How to Sort a List Using stream.orted() in Java
-
Use Stream
sorted()
to Sort a List in Java -
Use Stream
sorted()
to Sort a List in Reverse Order in Java
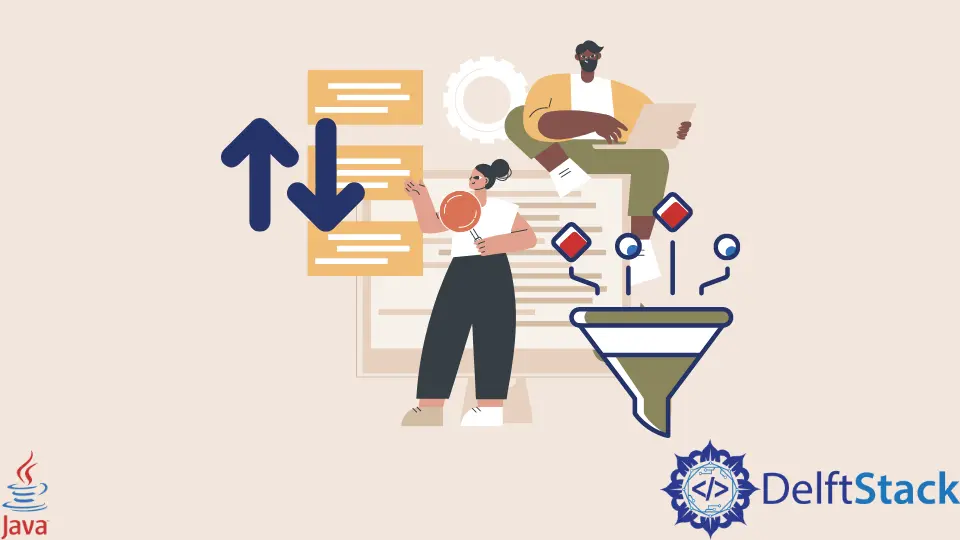
This tutorial will elaborate on the stream sorted()
method provided with the stream API in Java.
Use Stream sorted()
to Sort a List in Java
Java provides the stream API for easy processing of the objects of its collections. A stream takes input from input/output or lists to provide a result without modifying the original data structure.
Stream sorted()
returns a stream of elements, sorted in the natural order as per the input. This method is helpful for an ordered stream but gives an unstable result for the unordered streams, requiring further tweaking.
Syntax:
Stream<Interface> sorted()
Here is a simple example of code.
import java.util.*;
public class StreamSorting {
public static void main(String[] args) { // List of first 5 positive and even integers
List<Integer> MyList = Arrays.asList(10, 2, 6, 8, 4);
System.out.println("Stream Sorted returns: ");
// List to stream, sorting, and printing
MyList.stream().sorted().forEach(System.out::println);
}
}
Output:
Stream Sorted returns:
2
4
6
8
10
Use Stream sorted()
to Sort a List in Reverse Order in Java
We can also provide the order in which the sorting takes place. To get the output or sorted stream in reversed order, specify it inside the sorted
method by telling the comparator to use the reverse order.
Syntax:
.sorted(Comparator.reverseOrder())
After defining the class, specify how to compare the two objects of the class,
Syntax:
Stream<Interface> sorted(Comparator<? Super Interface> comparator)
Use the following syntax for printing a list after defining the comparator.
MyList.stream()
.sorted((obj1, obj2) -> obj1.getItem().getValue().compareTo(obj2.getItem().getValue()))
.forEach(System.out::println);
Below is an example of a sorted stream in reverse order.
import java.util.*;
public class StreamCompareToExample {
// Main functions
public static void main(String[] args) {
// Creating list
List<coordinate> MyList = new ArrayList<>();
// Adding objects to list
MyList.add(new coordinate(20, 200));
MyList.add(new coordinate(30, 300));
MyList.add(new coordinate(10, 100));
MyList.add(new coordinate(40, 400));
// List to stream, sorting two points P1, P2
// a in P1 is compared to a of P2, sorted, and then printed
MyList.stream().sorted((p1, p2) -> p1.a.compareTo(p2.a)).forEach(System.out::println);
}
}
// A class of coordinate point objects
class coordinate {
Integer a, b;
coordinate(Integer a, Integer b) {
this.a = a;
this.b = b;
}
public String toString() {
return this.a + ", " + this.b;
}
}
Output:
10, 100
20, 200
30, 300
40, 400