How to Iterate Through Set in Java
-
Iterate Over
Set/HashSet
in Java Using Enhancedfor
Loop -
Iterate a
Vector
Using Enumeration in Java -
Iterate Over
Set/HashSet
in Java by Converting It Into an Array -
Iterate Over
Set/HashSet
in Java by Using thestream()
Method of theArray
Class
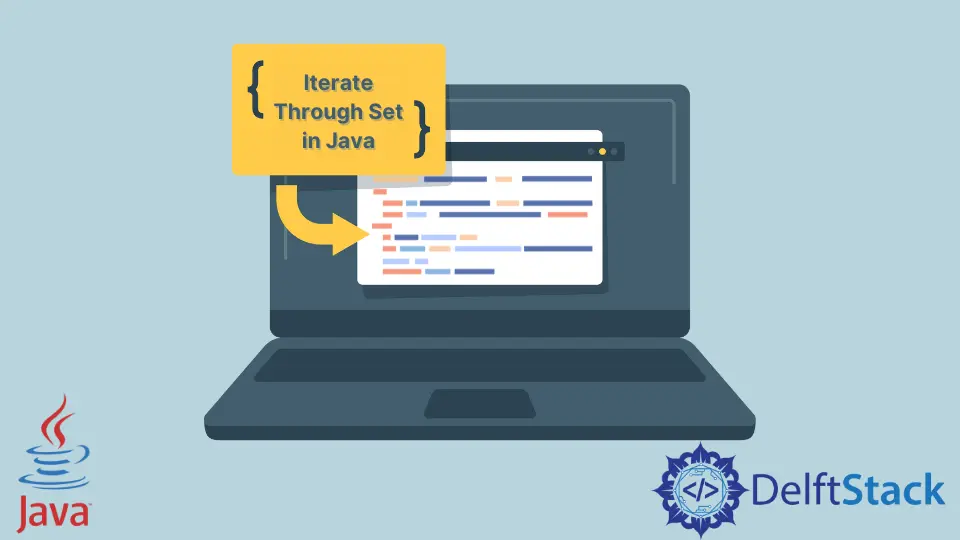
This article will introduce how to iterate through Set
or HashSet
in Java without using the Iterator. An iterator is used to return the elements in random order. This task can be accomplished using various methods other than an iterator.
Iterate Over Set/HashSet
in Java Using Enhanced for
Loop
for-each
loop in Java is also called an enhanced for
loop. It is used to iterate through elements of arrays and collections in Java.
Here we have created a Set
named mySet
using the HashSet
class, a collection of string elements. By calling the add()
method on mySet
we have added elements to mySet
.
Using the enhanced for
loop, we can iterate over each element inside the collection and print it out, as shown in the code. For each element s
of type String
in mySet
, we can iterate over it. Note that elements iterate in an unordered collection.
import java.util.HashSet;
import java.util.Set;
public class IterateOverHashSet {
public static void main(String[] args) {
Set<String> mySet = new HashSet<>();
mySet.add("Apple");
mySet.add("Banana");
mySet.add("Mango");
for (String s : mySet) {
System.out.println("s is : " + s);
}
}
}
Output:
s is : Mango
s is : Apple
s is : Banana
Iterate a Vector
Using Enumeration in Java
Vector
implements the List
interface, which helps us create resizeable arrays similar to the ArrayList
class. Firstly, we created a Set
of string type elements using the HashSet
class, later added elements to it using the add()
method.
myset
is then passed to the constructor of the Vector
class, and then the elements()
method is called on it to enumerate through the elements of Vector
.
We then use a while
loop to print till the Enumeration
(e
) has more elements. Consecutive calls to the method nextElements()
return successive elements of the series.
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Set;
import java.util.Vector;
public class IterateOverHashSet {
public static void main(String[] args) {
Set<String> mySet = new HashSet<>();
mySet.add("Apple");
mySet.add("Banana");
mySet.add("Mango");
Enumeration e = new Vector(mySet).elements();
while (e.hasMoreElements()) {
System.out.println(e.nextElement());
}
}
}
Output:
Mango
Apple
Banana
Iterate Over Set/HashSet
in Java by Converting It Into an Array
We first create a Set
of the String
type elements and add items to mySet
. To iterate over the elements, we convert our mySet
to an array using the toArray()
method. The toArray()
method returns an array that has the same elements as in HashSet
.
Then by using a for
loop, we can loop over all the elements in the new array named myArray
. Print each element, as shown in the code below.
import java.util.HashSet;
import java.util.Set;
public class IterateOverHashSet {
public static void main(String[] args) {
Set<String> mySet = new HashSet<>();
mySet.add("Apple");
mySet.add("Banana");
mySet.add("Mango");
String[] myArray = mySet.toArray(new String[mySet.size()]);
for (int index = 0; index < myArray.length; index++) {
System.out.println("element : " + myArray[index]);
}
}
}
Output:
element : Mango
element : Apple
element : Banana
Iterate Over Set/HashSet
in Java by Using the stream()
Method of the Array
Class
In the code shown below, we first create a Set
of String
type elements using the HashSet
class in Java. Then we add items to it by calling the add()
method on its instance fruits
.
The stream()
method returns a sequential stream of collection. We convert our Set
of String
- fruits
, into a stream
. Later we displayed the elements of the stream
using a lambda
expression.
import java.util.HashSet;
import java.util.Set;
import java.util.stream.Stream;
public class Simple {
public static void main(String args[]) {
Set<String> fruits = new HashSet<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Mango");
Stream<String> stream = fruits.stream();
stream.forEach((fruit) -> System.out.println(fruit));
}
}
Output:
Apple
Mango
Banana
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn