How to Iterate Through a Linked List in Java
-
Iterate Through the Linked-List Using the
for
Loop in Java -
Iterate Through the Linked List Using the Enhanced
for
Loop in Java -
Iterate Through the Linked List Using the
while
Loop -
Iterate the Linked List Using the
Iterator
Class in Java -
Iterate the Linked List Using Java 8
Streams
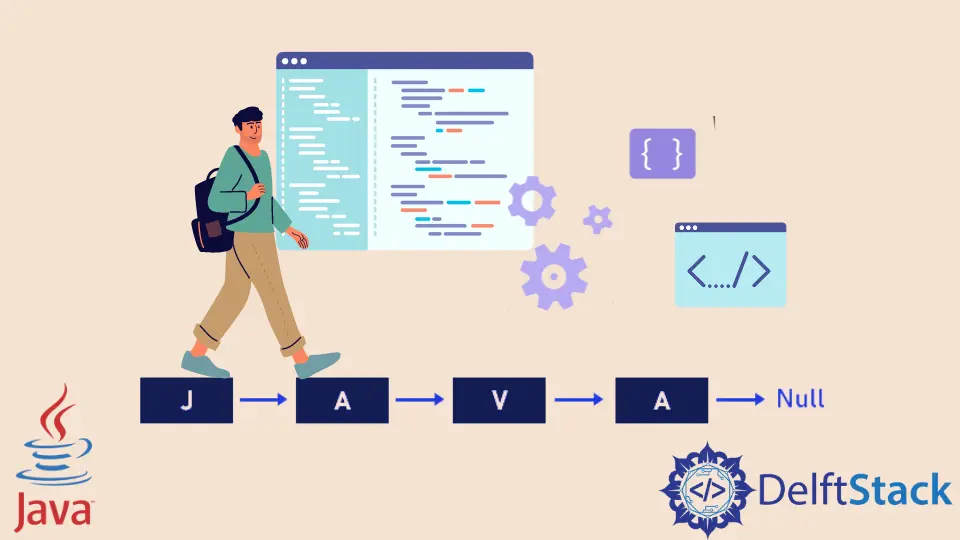
A linked list is a linear and ordered collection of data elements. The arrangement of elements is at ubiquitous or random locations in the memory. The linked list data structure is connected using nodes. The Node
holds the data field and reference link that are memory locations of the next element in the sequence.
We store the linked list elements in non-contiguous memory locations and, the last node contains a pointer to the null reference.
There are many ways to iterate over a linked list. Here are few ways mentioned below.
Iterate Through the Linked-List Using the for
Loop in Java
The LinkedList
class is instantiated using the new
keyword in the below code example. The add()
method of the LinkedList
class adds an element to the list. The add
method appends the specified element to the end of this list. This method is equivalent to the addLast
method. The method returns true
when the element gets successfully added to the list.
After the creation of the list, we use the for
loop for iteration over it. In the below code, int i=0
is an instantiation of the counter variable. Use a condition indicating the variable should be less than the size of the list. And the size of the list is calculated using the size()
method. At last, the value of the variable increments by one. This complete process will run until the variable value becomes more than the size of the list.
package linkedList;
import java.util.LinkedList;
public class IterateLinkedListUsingForLoop {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
System.out.println("Iterating the list using for-loop");
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
}
}
The above program generates the output as given below.
Iterating the list using for-loop
First
Second
Iterate Through the Linked List Using the Enhanced for
Loop in Java
The enhanced for
loop is also referred to as the for-each
loop and is one type of the for
loop.
In the below code, we should instantiate a linked list object. Then should add some elements (say String objects) to it. Now for iteration, we shall use for-each
in this case.
package linkedList;
import java.util.LinkedList;
public class IteratingLinkedListUsingForEach {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
for (String temp : list) {
System.out.println(temp);
}
}
}
The output of the program is the same as the one in the first program.
Iterate Through the Linked List Using the while
Loop
Instantiation and adding elements to the listing process will be similar in all the mentioned ways.
In the below example, the counter variable value gets instantiated to 0 outside the while
loop. The while
loop syntax has no place for instantiation. Now apply the condition. This checks if the variable is less than the size of the given list. It will return true, and the println()
function will get executed. After that, we should increment the counter variable. The process will repeat until the variable becomes more than the list size.
package linkedList;
public class IteratingLinkedListUsingWhileLoop {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
int i = 0;
while (i < list.size()) {
System.out.println(list.get(i));
i++;
}
}
}
Iterate the Linked List Using the Iterator
Class in Java
An Iterator
is a class that works on looping of Collections
objects. These are Array
, ArrayList
, LinkedList
and so on. We call it an Iterator
as it loops over the collection objects. One should use methods in the Iterator class to iterate over the Collections
objects.
We use the iterator()
method to get an Iterator
for any collection. The hasNext
method checks if the list has more- elements. It returns true
if the iteration has more elements present.
The next
method gets the next element in the loop. It returns the next present object in the iteration and throws NoSuchElementException
if no-element is present in the list.
The code below demonstrates the Iterator class and its methods.
package linkedList;
public class IteratingLinkedListUsingIterator {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
Iterate the Linked List Using Java 8 Streams
A stream
is always formed over a Collection
object. In the below program, we use the stream
method for the same. Functions get applied over the chain of stream functions. The forEach
is a terminal
operation. It means that one cannot perform any operation after a terminal function. It takes a consumer
stream, and this stream does not emits elements rather consumes them.
The below code shows the use of Java 8 streams and their function to iterate over the list.
package linkedList;
public class IteratingLinkedListUsingStreams {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
list.stream().forEach((element) -> { System.out.println(element); });
}
}
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn