How to Do Power in Java
-
Raise a Number to a Power Using the
pow()
Method in Java -
Raise a Number to Power Using the
while
Loop in Java -
Raise a Number to Power Using the
for
Loop in Java -
Raise a Number to a Power Using the
recursion
in Java
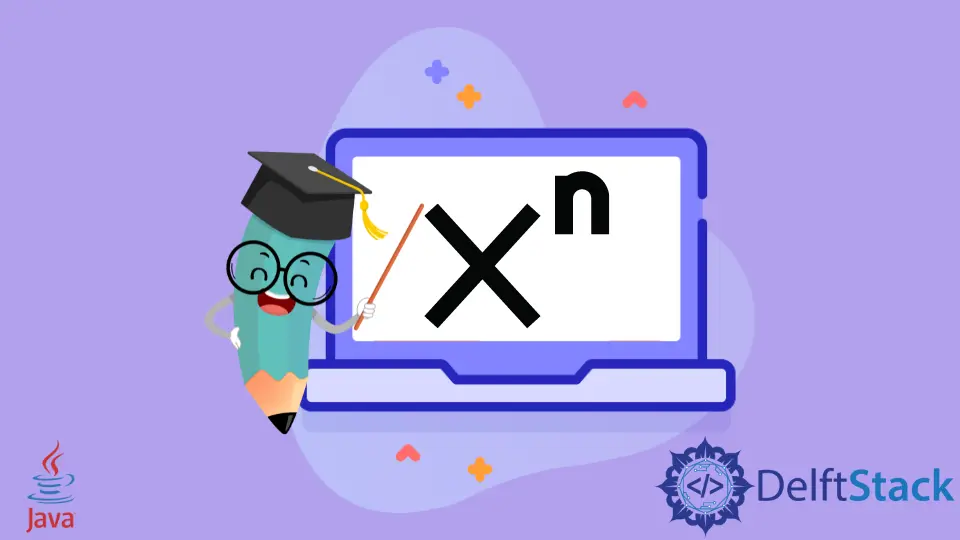
This tutorial introduces how to do power operation in Java and lists some example codes to understand the topic.
To raise a number to a power in Java, we can use the pow()
method of the Math
class or our own custom code that uses loop or recursion technique. Let’s see some examples.
Raise a Number to a Power Using the pow()
Method in Java
The pow()
method belongs to the Math
class in Java used to generate a number of the given power. It is a simple and straightforward approach because pow()
is a built-in method that reduces the effort to write custom code. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = Math.pow(a, power);
System.out.println(a + " power of " + power + " = " + result);
}
}
Output:
20.0 power of 2.0 = 400.0
Raise a Number to Power Using the while
Loop in Java
If you don’t want to use the built-in pow()
method, then use the custom code below. We use a while
loop inside this code to generate a number to a power. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = 1;
double temp = power;
while (temp != 0) {
result *= a;
--temp;
}
System.out.println(a + " power of " + power + " = " + result);
}
}
Output:
20.0 power of 2.0 = 400.0
Raise a Number to Power Using the for
Loop in Java
If you don’t want to use the built-in pow()
method, use the custom code below. We use the for
loop inside this code to generate a number to a power. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = 1;
double temp = power;
for (; temp != 0; --temp) {
result *= a;
}
System.out.println(a + " power of " + power + " = " + result);
}
}
Output:
20.0 power of 2.0 = 400.0
Raise a Number to a Power Using the recursion
in Java
This is another approach where we can use recursion
to raise a number to a power in Java. Recursion is a technique in which a function calls itself repeatedly until the base condition meets. Here, we create a recursion method, pow()
. See the example below.
public class SimpleTesting {
static double result = 1;
static void pow(double n, double p) {
if (p <= 0) {
return;
} else if (n == 0 && p >= 1) {
result = 0;
return;
} else
result = result * n;
pow(n, p - 1);
}
public static void main(String[] args) {
double a = 20;
double power = 2;
pow(a, power);
System.out.println(a + " power of " + power + " = " + result);
}
}
Output:
20.0 power of 2.0 = 400.0