How to Fix java.lang.IllegalStateException in Java
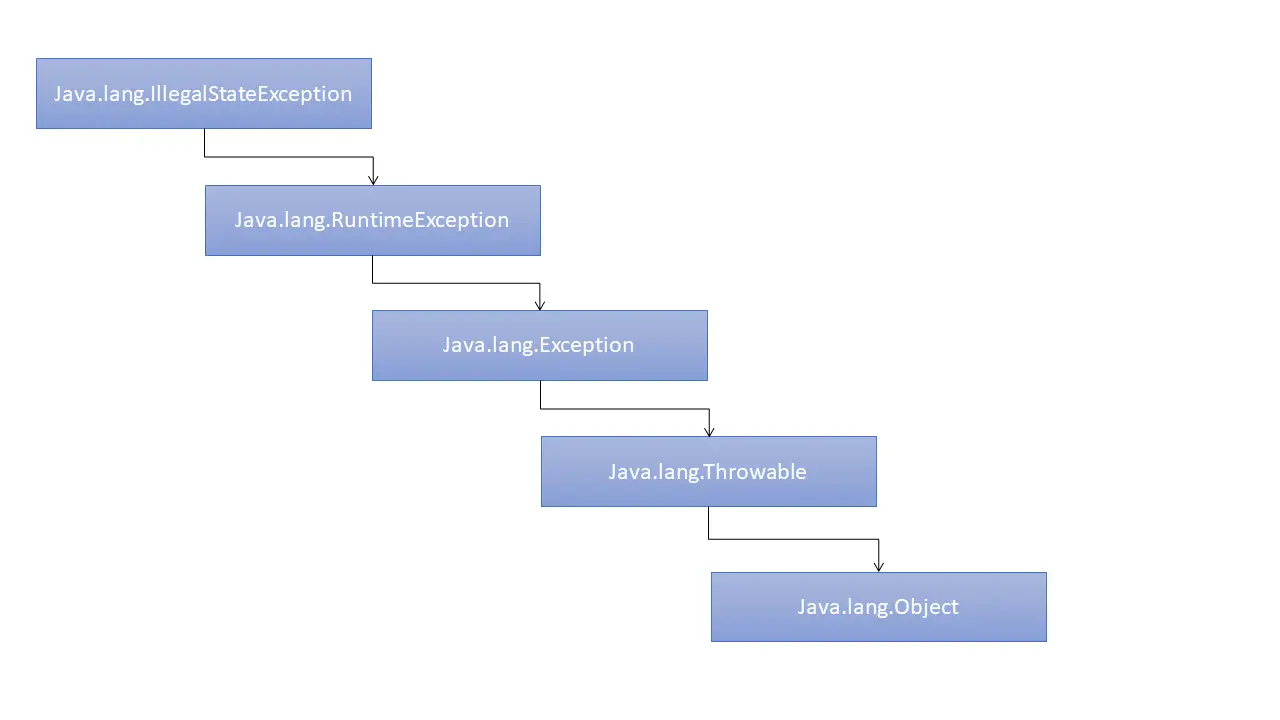
IllegalStateException
is an unchecked exception part of Java lang’s RuntimeException
class. IllegalStateException
is thrown when a called method is illegal or called at the wrong time.
This exception is set by the programmers or API developers. For example, when using an iterator, if we call the remove()
method before the next()
method, it will throw IllegalStateException
.
This tutorial demonstrates when the IllegalStateException
is thrown so that we can prevent it.
Demonstrate java.lang.IllegalStateException
in Java
The IllegalStateException
is usually thrown when the developer works with the List, Queue, Tree, Maps, Iterator, and the other Collections frameworks.
Mostly the list and queue are where the IllegalStateException
is thrown. The picture below shows the structure of IllegalStateException
.
Here is an example where the IllegalStateException
can be raised.
package delftstack;
import java.util.*;
public class Illegal_State_Exception {
public static void main(String args[]) {
List<String> Demo_List = new ArrayList<String>();
Demo_List.add("Delftstack1");
Demo_List.add("Delftstack2");
Demo_List.add("Delftstack3");
Demo_List.add("Delftstack4");
Demo_List.add("Delftstack5");
Iterator<String> Demo_Iter = Demo_List.iterator();
while (Demo_Iter.hasNext()) {
// System.out.print(Demo_Iter.next()+"\n");
// Calling remove() before next() will throw IllegalStateException
Demo_Iter.remove();
}
}
}
Calling the remove()
before next()
for iterator will throw an IllegalStateException
.
Output:
Exception in thread "main" java.lang.IllegalStateException
at java.base/java.util.ArrayList$Itr.remove(ArrayList.java:980)
at delftstack.Illegal_State_Exception.main(Illegal_State_Exception.java:18)
To prevent the IllegalStateException
, call next()
before remove()
.
package delftstack;
import java.util.*;
public class Illegal_State_Exception {
public static void main(String args[]) {
List<String> Demo_List = new ArrayList<String>();
Demo_List.add("Delftstack1");
Demo_List.add("Delftstack2");
Demo_List.add("Delftstack3");
Demo_List.add("Delftstack4");
Demo_List.add("Delftstack5");
Iterator<String> Demo_Iter = Demo_List.iterator();
while (Demo_Iter.hasNext()) {
System.out.print(Demo_Iter.next() + "\n");
// Calling remove() after next() will work fine
Demo_Iter.remove();
}
}
}
The code above will work fine now.
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook