How to Format Double Value in Java
-
Format
double
Value Using theDecimalFormat
Class in Java -
Format
double
Value Using theformat
Method in Java -
Format
double
Value Using theprintf
Method in Java -
Format
double
Value Usingformat
Method in Java -
Format
double
Value Using theString.format()
Method in Java -
Format
double
Value UsingDecimalFormat
Class in Java
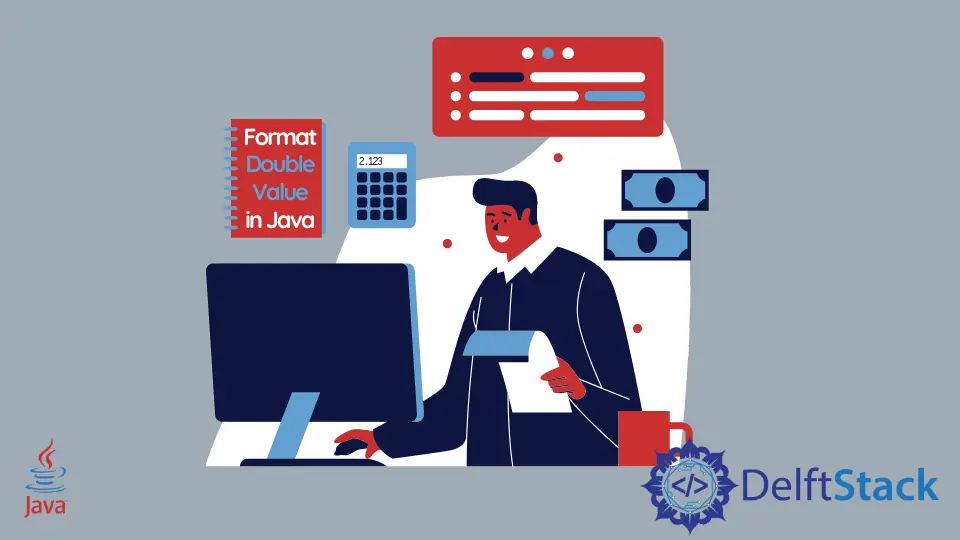
This tutorial introduces how to format double type value in Java.
There are several ways to format double value in Java such as the DecimalFormat
class, printf()
method, format()
method, String.format()
method, etc. Let’s take a close look at the examples.
Format double
Value Using the DecimalFormat
Class in Java
In this example, we use the DecimalFormat
class to format double type value in the specified format. For example, to format a double value to three decimal places, we use the format()
method and pass the DecimalFormat
constructor’s format style. See the example below.
import java.text.DecimalFormat;
import java.text.NumberFormat;
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 20.23;
System.out.println("Double Value: " + dVal);
String format = "0.000";
NumberFormat formatter = new DecimalFormat(format);
String newDVal = formatter.format(dVal);
System.out.println("Value After Formatting: " + newDVal);
}
}
Output:
String value: 123
Float value: 123.0
Format double
Value Using the format
Method in Java
This is one of the simplest examples, where we need to use the format()
method of System
class instead of print()
to format the double type value. This method acts as a printf()
method and prints the formatted output to the console. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 20.23;
System.out.println("Double Value: " + dVal);
System.out.format("Value after Formatting: %.3f", dVal);
}
}
Output:
Double Value: 20.23
Value after Formatting: 20.230
Format double
Value Using the printf
Method in Java
Java provides the printf()
method in the System class that can be used to print formatted output to the console. We use .2
for two decimal points and .3
for three decimal points. See the below example.
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 20.23;
System.out.println("Double Value: " + dVal);
System.out.printf("Value after Formatting: %.2f", dVal);
System.out.printf("\nValue after Formatting: %.3f", dVal);
}
}
Output:
Double Value: 20.23
Value after Formatting: 20.23
Value after Formatting: 20.230
Format double
Value Using format
Method in Java
The String
class contains a method format()
used to get a formatted String
in Java. We used these methods to format double type value in Java applications. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 20.23;
System.out.println("Double Value: " + dVal);
String val1 = String.format("%.2f", dVal);
String val2 = String.format("%.3f", dVal);
System.out.println("Value after Formatting: " + val1);
System.out.println("Value after Formatting: " + val2);
}
}
Output:
Double Value: 20.23
Value after Formatting: 20.23
Value after Formatting: 20.230
Format double
Value Using the String.format()
Method in Java
The String.format()
method allows one more feature to use a separator such as comma (,
) to format the double value in thousands, millions, etc. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 2000.23;
System.out.println("Double Value: " + dVal);
String val1 = String.format("$%,.2f", dVal);
String val2 = String.format("$%,.3f", dVal);
System.out.println("Value after Formatting: " + val1);
System.out.println("Value after Formatting: " + val2);
}
}
Output:
Double Value: 2000.23
Value after Formatting: $2,000.23
Value after Formatting: $2,000.230
Format double
Value Using DecimalFormat
Class in Java
We use the DecimalFormat
class and getCurrencyInstance()
method of the NumberFormat
class to create double type value to a currency instance. We use the setMinimumFractionDigits()
method to specify the number of digits after the decimal point in the double type value. See the example below.
import java.text.DecimalFormat;
import java.text.NumberFormat;
public class SimpleTesting {
public static void main(String[] args) {
double dVal = 2000.23;
System.out.println("Double Value: " + dVal);
DecimalFormat decimalFormat = (DecimalFormat) NumberFormat.getCurrencyInstance();
decimalFormat.setMinimumFractionDigits(2);
String val1 = decimalFormat.format(dVal);
System.out.println("Value after Formatting: " + val1);
decimalFormat.setMinimumFractionDigits(3);
String val2 = decimalFormat.format(dVal);
System.out.println("Value after Formatting: " + val2);
}
}
Output:
Double Value: 2000.23
Value after Formatting: $2,000.23
Value after Formatting: $2,000.230