How to Create an Ordered Map in Java
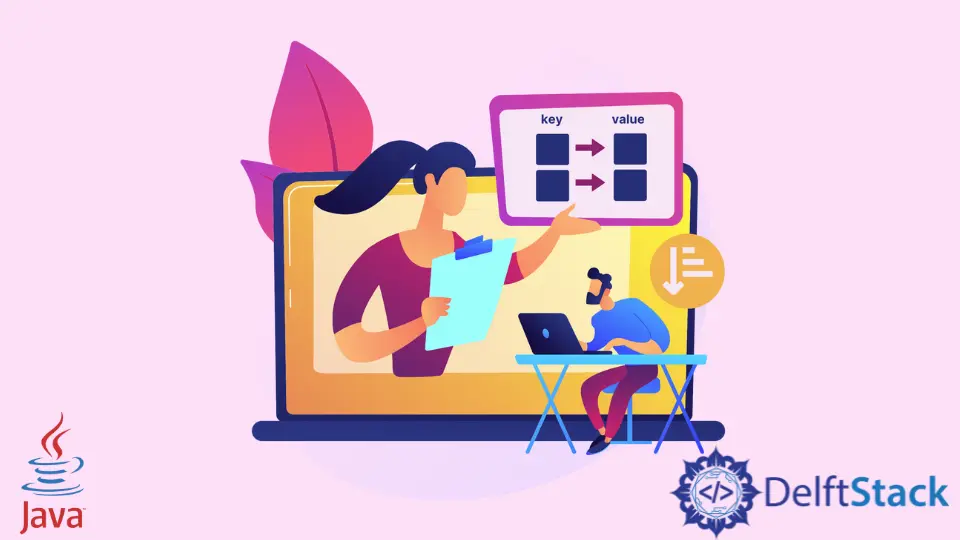
A map is a data structure in Java that stores key and value pairs. The map is an interface
present in the Collection
hierarchy. These keys are unique so, no duplicate keys are allowed; however, the variables mapped to the key can have duplicate values. Classes like HashMap
, LinkedHashMap
, and TreeMap
implement the Map
interface.
Sort the Map Using the TreeMap
Class in Java
Below, the program demonstrates the map ordering in the Java program.
import java.util.TreeMap;
public class Main {
public static void main(String[] args) {
TreeMap<String, Integer> map = new TreeMap<String, Integer>();
map.put("Third", 1);
map.put("First", 2);
map.put("Second", 3);
for (String key : map.keySet()) {
System.out.println(key + " ,ID = " + map.get(key));
}
}
}
The TreeMap
class sorts the map values in ascending order. It also implements the SortedMap
interface internally, so a map instance is created using a new keyword.
The datatype inside the treemap is specified at the time of instantiation. The Map
key is of the String
type, and its value is of the Integer
type.
The put
function inserts the key-value pairs in the treemap. Now, a for-each
loop gets defined to iterate over the map. In Java, direct iteration over the map is not possible. So, the keys of the map are initially converted to a Set
instance.
The map.keySet
function returns the Set
of keys present in the map. This function is in the TreeMap
class and returns the ordered view of the keys present. The get
function gets the value corresponding to the key.
Below is the output in ascending order.
First, ID = 2 Second, ID = 3 Third, ID = 1
Create a Map Ordering Using Java 8 Functions
Java 8 provides support for functional programming that allows users to work over the chain of functions.
Streams
is an interface in the java.util
package that provides an ease to work over the sequential operations in a single statement. The Streams
function works in the pipeline where an emitter emits data; it gets filtered, processed, transformed, and much more, depending on the users’ needs.
package F09;
import static java.util.AbstractMap.SimpleEntry;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class MapOrdering {
public static void main(String[] args) {
Map<String, String> mapSortedByKey =
Stream
.of(new SimpleEntry<>("key3", "value1"), new SimpleEntry<>("key1", "value2"),
new SimpleEntry<>("key2", "value3"))
.sorted(Map.Entry.comparingByKey())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue,
(oldVal, newValue) -> oldVal, LinkedHashMap::new));
System.out.println();
System.out.print("Ordered List: ");
for (String s1 : mapSortedByKey.keySet()) {
System.out.print(" " + s1);
}
}
}
The Stream
interface provides various functions and gets its implementation in different classes to work over them. Here, the stream of key-value pairs is formed using the new SimpleEntry
class. The values are inserted in the of
function to form a stream.
In the chain series, the sorted
function gets called. The function takes a Comparator
instance to arrange the keys in a sequence depending on the order defined. The function comparingByKey
returns the comparator that compares the key in natural ascending order.
The sorted
function finally returns a stream of values arranged in ascending order. The collect
function of the Stream
class collects the given map values in a new LinkedHashMap
instance. The class preserves the insertion order of the sequence provided. The function takes the Collector
instance as a parameter.
The first parameter is a supplier toMap
function that creates a new container. The second parameter is BiConsumer
that accumulates the value, and the last parameter is BiConsumer
that acts as a combiner that merges the results. So, the LinkedHashMap::new
command combines the result and returns the instance formed.
The mapSortedByKey
instance now holds the sequential map elements that get iterated using the for-each
loop above. The resultant map keys are printed in the standard output below.
Ordered List : key1 key2 key3
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java Map
- Increment Map in Java
- How to Convert Stream Element to Map in Java
- How to Convert Map Values Into a List in Java
- How to Convert List to Map in Java
- How to Filter A Value From Map in Java
- How to Create Map in Java