How to Fix Java Numberformatexception for Input String Error
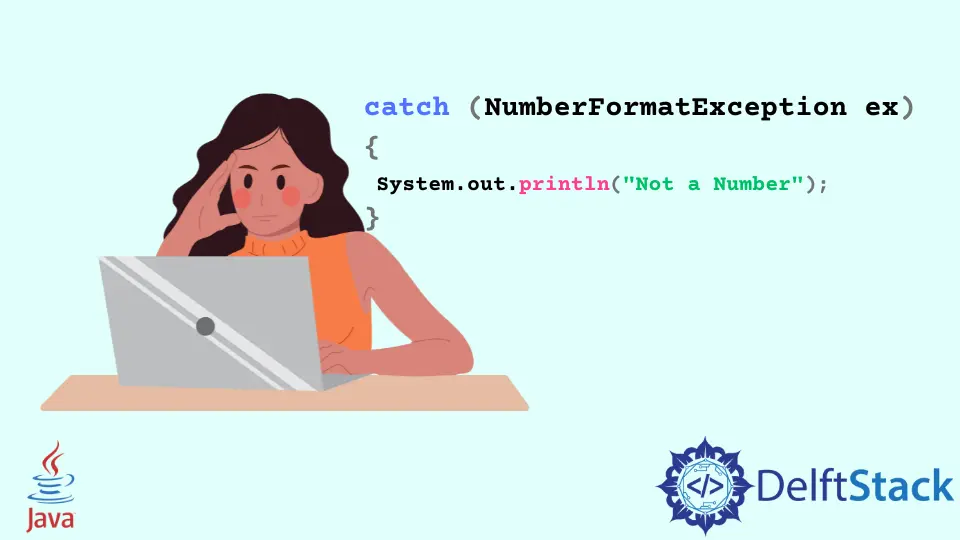
This guide will tell you how you can prevent numberformatexception
for input strings in Java. To understand it fully, we need to follow up on some of the basics of exception handling in Java. Let’s take a deeper look into it.
Exceptions in Java
The exception is a class that is used to handle some conditions. This class and its subclasses are a form of Throwable, indicating a certain condition that you need to catch while making applications.
Generally, you will see two types of exceptions. They are known as Checked Exception and Unchecked Exception.
Checked exceptions lie under the compile-time exceptions, while unchecked exceptions lie under RuntimeException
. A programmer can make their custom exception by extending from the exception class.
Learn more about the exception here.
Handling numberformatexception
for Input String in Java
In general, we handle the exception using the try...catch
method. The numberformatexception
for input string in Java is the same.
When sending a string as input and parsing it into an integer, it must throw a numberformatexception
. You can avoid the error by handing it in using the try...catch
methods.
Take a look at the following self-explanatory code.
import java.util.*;
public class Main {
public static void main(String args[]) {
String var = "N/A";
// When String is not an integer. It must throw NumberFormatException
// if you try to parse it to an integer.
// we can avoid from Exception by handling Exception.
// Exception Is usually Handle by try Catch Block.
try {
int i = Integer.parseInt(var);
// if var is not a number than this statement throw Exception
// and Catch Block will Run
System.out.println("Number");
} catch (NumberFormatException ex) { // handling exception
System.out.println(" Not A Number");
}
}
}
Output:
Not A Number
In the above code, you can see that parsing the var
string will not work. It’s a condition that we need to check.
So, using the try...catch
block, we handled it. If the string value is not a number, then the catch
block will run.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn