Java getContentPane()
-
Introduction to
getContentpane()
- Common Methods for Obtaining Content Pane
-
Best Practices for Implementing
getContentPane()
- Conclusion
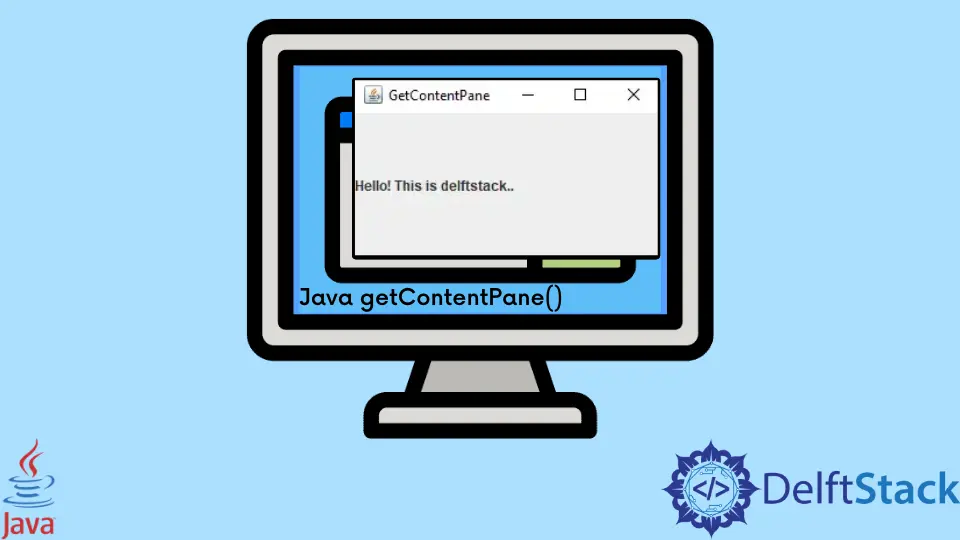
In Java Swing, a container has multiple layers in it, and the layer used to hold the objects is called the content pane. This content pane is implemented through the getContentPane()
method.
Navigating the intricacies of Swing development in Java involves understanding different methods for accessing the content pane, from direct usage of getContentPane()
to casting and utilizing getRootPane().getContentPane()
, all while adhering to best practices for optimal code clarity and reliability.
Introduction to getContentpane()
In Java, the getContentPane()
method doesn’t create a content pane; instead, it retrieves the existing content pane of a JFrame
. It’s crucial because it provides a direct way to add, remove, or customize Swing components within the main area of a graphical user interface.
By utilizing getContentPane()
, developers gain control over the visual presentation, enabling efficient manipulation of UI elements. This method simplifies the process of designing dynamic and interactive Java applications, making it a key practice for effective GUI development.
Common Methods for Obtaining Content Pane
Graphical User Interface (GUI) development in Java often involves the use of Swing, a powerful toolkit for creating interactive and visually appealing applications. One of the fundamental aspects of Swing programming is managing the content within a JFrame
.
The getContentPane()
method plays a pivotal role in this process, providing access to the content pane where UI components are added.
getContentPane()
Directly
Directly using getContentPane()
simplifies Swing development, providing a straightforward and concise way to access the content pane of a JFrame
. This method enhances code readability and reduces complexity, making it the preferred choice for adding components and customizing the user interface in a more intuitive manner.
Code Example:
import java.awt.*;
import javax.swing.*;
public class GetContentPaneExample extends JFrame {
public GetContentPaneExample(String title) {
super(title);
// Obtain the content pane directly
Container contentPane = getContentPane();
// Add a JButton to the content pane
contentPane.add(new JButton("Click Me"));
// Set layout manager (optional)
contentPane.setLayout(new FlowLayout());
// Set frame properties
setSize(300, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null); // Center the frame
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
// Create and display the frame
new GetContentPaneExample("Direct getContentPane() Example").setVisible(true);
});
}
}
In the GetContentPaneExample
class, which extends JFrame
for our graphical user interface, we start by initializing the frame with a specific title in the constructor. To manage the content within the frame, we utilize the getContentPane()
method.
Subsequently, a JButton
is added to the content pane, contributing to the user interface. While optional, we demonstrate the use of a layout manager, employing FlowLayout
to control component arrangement.
Additionally, various properties like size, default close operation, and location are set for the frame. The main
method ensures the application’s initiation on the Event Dispatch Thread (EDT) through SwingUtilities.invokeLater()
. This approach, centered around getContentPane()
, streamlines the process of constructing Swing applications with clarity and efficiency.
Output:
Upon running the program, a JFrame
window titled Direct getContentPane() Example
will appear. It contains a button with the label Click Me
positioned according to the specified layout manager.
This demonstrates how the direct use of getContentPane()
simplifies the process of adding components to the content pane, contributing to the efficiency of Swing-based GUI development.
Understanding and leveraging the getContentPane()
method enhances the clarity and conciseness of Swing code, making GUI development in Java an even more enjoyable experience.
Casting From getContentPane()
to Container
Casting from getContentPane()
to Container
offers precise control over the content pane, allowing specific manipulations. This method ensures type safety, enabling developers to tailor the container to their needs while maintaining clarity and avoiding ambiguity in Swing applications.
Code Example:
import java.awt.*;
import javax.swing.*;
public class CastedGetContentPaneExample extends JFrame {
public CastedGetContentPaneExample(String title) {
super(title);
// Cast getContentPane() result to Container
Container contentPane = (Container) getContentPane();
// Add a JButton to the content pane
contentPane.add(new JButton("Click Me"));
// Set layout manager (optional)
contentPane.setLayout(new FlowLayout());
// Set frame properties
setSize(300, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null); // Center the frame
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
// Create and display the frame
new CastedGetContentPaneExample("Casted getContentPane() Example").setVisible(true);
});
}
}
In the CastedGetContentPaneExample
class, an extension of JFrame
in our GUI, the constructor plays a pivotal role by leveraging casting to transform the result of getContentPane()
into a Container
. This casting ensures precision in manipulating the content pane.
A JButton
is then seamlessly added to the content pane, integrating it into the graphical interface. While optional, the use of a layout manager, such as FlowLayout
, is demonstrated for optimal component arrangement.
Additionally, various properties like size, default close operation, and location are set for the frame. The main
method ensures the application’s smooth initiation on the Event Dispatch Thread (EDT) through SwingUtilities.invokeLater()
.
This approach, centered around casting from getContentPane()
to Container
, provides developers with a clear and controlled means of customizing their Swing applications.
Output:
Upon executing the code, a JFrame
window titled Casted getContentPane() Example
will emerge. The window hosts a button labeled Click Me
, placed according to the specified layout manager.
This demonstration illustrates the power of casting in seamlessly integrating getContentPane()
with your application, allowing for enhanced control and customization in your Swing-based GUI development.
Casting the result of getContentPane()
to a Container
expands the developer’s toolkit, providing a versatile method for manipulating the content pane. This technique not only streamlines code but also offers greater flexibility in crafting sophisticated Swing applications.
getRootPane().getCOntentPane()
Using getRootPane().getContentPane()
provides a more comprehensive approach to accessing the content pane, allowing developers to navigate Swing’s hierarchy effectively. This method grants a deeper understanding of the component structure, facilitating precise customization and control over the graphical user interface in Java applications.
Code Example:
import java.awt.*;
import javax.swing.*;
public class HierarchyAccessExample extends JFrame {
public HierarchyAccessExample(String title) {
super(title);
// Access content pane via getRootPane()
Container contentPane = getRootPane().getContentPane();
// Add a JButton to the content pane
contentPane.add(new JButton("Click Me"));
// Set layout manager (optional)
contentPane.setLayout(new FlowLayout());
// Set frame properties
setSize(300, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null); // Center the frame
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
// Create and display the frame
new HierarchyAccessExample("Hierarchy Access Example").setVisible(true);
});
}
}
In the HierarchyAccessExample
class, extending JFrame
for our Swing application, the constructor takes a crucial step by utilizing getRootPane().getContentPane()
to access the content pane. This method provides a deeper understanding of Swing’s hierarchy, enabling precise customization and control over the graphical user interface.
A JButton
is seamlessly added to the content pane, becoming an integral part of the graphical interface. While optional, the use of a layout manager, exemplified here with FlowLayout
, aids in the optimal component arrangement.
Additional properties, including size and default close operation, are set for the frame. The main
method ensures the application’s initiation on the Event Dispatch Thread (EDT) through SwingUtilities.invokeLater()
.
This approach, centered around getRootPane().getContentPane()
, offers developers an insightful and powerful means of navigating and customizing the Swing component hierarchy.
Output:
Executing the code yields a JFrame
window titled Hierarchy Access Example
.
The window contains a button labeled Click Me
, positioned according to the specified layout manager. This showcases the effectiveness of using getRootPane().getContentPane()
as a method to navigate the Swing hierarchy and access the content pane seamlessly.
Grasping the intricacies of Swing’s component hierarchy can enhance your ability to manipulate and customize UI elements. The use of getRootPane().getContentPane()
serves as a bridge to understanding this hierarchy, providing developers with a unique perspective on content pane access in Swing-based applications.
Best Practices for Implementing getContentPane()
Practicing best practices for implementing getContentPane()
ensures code clarity, maintainability, and adherence to Swing’s threading rules. This approach promotes efficient GUI development, minimizing errors and enhancing the overall reliability of Java applications.
Purposeful Access
Access getContentPane()
only when necessary for direct manipulation or customization of the content pane. Avoid unnecessary calls to enhance code clarity.
Component Addition
Utilize getContentPane()
to add Swing components to the content pane. This method simplifies the process of building graphical user interfaces within a JFrame
.
Layout Management
When modifying the content pane, employ layout managers (e.g., FlowLayout
, BorderLayout
) to maintain a structured and organized arrangement of UI elements.
Customization
Leverage getContentPane()
for customizing the appearance of the content pane, such as setting background colors, borders, or other visual properties to enhance the overall user interface.
Component Removal
Use getContentPane()
to remove components from the content pane when necessary. This aids in dynamic user interface updates and efficient management of displayed elements.
Refrain From Redundancy
Avoid redundant calls to getContentPane()
within the same scope. Store the reference if multiple operations on the content pane are required to improve code efficiency.
Nested Content Panes
Consider using nested content panes for complex UI structures. This approach enables a hierarchical organization of components, enhancing code modularity and readability.
Responsive Repainting
Ensure to call repaint()
on the content pane after making modifications to guarantee that visual changes are reflected promptly in the user interface.
Consistent Style
Maintain a consistent coding style when utilizing getContentPane()
. Adhering to a standardized approach improves code readability and collaboration within development teams.
Conclusion
Understanding and applying various methods for obtaining the content pane, such as getContentPane()
directly, casting, or using getRootPane().getContentPane()
, are pivotal in Swing development.
However, practicing best practices, such as direct access and adherence to threading rules when implementing getContentPane()
, ensures code clarity, reliability, and efficient GUI development in Java applications.
Choose the method that aligns with the specific requirements of your application while embracing these best practices for a seamless and maintainable user interface.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook