How to Handle OutOfMemoryError Exception in Java
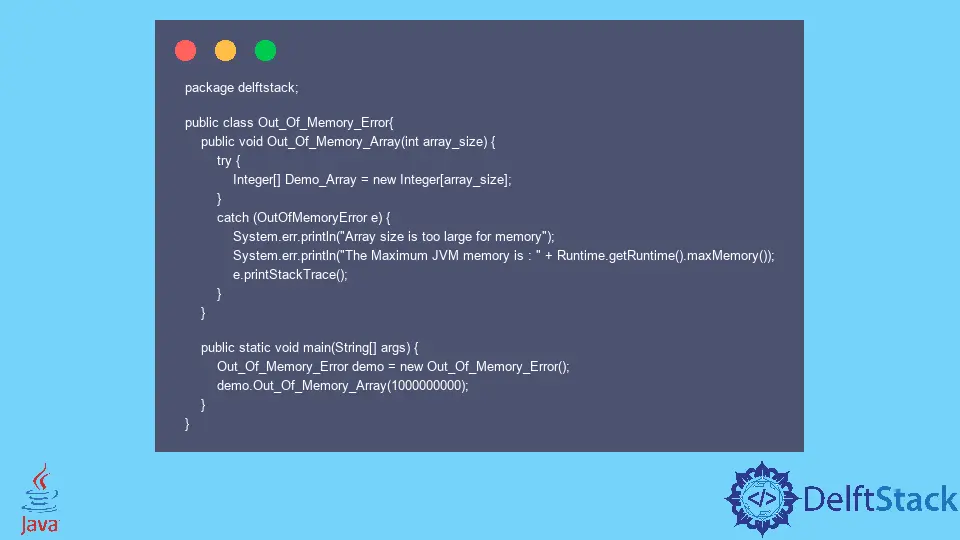
The JVM throws the OutOfMemoryError exception when it cannot allocate an object in the heap space. The heap space is used to store the object created at runtime.
There are two different parts of space in JVM, Permgen and Heap Space. This tutorial describes the OutOfMemoryError exception and demonstrates how to handle it.
The OutOfMemoryError Exception in Java
The Java Virtual Machine throws the OutOfMemoryError when it finds that insufficient memory is left to store the new object in heap space.
The OutOfMemoryError is thrown when the user is doing something wrong. For example, the user is trying to process high data by holding the objects for a long time.
It can also be an error when the user cannot handle a third-party library that catches strings or an uncleaned application server after deployments.
This error can also be thrown by native library code; for example, when swap space is low, the native allocation cannot be satisfied.
Example:
package delftstack;
public class Out_Of_Memory_Error {
public void Out_Of_Memory_Array(int array_size) {
try {
Integer[] Demo_Array = new Integer[array_size];
} catch (OutOfMemoryError e) {
System.err.println("Array size is too large for memory");
System.err.println("The Maximum JVM memory is : " + Runtime.getRuntime().maxMemory());
e.printStackTrace();
}
}
public static void main(String[] args) {
Out_Of_Memory_Error demo = new Out_Of_Memory_Error();
demo.Out_Of_Memory_Array(1000000000);
}
}
This code tries creating an array with a length of 1000000000, which is impossible for JVM because it goes out of the memory. The output will show the JVM memory and the error.
Output:
Array size is too large for memory
The Maximum JVM memory is : 1046478848
java.lang.OutOfMemoryError: Java heap space
at delftstack.Out_Of_Memory_Error.Out_Of_Memory_Array(Out_Of_Memory_Error.java:6)
at delftstack.Out_Of_Memory_Error.main(Out_Of_Memory_Error.java:17)
Handle the OutOfMemoryError Exception in Java
Once we know what causes the OutOfMemoryError, we can fix it so the JVM can store the objects in Java heap space. Let’s see the different solutions for different reasons of java.lang.OutOfMemoryError.
Use the -Xmx
Option to Handle OutOfMemoryError Exception in Java
The most common reason for OutOfMemoryError is the size of Java Virtual Machine heap space. The IDE can solve this problem using the -Xmx
option.
This configuration will increase the heap space up to 1024 size. However, increasing the heap size doesn’t guarantee solving all the errors, such as memory leaks errors.
An increase in the heap space size will also increase the length of GC. Let’s see how to solve the heap size problem.
As we are using Eclipse IDE, open the eclipse.ini
file from the installation folder:
Now, we can change the Xmx value as per our system requirement:
Now, relaunch the Eclipse to solve the error.
Use the Memory Analyzer Tool to Handle OutOfMemoryError Exception in Java
Increasing the heap size can generate other problems, and it doesn’t guarantee to solve the problem. The better way is to look where the error lies and solve it accordingly.
One other common reason is memory leakage, and if there is a memory leakage in the JVM, it can also throw the OutOfMemoryError. To check the memory leakage, we can use the IDE tools.
For example, Eclipse has Memory Analyzer Tool (MAT), which checks for memory leaks and reduces memory consumption. The MAT can be downloaded from here.
Using this tool, we can analyze the memory leak and solve the OutOfMemoryErorr error.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook