String Interning in Java
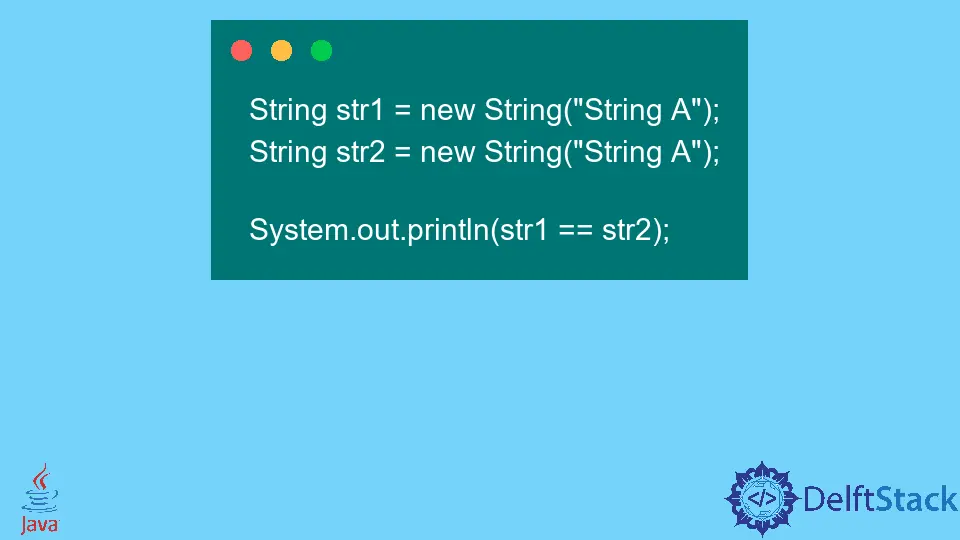
Today we will discuss String Interning or the intern()
method of the String class. In the below points, we will learn what String Interning is and why we need to use it, using an example.
What Is String Interning in Java and When to Use It
Java is an object-oriented programming language. When we create any object or variable, it takes some memory, which means that if we make a hundred instances of the String
class, they will be stored at different places in memory like a heap, which can consume a lot of space.
To tackle the issue, the Java Developers introduced the idea of a String Constant Pool (SCP) that contains objects that share the same memory pool.
The SCP contains all the String objects with the same content, making it an easy and memory-saving task to fetch the object as we do not have to find multiple objects. We only need a single object that can refer.
To understand it better, we can take a look at a simple example:
String str1 = new String("String A");
String str2 = new String("String A");
System.out.println(str1 == str2);
Output:
false
In the above piece of code, there are two objects of the String
class str1
and str2
, but both contain the same content. If we compare them using the ==
operator to check if they are equal, the output shows false
, making them unequal.
It happens because they are located at different locations in a heap. Besides being two objects, there are no significant differences in both objects.
If we use the concept of String Interning, we only create a single object let us assume it as strObj
, and now it contains the content of the objects. When we want to access the objects, we can refer to this object and get the content.
Using String Interning in Java
In the below example, we see how we can use the method of String Interning using the intern()
. We create five String variables.
The first String variable str
is created using new String()
, and the string is passed in the constructor. Next, we create another string object using the new
keyword, but we call the intern()
method from the String()
constructor.
The last three variables are string literals, in which the last variable, str5
, contains different content from all the other string variables. Notice that we have not used any intern()
method with the last three variables because the string literals are already placed in the SCP, which means that interning is applied to them automatically.
Now we compare the variables to check if they match. We use the ==
operator that returns true
if the object references the exact memory location. As we do not call the intern()
method with the first string str1
, it is in a different memory location, and thus str1 == str2
does not execute.
str2
is in the SCP because we call intern()
with it, and when we compare it with str3
, which is a string literal, it returns true
and executes the output. Next, we compare two string literals with the same content, str3
and str4
, that outputs the message in the console.
public class JavaExample {
public static void main(String[] args) {
String str1 = new String("String A");
String str2 = new String("String A").intern();
String str3 = "String A";
String str4 = "String A";
String str5 = "String B";
if (str1 == str2) {
System.out.println("str1 and str2 are in the same SCP");
}
if (str1 == str3) {
System.out.println("str1 and str3 are in the same SCP");
}
if (str2 == str3) {
System.out.println("str2 and str3 are in the same SCP");
}
if (str3 == str4) {
System.out.println("str3 and str4 are in the same SCP");
}
if (str4 == str5) {
System.out.println("str3 and str4 are in the same SCP");
}
}
}
Output:
str2 and str3 are in the same SCP
str3 and str4 are in the same SCP
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn