How to Handle EOFException in Java
- Java EOFException as a Subclass of Java IOException
- the Java EOFException
- Avoid the EOFException While Reading From the Stream in Java
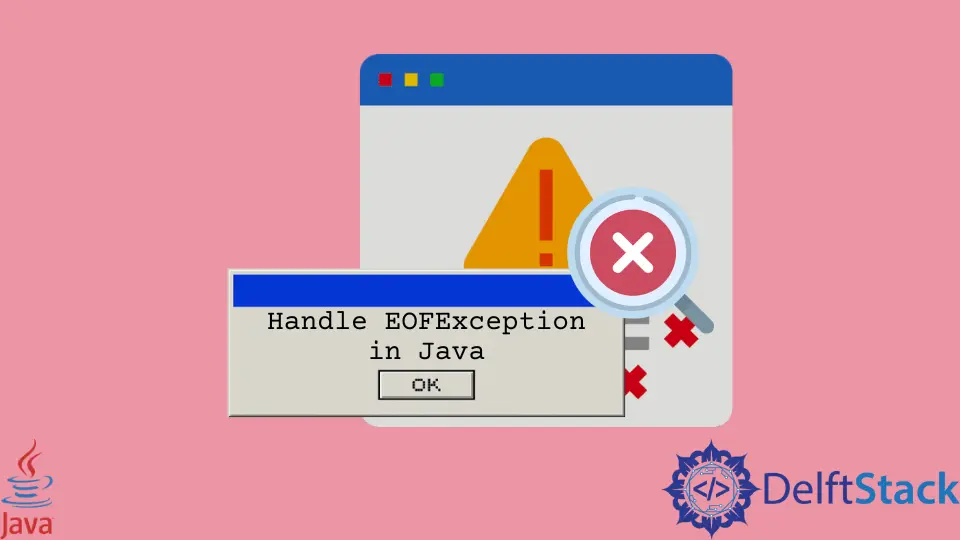
This article will discuss Java EOFException as a subclass of Java IOException and how to handle EOFException in Java.
Java EOFException as a Subclass of Java IOException
The Java EOFException is a subclass or a specific type of Java IOException, so we should know the IOException to understand EOFException.
Java throws the IOException to signal that some disruption due to input or output operation has occurred to the program during execution.
The IOException is a checked exception which means we must handle the exception correctly. We can handle exceptions using a try/catch
block or throwing the exception again.
If we do not handle the exceptions, the JVM will handle the exception and terminate the program’s execution.
Examples of Java IOException
We might be reading the data from a stream, and some other program closes the stream during the operation. In this case, Java will raise an IOException by throwing an IOException class object.
Other examples of raised IOException include network failure while reading from the server, inability to write to a file due to low disk space, and other file handling operations.
Example:
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class ExceptionExample {
public static void main(String[] args) {
File file = new File("MyFile.txt", "r");
try {
FileReader fr = new FileReader(file.getAbsoluteFile());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
java.io.FileNotFoundException: /home/stark/eclipse-workspace-java/JavaArticles/MyFile.txt/r (No such file or directory)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:219)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.io.FileReader.<init>(FileReader.java:75)
at ExceptionExample.main(ExceptionExample.java:12)
Note that the code uses the try/catch
block to handle the exception.
We must also note that the IOException object is not directly thrown in most cases. Instead, one of its subclass objects is thrown according to the exception that occurred.
the Java EOFException
A Java program raises an EOF (End Of File) Exception when the execution unexpectedly reaches the end of the file or end of the stream. This exception occurs when we read contents from files using stream objects.
When the end of the file is reached, it should be terminated. However, if the program still tries to read the file, Java raises the EOFException.
This type of exception is also a checked exception.
Example:
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStreamReader;
public class ExceptionExample {
public static void main(String[] args) {
File file = new File("/home/stark/eclipse-workspace-java/JavaArticles/src/MyFile.txt");
try {
DataInputStream dis = new DataInputStream(new FileInputStream(file));
int data;
while (true) {
data = dis.readInt();
System.out.println(data);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (EOFException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
1629512224
1663067168
1696622112
1730177056
1763732000
1797286944
1830842144
java.io.EOFException
at java.base/java.io.DataInputStream.readInt(DataInputStream.java:397)
at ExceptionExample.main(ExceptionExample.java:20)
In the example, code continuously reads a file using a stream in an infinite loop. Therefore, even after reaching the end of the file, code tries to read from the stream.
This is the reason we get the EOFException.
You should note that code handles the EOFException using the try/catch
block and prints the stack trace or the trace of executions that lead to the exception.
Avoid the EOFException While Reading From the Stream in Java
We can avoid the EOFException in the code if we correctly terminate the operation of reading the file as soon as the end of the file is reached.
Let us see the changes in the above example code to avoid the EOFException.
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStreamReader;
public class ExceptionExample {
public static void main(String[] args) {
File file = new File("/home/stark/eclipse-workspace-java/JavaArticles/src/MyFile.txt");
int data = 0;
try {
DataInputStream dis = new DataInputStream(new FileInputStream(file));
while (dis.available() > 4) {
data = dis.readInt();
System.out.println(data);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (EOFException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
1629512224
1663067168
1696622112
1730177056
1763732000
1797286944
1830842144
You can see that the code throws no exception. The code defines a condition that if there are less than 4 bytes in the stream, then the loop terminates.
This is because the size of the integer is 4 bytes in Java. You can define your conditions accordingly for terminating the read operations.
Alternatively, you can use a catch
block to terminate the reading operations after the exception is thrown, but it should not be preferred. However, you can use the catch
block to avoid unexpected inputs.