus = (age >= 18) ? "Adult" : "Minor";
System.out.println("The person is: " + eligibilityStatus);
}
}
In the above code snippet, we have a variable age
initialized with the value 22
. The critical line is:
String eligibilityStatus = (age >= 18) ? "Adult" : "Minor";
In this code snippet, the age of an individual is evaluated using the ternary conditional operator. The condition (age >= 18)
checks if the age is greater than or equal to 18.
If true, the expression before :
is executed; otherwise, the expression after :
is executed. The result of this operation is assigned to the variable eligibilityStatus
.
Finally, an output statement prints the person’s status, stating whether they are an adult or a minor. This concise approach to conditional logic enhances code readability, making it a valuable tool in Java programming.
The output confirms the individual’s status as an adult, demonstrating the effectiveness of the ternary conditional operator in simplifying decision-making processes.
In this example, the ternary conditional operator enables us to succinctly express a common decision-making process. The code is concise, easy to read, and efficiently conveys the logic of determining whether an individual is an adult or a minor.
Running the program would produce the following output:
The person is: Adult
Such concise and clear code is invaluable in real-world applications, making the ternary conditional operator a valuable tool in a Java programmer’s toolkit.
Use Nested Question Mark and Colon Operator in Java
In the vast landscape of Java programming, mastering the art of concise and expressive conditional statements is essential. The nested question mark (?
) and colon (:
) operators, embedded within each other, offer a fascinating approach to handling complex conditions.
Let’s unravel the intricacies of nested ternary operators through a practical example. Imagine a scenario where we want to determine the maximum of three numbers: x
, y
, and z
.
public class NestedTernaryExample {
public static void main(String[] args) {
int x = 15, y = 20, z = 10;
int result = (x > y) ? ((x > z) ? x : z) : ((y > z) ? y : z);
System.out.println("The maximum number is: " + result);
}
}
In this code, we’re using a nested ternary operator to find the maximum among x
, y
, and z
.
In this code, we determine the maximum of three numbers—x
, y
, and z
—using nested ternary operators. First, we check if x
is greater than y
.
If true, we evaluate whether x
is greater than z
using an inner ternary operator. The result of this inner operation is assigned if the outer condition is true.
If the outer condition is false, we move to another ternary operator, checking if y
is greater than z
. The result of this inner operation is assigned if the outer condition is false.
Ultimately, the variable result
is assigned the value from either the innermost true or false branch. The program concludes by printing the maximum number with a descriptive message, showcasing the efficiency of nested ternary operators in handling complex conditions.
When executed, the output confirms the correct identification of the maximum number among x
, y
, and z
, highlighting the utility of nested ternary operators in Java programming.
The use of nested ternary operators in this example demonstrates their ability to handle intricate conditions with conciseness. Although nested ternary operators should be used judiciously to maintain code readability, they provide a powerful tool when navigating through multiple decision branches.
Running the program would produce the following output:
The maximum number is: 20
This output confirms that the nested ternary operators correctly identified and returned the maximum number among the given variables x
, y
, and z
. The flexibility of nested ternary operators allows for elegant and efficient handling of complex decision-making scenarios.
Conclusion
The question mark (?
) and colon (:
) operators, collectively known as the ternary conditional operator, stand as powerful tools in Java programming. Their usage, demonstrated in scenarios like conditional assignments, return
statements, print
statements, and method arguments, provides a concise and readable means of expressing decision-making logic.
Furthermore, delving into nested ternary operators reveals their capability to handle complex conditions efficiently. While their application should be mindful of code readability, the versatility of these operators significantly contributes to crafting clear and expressive code in Java.