Infinity in Java
-
Introduction to
Infinity
in Java -
Methods in Implementing
Infinity
in Java -
Best Practices in Implementing
Infinity
in Java - Conclusion
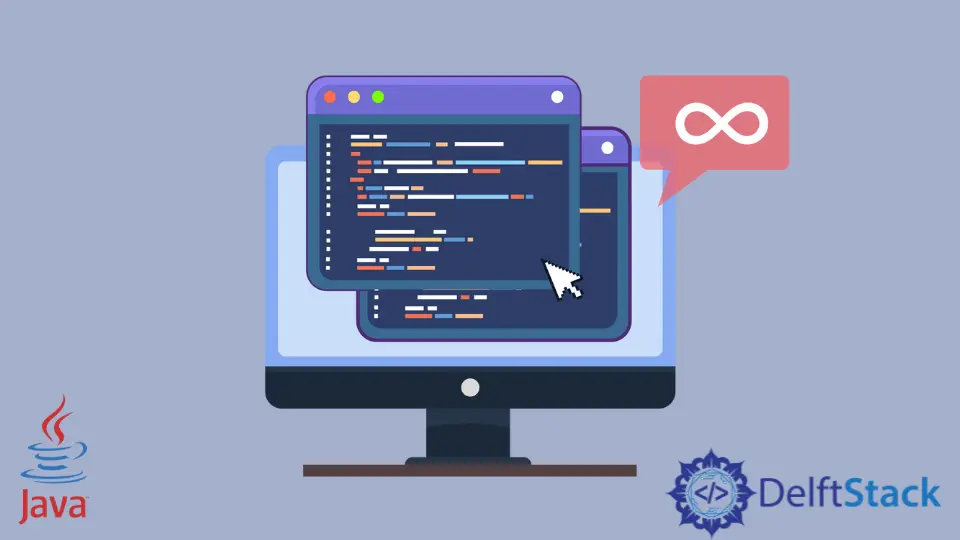
In this article, we explore the concept of infinity in Java, covering various methods for its implementation and best practices to ensure reliable and maintainable code.
Introduction to Infinity
in Java
In Java, infinity is a concept representing an unbounded numerical value. It’s used to denote values exceeding the representational limits, crucial in scenarios like mathematical computations and handling exceptional cases where limitless quantities are involved.
Methods in Implementing Infinity
in Java
Double and Float Constants
Representing infinity in Java using Double
and Float
constants is a straightforward yet powerful technique. These constants simplify the handling of unbounded values in mathematical computations and scenarios where infinity plays a crucial role.
By incorporating this method into your code, you enhance its readability and ensure a clear representation of infinity, contributing to the overall robustness of your Java applications.
Code Example:
public class InfinityExample {
public static void main(String[] args) {
// Using Double constants
double positiveInfinity = Double.POSITIVE_INFINITY;
double negativeInfinity = Double.NEGATIVE_INFINITY;
// Using Float constants
float positiveFloatInfinity = Float.POSITIVE_INFINITY;
float negativeFloatInfinity = Float.NEGATIVE_INFINITY;
// Displaying the results
System.out.println("Double Positive Infinity: " + positiveInfinity);
System.out.println("Double Negative Infinity: " + negativeInfinity);
System.out.println("Float Positive Infinity: " + positiveFloatInfinity);
System.out.println("Float Negative Infinity: " + negativeFloatInfinity);
}
}
The main
method acts as the entry point for the program.
Two double
variables, namely positiveInfinity
and negativeInfinity
, are declared and initialized using the POSITIVE_INFINITY
and NEGATIVE_INFINITY
constants provided by the Double
class. Similarly, two float
variables, positiveFloatInfinity
and negativeFloatInfinity
, are declared and initialized using the POSITIVE_INFINITY
and NEGATIVE_INFINITY
constants from the Float
class.
Finally, the program prints the values of positive and negative infinity for both double
and float
types.
When you run this Java program, the output will be as follows:
Double Positive Infinity: Infinity
Double Negative Infinity: -Infinity
Float Positive Infinity: Infinity
Float Negative Infinity: -Infinity
Division by Zero
The division by zero method offers a unique and practical way to implement infinity in Java. Although unconventional, this technique is a valuable tool when dealing with unbounded values, providing a clear representation of infinity in both double
and float
data types.
By leveraging this approach, Java programmers can enhance their ability to handle scenarios involving limitless numerical values with precision and simplicity.
Code Example:
public class DivisionByZeroExample {
public static void main(String[] args) {
// Using division by zero for double
double positiveInfinityDouble = 1.0 / 0.0;
double negativeInfinityDouble = -1.0 / 0.0;
// Using division by zero for float
float positiveInfinityFloat = 1.0f / 0.0f;
float negativeInfinityFloat = -1.0f / 0.0f;
// Displaying the results
System.out.println("Double Positive Infinity: " + positiveInfinityDouble);
System.out.println("Double Negative Infinity: " + negativeInfinityDouble);
System.out.println("Float Positive Infinity: " + positiveInfinityFloat);
System.out.println("Float Negative Infinity: " + negativeInfinityFloat);
}
}
In the provided Java code, the class DivisionByZeroExample
is introduced to illustrate how division by zero can result in the representation of infinity. The main
method acts as the starting point for the program’s execution.
Through the method of division by zero, double
variables—namely, positiveInfinityDouble
and negativeInfinityDouble
—are initialized to signify positive and negative infinity, respectively. Similarly, the same division by zero approach is employed for float
variables, positiveInfinityFloat
and negativeInfinityFloat
.
The concluding section of the code involves displaying the outcomes, where the program prints the values of positive and negative infinity for both double
and float
types.
Upon running the program, the output will be as follows:
Double Positive Infinity: Infinity
Double Negative Infinity: -Infinity
Float Positive Infinity: Infinity
Float Negative Infinity: -Infinity
This concise demonstration underscores how division by zero can be leveraged in Java to succinctly represent infinity, offering a straightforward approach for handling unbounded values in specific mathematical contexts.
Best Practices in Implementing Infinity
in Java
Choosing the Right Representation
When implementing infinity in Java, it’s crucial to select the appropriate representation based on the data type and requirements of your application. Understand the distinctions between double
and float
and choose the one that aligns with your precision needs.
Utilizing Constants for Clarity
Java provides constants such as Double.POSITIVE_INFINITY
and Float.POSITIVE_INFINITY
. Leveraging these constants enhances code clarity and readability, making it evident that the value represents positive infinity.
Handling Negative Infinity
Consider scenarios where negative infinity is relevant. Java accommodates this with constants like Double.NEGATIVE_INFINITY
and Float.NEGATIVE_INFINITY
. Ensure your implementation addresses both positive and negative infinity based on the context.
Math Class for Consistency
Using the Math
class constants (Math.POSITIVE_INFINITY
and Math.NEGATIVE_INFINITY
) is a consistent approach. This method ensures conformity in your code and may be preferable in situations where the Math
class is extensively used.
Division by Zero Caution
While the division by zero method is intriguing, exercise caution. It may lead to unexpected results or exceptions, and its usage is less conventional compared to constants or the isInfinite()
method.
Leveraging isInfinite()
for Detection
The isInfinite()
method is a valuable tool for detecting infinity in Java. Incorporate this method when you need to check whether a given value is infinite without relying on specific constants.
Ensuring Precision With isInfinite()
When dealing with floating-point numbers, be aware of precision issues. Utilize the isInfinite()
method judiciously, considering the implications of floating-point arithmetic and potential rounding errors.
Documentation for Clarity
Regardless of the chosen method, document your code. Clearly state the intention behind representing infinity, especially if unconventional methods like division by zero are employed. This documentation aids understanding for both yourself and other developers.
Unit Testing for Robustness
Implement unit tests to ensure the robustness of your infinity representation. Validate that your code handles positive and negative infinity appropriately and performs as expected in different scenarios.
Consideration for Specific Use Cases
Evaluate your application’s specific use cases. Depending on the domain and requirements, certain representations or methods may be more suitable. Tailor your approach to align with the broader context of your software.
Maintaining Consistency Across Codebase
If infinity representation is a recurring theme in your codebase, strive for consistency. Adopt a unified approach to ensure that different parts of your application handle infinity in a coherent manner. This consistency enhances maintainability and reduces the likelihood of errors.
Conclusion
Handling infinity in Java involves various methods, each suited to specific scenarios. Whether using constants or division by zero, it’s crucial to choose an approach aligned with best practices.
Best practices include avoiding division by zero, documenting the use of infinity, and testing edge cases to ensure robustness. By following these practices, developers can implement infinity in Java effectively, ensuring code readability, maintainability, and predictable behavior.